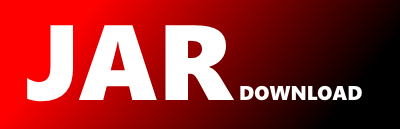
io.vertx.rxjava3.ext.web.client.HttpResponse Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.web.client;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* An HTTP response.
*
* The usual HTTP response attributes are available:
*
* - {@link io.vertx.rxjava3.core.http.HttpResponseHead#statusCode} the HTTP status code
* - {@link io.vertx.rxjava3.core.http.HttpResponseHead#statusMessage} the HTTP status message
* - {@link io.vertx.rxjava3.core.http.HttpResponseHead#headers} the HTTP headers
* - {@link io.vertx.rxjava3.core.http.HttpResponseHead#version} the HTTP version
*
*
* The body of the response is returned by {@link io.vertx.rxjava3.ext.web.client.HttpResponse#body} decoded as the format specified by the {@link io.vertx.rxjava3.ext.web.codec.BodyCodec} that
* built the response.
*
* Keep in mind that using this HttpResponse
impose to fully buffer the response body and should be used for payload
* that can fit in memory.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.client.HttpResponse original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.web.client.HttpResponse.class)
public class HttpResponse implements io.vertx.rxjava3.core.http.HttpResponseHead {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
HttpResponse that = (HttpResponse) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new HttpResponse((io.vertx.ext.web.client.HttpResponse) obj),
HttpResponse::getDelegate
);
private final io.vertx.ext.web.client.HttpResponse delegate;
public final TypeArg __typeArg_0;
public HttpResponse(io.vertx.ext.web.client.HttpResponse delegate) {
this.delegate = delegate;
this.__typeArg_0 = TypeArg.unknown(); }
public HttpResponse(Object delegate, TypeArg typeArg_0) {
this.delegate = (io.vertx.ext.web.client.HttpResponse)delegate;
this.__typeArg_0 = typeArg_0;
}
public io.vertx.ext.web.client.HttpResponse getDelegate() {
return delegate;
}
/**
* @return the version of the response
*/
public io.vertx.core.http.HttpVersion version() {
io.vertx.core.http.HttpVersion ret = delegate.version();
return ret;
}
/**
* @return the status code of the response
*/
public int statusCode() {
int ret = delegate.statusCode();
return ret;
}
/**
* @return the status message of the response
*/
public java.lang.String statusMessage() {
java.lang.String ret = delegate.statusMessage();
return ret;
}
/**
* @return the headers
*/
public io.vertx.rxjava3.core.MultiMap headers() {
if (cached_0 != null) {
return cached_0;
}
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.headers());
cached_0 = ret;
return ret;
}
/**
* Return the first header value with the specified name
* @param headerName the header name
* @return the header value
*/
public java.lang.String getHeader(java.lang.String headerName) {
java.lang.String ret = delegate.getHeader(headerName);
return ret;
}
/**
* @return the Set-Cookie headers (including trailers)
*/
public java.util.List cookies() {
if (cached_1 != null) {
return cached_1;
}
java.util.List ret = delegate.cookies();
cached_1 = ret;
return ret;
}
/**
* @return the trailers
*/
public io.vertx.rxjava3.core.MultiMap trailers() {
if (cached_2 != null) {
return cached_2;
}
io.vertx.rxjava3.core.MultiMap ret = io.vertx.rxjava3.core.MultiMap.newInstance((io.vertx.core.MultiMap)delegate.trailers());
cached_2 = ret;
return ret;
}
/**
* Return the first trailer value with the specified name
* @param trailerName the trailer name
* @return the trailer value
*/
public java.lang.String getTrailer(java.lang.String trailerName) {
java.lang.String ret = delegate.getTrailer(trailerName);
return ret;
}
/**
* @return the response body in the format it was decoded.
*/
public T body() {
if (cached_3 != null) {
return cached_3;
}
T ret = (T)__typeArg_0.wrap(delegate.body());
cached_3 = ret;
return ret;
}
/**
* @return the response body decoded as a , or null
if a codec other than was used
*/
public io.vertx.rxjava3.core.buffer.Buffer bodyAsBuffer() {
if (cached_4 != null) {
return cached_4;
}
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.bodyAsBuffer());
cached_4 = ret;
return ret;
}
/**
* @return the list of all followed redirects, including the final location.
*/
public java.util.List followedRedirects() {
if (cached_5 != null) {
return cached_5;
}
java.util.List ret = delegate.followedRedirects();
cached_5 = ret;
return ret;
}
/**
* @return the response body decoded as a String
, or null
if a codec other than was used
*/
public java.lang.String bodyAsString() {
if (cached_6 != null) {
return cached_6;
}
java.lang.String ret = delegate.bodyAsString();
cached_6 = ret;
return ret;
}
/**
* @param encoding
* @return the response body decoded as a String
given a specific encoding
, or null
if a codec other than was used
*/
public java.lang.String bodyAsString(java.lang.String encoding) {
java.lang.String ret = delegate.bodyAsString(encoding);
return ret;
}
/**
* @return the response body decoded as , or null
if a codec other than was used
*/
public io.vertx.core.json.JsonObject bodyAsJsonObject() {
if (cached_7 != null) {
return cached_7;
}
io.vertx.core.json.JsonObject ret = delegate.bodyAsJsonObject();
cached_7 = ret;
return ret;
}
/**
* @return the response body decoded as a , or null
if a codec other than was used
*/
public io.vertx.core.json.JsonArray bodyAsJsonArray() {
if (cached_8 != null) {
return cached_8;
}
io.vertx.core.json.JsonArray ret = delegate.bodyAsJsonArray();
cached_8 = ret;
return ret;
}
/**
* @param type
* @return the response body decoded as the specified type
with the Jackson mapper, or null
if a codec other than was used
*/
public R bodyAsJson(java.lang.Class type) {
R ret = (R)TypeArg.of(type).wrap(delegate.bodyAsJson(io.vertx.lang.rxjava3.Helper.unwrap(type)));
return ret;
}
/**
* Return the first header value with the specified name
* @param headerName the header name
* @return the header value
*/
public java.lang.String getHeader(java.lang.CharSequence headerName) {
java.lang.String ret = delegate.getHeader(headerName);
return ret;
}
private io.vertx.rxjava3.core.MultiMap cached_0;
private java.util.List cached_1;
private io.vertx.rxjava3.core.MultiMap cached_2;
private T cached_3;
private io.vertx.rxjava3.core.buffer.Buffer cached_4;
private java.util.List cached_5;
private java.lang.String cached_6;
private io.vertx.core.json.JsonObject cached_7;
private io.vertx.core.json.JsonArray cached_8;
public static HttpResponse newInstance(io.vertx.ext.web.client.HttpResponse arg) {
return arg != null ? new HttpResponse(arg) : null;
}
public static HttpResponse newInstance(io.vertx.ext.web.client.HttpResponse arg, TypeArg __typeArg_T) {
return arg != null ? new HttpResponse(arg, __typeArg_T) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy