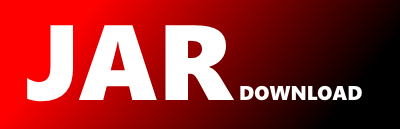
io.vertx.rxjava3.ext.web.handler.SessionHandler Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.web.handler;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A handler that maintains a {@link io.vertx.rxjava3.ext.web.Session} for each browser
* session.
*
* It looks up the session for each request based on a session cookie which
* contains a session ID. It stores the session when the response is ended in
* the session store.
*
* The session is available on the routing context with
* .
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.handler.SessionHandler original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.web.handler.SessionHandler.class)
public class SessionHandler implements io.vertx.rxjava3.ext.web.handler.PlatformHandler, Handler {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SessionHandler that = (SessionHandler) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new SessionHandler((io.vertx.ext.web.handler.SessionHandler) obj),
SessionHandler::getDelegate
);
private final io.vertx.ext.web.handler.SessionHandler delegate;
public SessionHandler(io.vertx.ext.web.handler.SessionHandler delegate) {
this.delegate = delegate;
}
public SessionHandler(Object delegate) {
this.delegate = (io.vertx.ext.web.handler.SessionHandler)delegate;
}
public io.vertx.ext.web.handler.SessionHandler getDelegate() {
return delegate;
}
/**
* Something has happened, so handle it.
* @param event the event to handle
*/
public void handle(io.vertx.rxjava3.ext.web.RoutingContext event) {
delegate.handle(event.getDelegate());
}
/**
* Create a session handler
* @param sessionStore the session store
* @return the handler
*/
public static io.vertx.rxjava3.ext.web.handler.SessionHandler create(io.vertx.rxjava3.ext.web.sstore.SessionStore sessionStore) {
io.vertx.rxjava3.ext.web.handler.SessionHandler ret = io.vertx.rxjava3.ext.web.handler.SessionHandler.newInstance((io.vertx.ext.web.handler.SessionHandler)io.vertx.ext.web.handler.SessionHandler.create(sessionStore.getDelegate()));
return ret;
}
/**
* Set the session timeout
* @param timeout the timeout, in ms.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setSessionTimeout(long timeout) {
delegate.setSessionTimeout(timeout);
return this;
}
/**
* Set whether a nagging log warning should be written if the session handler is
* accessed over HTTP, not HTTPS
* @param nag true to nag
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setNagHttps(boolean nag) {
delegate.setNagHttps(nag);
return this;
}
/**
* Sets whether the 'secure' flag should be set for the session cookie. When set
* this flag instructs browsers to only send the cookie over HTTPS. Note that
* this will probably stop your sessions working if used without HTTPS (e.g. in
* development).
* @param secure true to set the secure flag on the cookie
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setCookieSecureFlag(boolean secure) {
delegate.setCookieSecureFlag(secure);
return this;
}
/**
* Sets whether the 'HttpOnly' flag should be set for the session cookie. When
* set this flag instructs browsers to prevent Javascript access to the the
* cookie. Used as a line of defence against the most common XSS attacks.
* @param httpOnly true to set the HttpOnly flag on the cookie
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setCookieHttpOnlyFlag(boolean httpOnly) {
delegate.setCookieHttpOnlyFlag(httpOnly);
return this;
}
/**
* Set the session cookie name
* @param sessionCookieName the session cookie name
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setSessionCookieName(java.lang.String sessionCookieName) {
delegate.setSessionCookieName(sessionCookieName);
return this;
}
/**
* Set the session cookie path
* @param sessionCookiePath the session cookie path
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setSessionCookiePath(java.lang.String sessionCookiePath) {
delegate.setSessionCookiePath(sessionCookiePath);
return this;
}
/**
* Set expected session id minimum length.
* @param minLength the session id minimal length
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setMinLength(int minLength) {
delegate.setMinLength(minLength);
return this;
}
/**
* Set the session cookie SameSite policy to use.
* @param policy to use, null
for no policy.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setCookieSameSite(io.vertx.core.http.CookieSameSite policy) {
delegate.setCookieSameSite(policy);
return this;
}
/**
* Use a lazy session creation mechanism. The session will only be created when accessed from the context. Thus the
* session cookie is set only if the session was accessed.
* @param lazySession true to have a lazy session creation.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setLazySession(boolean lazySession) {
delegate.setLazySession(lazySession);
return this;
}
/**
* Set a Cookie max-age to the session cookie. When doing this the Cookie will be persistent across browser restarts.
* This can be dangerous as closing a browser windows does not invalidate the session. For more information refer to
* https://cheatsheetseries.owasp.org/cheatsheets/Session_Management_Cheat_Sheet.html#Expire_and_Max-Age_Attributes
* @param cookieMaxAge a non negative max-age, note that 0 means expire now.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setCookieMaxAge(long cookieMaxAge) {
delegate.setCookieMaxAge(cookieMaxAge);
return this;
}
/**
* Flush a context session earlier to the store, this will allow the end user to have full control on the event of
* a failure at the store level. Once a session is flushed no automatic save will be performed at end of request.
* @param ctx the current context
* @return fluent self
*/
public io.reactivex.rxjava3.core.Completable flush(io.vertx.rxjava3.ext.web.RoutingContext ctx) {
io.reactivex.rxjava3.core.Completable ret = rxFlush(ctx);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Flush a context session earlier to the store, this will allow the end user to have full control on the event of
* a failure at the store level. Once a session is flushed no automatic save will be performed at end of request.
* @param ctx the current context
* @return fluent self
*/
public io.reactivex.rxjava3.core.Completable rxFlush(io.vertx.rxjava3.ext.web.RoutingContext ctx) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.flush(ctx.getDelegate(), handler);
});
}
/**
* Flush a context session earlier to the store, this will allow the end user to have full control on the event of
* a failure at the store level. Once a session is flushed no automatic save will be performed at end of request.
* @param ctx the current context
* @param ignoreStatus flush regardless of response status code
* @return fluent self
*/
public io.reactivex.rxjava3.core.Completable flush(io.vertx.rxjava3.ext.web.RoutingContext ctx, boolean ignoreStatus) {
io.reactivex.rxjava3.core.Completable ret = rxFlush(ctx, ignoreStatus);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Flush a context session earlier to the store, this will allow the end user to have full control on the event of
* a failure at the store level. Once a session is flushed no automatic save will be performed at end of request.
* @param ctx the current context
* @param ignoreStatus flush regardless of response status code
* @return fluent self
*/
public io.reactivex.rxjava3.core.Completable rxFlush(io.vertx.rxjava3.ext.web.RoutingContext ctx, boolean ignoreStatus) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.flush(ctx.getDelegate(), ignoreStatus, handler);
});
}
/**
* Use sessions based on url paths instead of cookies. This is an potential less safe alternative to cookies
* but offers an alternative when Cookies are not desired, for example, to avoid showing banners on a website
* due to cookie laws, or doing machine to machine operations where state is required to maintain.
* @param cookieless true if a cookieless session should be used
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.ext.web.handler.SessionHandler setCookieless(boolean cookieless) {
delegate.setCookieless(cookieless);
return this;
}
/**
* Create a new session
* @param context the routing context
* @return the session
*/
public io.vertx.rxjava3.ext.web.Session newSession(io.vertx.rxjava3.ext.web.RoutingContext context) {
io.vertx.rxjava3.ext.web.Session ret = io.vertx.rxjava3.ext.web.Session.newInstance((io.vertx.ext.web.Session)delegate.newSession(context.getDelegate()));
return ret;
}
/**
* Set the user for the session
* @param context the routing context
* @param user the user
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable setUser(io.vertx.rxjava3.ext.web.RoutingContext context, io.vertx.rxjava3.ext.auth.User user) {
io.reactivex.rxjava3.core.Completable ret = rxSetUser(context, user);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Set the user for the session
* @param context the routing context
* @param user the user
* @return a reference to this, so the API can be used fluently
*/
public io.reactivex.rxjava3.core.Completable rxSetUser(io.vertx.rxjava3.ext.web.RoutingContext context, io.vertx.rxjava3.ext.auth.User user) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.setUser(context.getDelegate(), user.getDelegate(), handler);
});
}
/**
* Default name of session cookie
*/
public static final java.lang.String DEFAULT_SESSION_COOKIE_NAME = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSION_COOKIE_NAME;
/**
* Default path of session cookie
*/
public static final java.lang.String DEFAULT_SESSION_COOKIE_PATH = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSION_COOKIE_PATH;
/**
* Default time, in ms, that a session lasts for without being accessed before
* expiring.
*/
public static final long DEFAULT_SESSION_TIMEOUT = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSION_TIMEOUT;
/**
* Default of whether a nagging log warning should be written if the session
* handler is accessed over HTTP, not HTTPS
*/
public static final boolean DEFAULT_NAG_HTTPS = io.vertx.ext.web.handler.SessionHandler.DEFAULT_NAG_HTTPS;
/**
* Default of whether the cookie has the HttpOnly flag set More info:
* https://www.owasp.org/index.php/HttpOnly
*/
public static final boolean DEFAULT_COOKIE_HTTP_ONLY_FLAG = io.vertx.ext.web.handler.SessionHandler.DEFAULT_COOKIE_HTTP_ONLY_FLAG;
/**
* Default of whether the cookie has the 'secure' flag set to allow transmission
* over https only. More info: https://www.owasp.org/index.php/SecureFlag
*/
public static final boolean DEFAULT_COOKIE_SECURE_FLAG = io.vertx.ext.web.handler.SessionHandler.DEFAULT_COOKIE_SECURE_FLAG;
/**
* Default min length for a session id. More info:
* https://www.owasp.org/index.php/Session_Management_Cheat_Sheet
*/
public static final int DEFAULT_SESSIONID_MIN_LENGTH = io.vertx.ext.web.handler.SessionHandler.DEFAULT_SESSIONID_MIN_LENGTH;
/**
* Default of whether the session should be created lazily.
*/
public static final boolean DEFAULT_LAZY_SESSION = io.vertx.ext.web.handler.SessionHandler.DEFAULT_LAZY_SESSION;
public static SessionHandler newInstance(io.vertx.ext.web.handler.SessionHandler arg) {
return arg != null ? new SessionHandler(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy