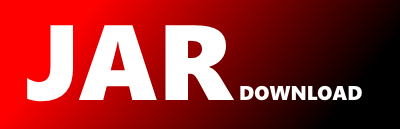
io.vertx.rxjava3.ext.web.client.HttpRequest Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.web.client;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A client-side HTTP request.
*
* Instances are created by an {@link io.vertx.rxjava3.ext.web.client.WebClient} instance, via one of the methods corresponding to the specific
* HTTP methods such as {@link io.vertx.rxjava3.ext.web.client.WebClient#get}, etc...
*
* The request shall be configured prior sending, the request is immutable and when a mutator method
* is called, a new request is returned allowing to expose the request in a public API and apply further customization.
*
* After the request has been configured, the methods
*
* - {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send}
* - {@link io.vertx.rxjava3.ext.web.client.HttpRequest#sendStream}
* - {@link io.vertx.rxjava3.ext.web.client.HttpRequest#sendJson} ()}
* - {@link io.vertx.rxjava3.ext.web.client.HttpRequest#sendForm}
*
* can be called.
* The sendXXX
methods perform the actual request, they can be called multiple times to perform the same HTTP
* request at different points in time.
*
* The handler is called back with
*
* - an {@link io.vertx.rxjava3.ext.web.client.HttpResponse} instance when the HTTP response has been received
* - a failure when the HTTP request failed (like a connection error) or when the HTTP response could
* not be obtained (like connection or unmarshalling errors)
*
*
* Most of the time, this client will buffer the HTTP response fully unless a specific is used
* such as .
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.client.HttpRequest original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.web.client.HttpRequest.class)
public class HttpRequest {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpRequest that = (HttpRequest) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpRequest((io.vertx.ext.web.client.HttpRequest) obj),
HttpRequest::getDelegate
);
private final io.vertx.ext.web.client.HttpRequest delegate;
public final TypeArg __typeArg_0;
public HttpRequest(io.vertx.ext.web.client.HttpRequest delegate) {
this.delegate = delegate;
this.__typeArg_0 = TypeArg.unknown(); }
public HttpRequest(Object delegate, TypeArg typeArg_0) {
this.delegate = (io.vertx.ext.web.client.HttpRequest)delegate;
this.__typeArg_0 = typeArg_0;
}
public io.vertx.ext.web.client.HttpRequest getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.ext.web.client.predicate.ResponsePredicate.newInstance((io.vertx.ext.web.client.predicate.ResponsePredicate)o1), o1 -> o1.getDelegate());
/**
* Configure the request to use a new method value
.
* @param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest method(io.vertx.core.http.HttpMethod value) {
delegate.method(value);
return this;
}
/**
* @return the request method
*/
public io.vertx.core.http.HttpMethod method() {
io.vertx.core.http.HttpMethod ret = delegate.method();
return ret;
}
/**
* Configure the request to use a new port value
.
* This overrides the port set by absolute URI requests
* @param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest port(int value) {
delegate.port(value);
return this;
}
/**
* @return the request port or 0
when none is set for absolute URI templates
*/
public int port() {
int ret = delegate.port();
return ret;
}
/**
* Configure the request to decode the response with the responseCodec
.
* @param responseCodec the response codec
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest as(io.vertx.rxjava3.ext.web.codec.BodyCodec responseCodec) {
io.vertx.rxjava3.ext.web.client.HttpRequest ret = io.vertx.rxjava3.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.as(responseCodec.getDelegate()), responseCodec.__typeArg_0);
return ret;
}
/**
* @return the request body codec
*/
public io.vertx.rxjava3.ext.web.codec.BodyCodec bodyCodec() {
io.vertx.rxjava3.ext.web.codec.BodyCodec ret = io.vertx.rxjava3.ext.web.codec.BodyCodec.newInstance((io.vertx.ext.web.codec.BodyCodec)delegate.bodyCodec(), __typeArg_0);
return ret;
}
/**
* Configure the request to use a new host value
.
* This overrides the host set by absolute URI requests
* @param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest host(java.lang.String value) {
delegate.host(value);
return this;
}
/**
* @return the request host or null
when none is set for absolute URI templates
*/
public java.lang.String host() {
java.lang.String ret = delegate.host();
return ret;
}
/**
* Configure the request to use a virtual host value
.
*
* Usually the header host (:authority pseudo header for HTTP/2) is set from the request host value
* since this host value resolves to the server IP address.
*
* Sometimes you need to set a host header for an address that does not resolve to the server IP address.
* The virtual host value overrides the value of the actual host header (:authority pseudo header
* for HTTP/2).
*
* The virtual host is also be used for SNI.
* @param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest virtualHost(java.lang.String value) {
delegate.virtualHost(value);
return this;
}
/**
* @return the request virtual host if any or null
*/
public java.lang.String virtualHost() {
java.lang.String ret = delegate.virtualHost();
return ret;
}
/**
* Configure the request to use a new request URI value
.
* This overrides the port set by absolute URI requests
*
When the uri has query parameters, they are set in the {@link io.vertx.rxjava3.ext.web.client.HttpRequest#queryParams}, overwriting
* any parameters previously set
* @param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest uri(java.lang.String value) {
delegate.uri(value);
return this;
}
/**
* @return the request uri or null
when none is set for absolute URI templates
*/
public java.lang.String uri() {
java.lang.String ret = delegate.uri();
return ret;
}
/**
* Configure the request to add multiple HTTP headers .
* @param headers The HTTP headers
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest putHeaders(io.vertx.rxjava3.core.MultiMap headers) {
delegate.putHeaders(headers.getDelegate());
return this;
}
/**
* Configure the request to set a new HTTP header.
* @param name the header name
* @param value the header value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest putHeader(java.lang.String name, java.lang.String value) {
delegate.putHeader(name, value);
return this;
}
/**
* @return The HTTP headers
*/
public io.vertx.rxjava3.core.MultiMap headers() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.headers());
cached_0 = ret;
return ret;
}
/**
* Configure the request to perform basic access authentication.
*
* In basic HTTP authentication, a request contains a header field of the form 'Authorization: Basic <credentials>',
* where credentials is the base64 encoding of id and password joined by a colon.
*
* In practical terms the arguments are converted to a object.
* @param id the id
* @param password the password
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest basicAuthentication(java.lang.String id, java.lang.String password) {
delegate.basicAuthentication(id, password);
return this;
}
/**
* Configure the request to perform basic access authentication.
*
* In basic HTTP authentication, a request contains a header field of the form 'Authorization: Basic <credentials>',
* where credentials is the base64 encoding of id and password joined by a colon.
*
* In practical terms the arguments are converted to a object.
* @param id the id
* @param password the password
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest basicAuthentication(io.vertx.rxjava3.core.buffer.Buffer id, io.vertx.rxjava3.core.buffer.Buffer password) {
delegate.basicAuthentication(id.getDelegate(), password.getDelegate());
return this;
}
/**
* Configure the request to perform bearer token authentication.
*
* In OAuth 2.0, a request contains a header field of the form 'Authorization: Bearer <bearerToken>',
* where bearerToken is the bearer token issued by an authorization server to access protected resources.
*
* In practical terms the arguments are converted to a object.
* @param bearerToken the bearer token
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest bearerTokenAuthentication(java.lang.String bearerToken) {
delegate.bearerTokenAuthentication(bearerToken);
return this;
}
/**
* Configure the request whether to use SSL.
* This overrides the SSL value set by absolute URI requests
* @param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest ssl(java.lang.Boolean value) {
delegate.ssl(value);
return this;
}
/**
* @return whether the request uses SSL or null
when none is set for absolute URI templates
*/
public java.lang.Boolean ssl() {
java.lang.Boolean ret = delegate.ssl();
return ret;
}
/**
* Sets both connect and idle timeouts for the request
*
*
* - connect timeout: if the request is not obtained from the client within the timeout period, the
Future
* obtained from the client is failed with a {@link java.util.concurrent.TimeoutException}.
* - idle timeout: if the request does not return any data within the timeout period, the request/response is closed and the
* related futures are failed with a {@link java.util.concurrent.TimeoutException}, e.g.
Future
* or Future
response body.
*
*
* The connect and idle timeouts can be set separately using {@link io.vertx.rxjava3.ext.web.client.HttpRequest#connectTimeout} and {@link io.vertx.rxjava3.ext.web.client.HttpRequest#idleTimeout}
* @param value
* @return
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest timeout(long value) {
delegate.timeout(value);
return this;
}
/**
* @return the current timeout in milliseconds
*/
public long timeout() {
long ret = delegate.timeout();
return ret;
}
/**
* Sets the amount of time after which, if the request does not return any data within the timeout period,
* the request/response is closed and the related futures are failed with a {@link java.util.concurrent.TimeoutException}.
*
* The timeout starts after a connection is obtained from the client.
* @param timeout the amount of time in milliseconds.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest idleTimeout(long timeout) {
delegate.idleTimeout(timeout);
return this;
}
/**
* @return the idle timeout in milliseconds
*/
public long idleTimeout() {
long ret = delegate.idleTimeout();
return ret;
}
/**
* Sets the amount of time after which, if the request is not obtained from the client within the timeout period,
* the response is failed with a {@link java.util.concurrent.TimeoutException}.
*
* Note this is not related to the TCP option, when a request is made against
* a pooled HTTP client, the timeout applies to the duration to obtain a connection from the pool to serve the request, the timeout
* might fire because the server does not respond in time or the pool is too busy to serve a request.
* @param timeout the amount of time in milliseconds.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest connectTimeout(long timeout) {
delegate.connectTimeout(timeout);
return this;
}
/**
* @return the connect timeout in milliseconds
*/
public long connectTimeout() {
long ret = delegate.connectTimeout();
return ret;
}
/**
* Add a query parameter to the request.
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest addQueryParam(java.lang.String paramName, java.lang.String paramValue) {
delegate.addQueryParam(paramName, paramValue);
return this;
}
/**
* Set a query parameter to the request.
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest setQueryParam(java.lang.String paramName, java.lang.String paramValue) {
delegate.setQueryParam(paramName, paramValue);
return this;
}
/**
* Set a request URI template string parameter to the request, expanded when the request URI is a .
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest setTemplateParam(java.lang.String paramName, java.lang.String paramValue) {
delegate.setTemplateParam(paramName, paramValue);
return this;
}
/**
* Set a request URI template list parameter to the request, expanded when the request URI is a .
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest setTemplateParam(java.lang.String paramName, java.util.List paramValue) {
delegate.setTemplateParam(paramName, paramValue);
return this;
}
/**
* Set a request URI template map parameter to the request, expanded when the request URI is a .
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest setTemplateParam(java.lang.String paramName, java.util.Map paramValue) {
delegate.setTemplateParam(paramName, paramValue);
return this;
}
/**
* Set whether to follow request redirections
* @param value true if redirections should be followed
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest followRedirects(boolean value) {
delegate.followRedirects(value);
return this;
}
/**
* @return whether to follow request redirections
*/
public boolean followRedirects() {
boolean ret = delegate.followRedirects();
return ret;
}
/**
* Configure the request to set a proxy for this request.
*
* Setting proxy here supersedes the proxy set on the client itself
* @param proxyOptions The proxy options
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest proxy(io.vertx.core.net.ProxyOptions proxyOptions) {
delegate.proxy(proxyOptions);
return this;
}
/**
* @return the proxy for this request
*/
public io.vertx.core.net.ProxyOptions proxy() {
io.vertx.core.net.ProxyOptions ret = delegate.proxy();
return ret;
}
/**
* Add an expectation that the response is valid according to the provided predicate
.
*
* Multiple predicates can be added.
* @param predicate the predicate
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.ext.web.client.HttpRequest expect(java.util.function.Function,io.vertx.rxjava3.ext.web.client.predicate.ResponsePredicateResult> predicate) {
delegate.expect(new Function,io.vertx.ext.web.client.predicate.ResponsePredicateResult>() {
public io.vertx.ext.web.client.predicate.ResponsePredicateResult apply(io.vertx.ext.web.client.HttpResponse arg) {
io.vertx.rxjava3.ext.web.client.predicate.ResponsePredicateResult ret = predicate.apply(io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)arg, TypeArg.unknown()));
return ret.getDelegate();
}
});
return this;
}
/**
* Add an expectation that the response is valid according to the provided predicate
.
*
* Multiple predicates can be added.
* @param predicate the predicate
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.ext.web.client.HttpRequest expect(io.vertx.rxjava3.ext.web.client.predicate.ResponsePredicate predicate) {
delegate.expect(predicate.getDelegate());
return this;
}
/**
* @return a read-only list of the response predicate expectations
*/
@Deprecated()
public java.util.List expectations() {
java.util.List ret = delegate.expectations().stream().map(elt -> io.vertx.rxjava3.ext.web.client.predicate.ResponsePredicate.newInstance((io.vertx.ext.web.client.predicate.ResponsePredicate)elt)).collect(Collectors.toList());
return ret;
}
/**
* Return the current query parameters.
* @return the current query parameters
*/
public io.vertx.rxjava3.core.MultiMap queryParams() {
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.queryParams());
return ret;
}
/**
* Return the current request URI template parameters.
* @return the current request URI template parameters
*/
public io.vertx.rxjava3.uritemplate.Variables templateParams() {
io.vertx.rxjava3.uritemplate.Variables ret = io.vertx.rxjava3.uritemplate.Variables.newInstance((io.vertx.uritemplate.Variables)delegate.templateParams());
return ret;
}
/**
* Copy this request
* @return a copy of this request
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest copy() {
io.vertx.rxjava3.ext.web.client.HttpRequest ret = io.vertx.rxjava3.ext.web.client.HttpRequest.newInstance((io.vertx.ext.web.client.HttpRequest)delegate.copy(), __typeArg_0);
return ret;
}
/**
* Allow or disallow multipart mixed encoding when sending having files sharing the same
* file name.
*
* The default value is true
.
*
* Set to false
if you want to achieve the behavior for HTML5.
* @param allow true
allows use of multipart mixed encoding
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest multipartMixed(boolean allow) {
delegate.multipartMixed(allow);
return this;
}
/**
* @return whether multipart mixed encoding is allowed
*/
public boolean multipartMixed() {
boolean ret = delegate.multipartMixed();
return ret;
}
/**
* Trace operation name override.
* @param traceOperation Name of operation to use in traces
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest traceOperation(java.lang.String traceOperation) {
delegate.traceOperation(traceOperation);
return this;
}
/**
* @return the trace operation name override
*/
public java.lang.String traceOperation() {
java.lang.String ret = delegate.traceOperation();
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
stream.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> sendStream(io.reactivex.rxjava3.core.Flowable body) {
io.reactivex.rxjava3.core.Single> ret = rxSendStream(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
stream.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendStream(io.reactivex.rxjava3.core.Flowable body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendStream(io.vertx.rxjava3.impl.ReadStreamSubscriber.asReadStream(body, obj -> obj.getDelegate()).resume(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
buffer.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> sendBuffer(io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Single> ret = rxSendBuffer(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
buffer.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendBuffer(io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendBuffer(body.getDelegate(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> sendJsonObject(io.vertx.core.json.JsonObject body) {
io.reactivex.rxjava3.core.Single> ret = rxSendJsonObject(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendJsonObject(io.vertx.core.json.JsonObject body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendJsonObject(body, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> sendJson(java.lang.Object body) {
io.reactivex.rxjava3.core.Single> ret = rxSendJson(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendJson(java.lang.Object body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendJson(body, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to application/x-www-form-urlencoded
.
*
* When the content type header is previously set to multipart/form-data
it will be used instead.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> sendForm(io.vertx.rxjava3.core.MultiMap body) {
io.reactivex.rxjava3.core.Single> ret = rxSendForm(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to application/x-www-form-urlencoded
.
*
* When the content type header is previously set to multipart/form-data
it will be used instead.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendForm(io.vertx.rxjava3.core.MultiMap body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendForm(body.getDelegate(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to application/x-www-form-urlencoded
.
*
* When the content type header is previously set to multipart/form-data
it will be used instead.
*
* NOTE: the use of this method is strongly discouraged to use when the form is a application/x-www-form-urlencoded
* encoded form since the charset to use must be UTF-8.
* @param body the body
* @param charset
* @return
*/
public io.reactivex.rxjava3.core.Single> sendForm(io.vertx.rxjava3.core.MultiMap body, java.lang.String charset) {
io.reactivex.rxjava3.core.Single> ret = rxSendForm(body, charset);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to application/x-www-form-urlencoded
.
*
* When the content type header is previously set to multipart/form-data
it will be used instead.
*
* NOTE: the use of this method is strongly discouraged to use when the form is a application/x-www-form-urlencoded
* encoded form since the charset to use must be UTF-8.
* @param body the body
* @param charset
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendForm(io.vertx.rxjava3.core.MultiMap body, java.lang.String charset) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendForm(body.getDelegate(), charset, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to multipart/form-data
. You may use this method to send attributes and upload files.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> sendMultipartForm(io.vertx.rxjava3.ext.web.multipart.MultipartForm body) {
io.reactivex.rxjava3.core.Single> ret = rxSendMultipartForm(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to multipart/form-data
. You may use this method to send attributes and upload files.
* @param body the body
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSendMultipartForm(io.vertx.rxjava3.ext.web.multipart.MultipartForm body) {
return AsyncResultSingle.toSingle( handler -> {
delegate.sendMultipartForm(body.getDelegate(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Send a request, the handler
will receive the response as an {@link io.vertx.rxjava3.ext.web.client.HttpResponse}.
* @return
*/
public io.reactivex.rxjava3.core.Single> send() {
io.reactivex.rxjava3.core.Single> ret = rxSend();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Send a request, the handler
will receive the response as an {@link io.vertx.rxjava3.ext.web.client.HttpResponse}.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxSend() {
return AsyncResultSingle.toSingle( handler -> {
delegate.send(new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.client.HttpResponse.newInstance((io.vertx.ext.web.client.HttpResponse)event, __typeArg_0))));
});
}
/**
* Configure the request to set a new HTTP header with multiple values.
* @param name the header name
* @param value the header value
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest putHeader(java.lang.String name, java.lang.Iterable value) {
delegate.putHeader(name, value);
return this;
}
/**
* Configure the request to perform HTTP Authentication.
*
* Performs a generic authentication using the credentials provided by the user. For the sake of validation safety
* it is recommended that is called to ensure that the credentials
* are applicable to the HTTP Challenged received on a previous request that returned a 401 response code.
* @param credentials the credentials to use.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.client.HttpRequest authentication(io.vertx.ext.auth.authentication.Credentials credentials) {
delegate.authentication(credentials);
return this;
}
private io.vertx.rxjava3.core.MultiMap cached_0;
public static HttpRequest newInstance(io.vertx.ext.web.client.HttpRequest arg) {
return arg != null ? new HttpRequest(arg) : null;
}
public static HttpRequest newInstance(io.vertx.ext.web.client.HttpRequest arg, TypeArg __typeArg_T) {
return arg != null ? new HttpRequest(arg, __typeArg_T) : null;
}
}