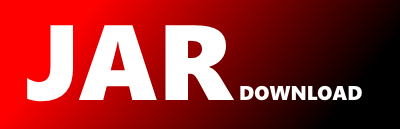
io.vertx.rxjava3.ext.web.openapi.RouterBuilder Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.ext.web.openapi;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Interface to build a Vert.x Web from an OpenAPI 3 contract.
* To add an handler, use {@link io.vertx.rxjava3.ext.web.openapi.RouterBuilder#operation} (String, Handler)}
* Usage example:
*
* RouterBuilder.create(vertx, "src/resources/spec.yaml", asyncResult -> {
if (!asyncResult.succeeded()) {
// IO failure or spec invalid
else {
* RouterBuilder routerBuilder = asyncResult.result();
* RouterBuilder.operation("operation_id").handler(routingContext -> {
* // Do something
* }, routingContext -> {
* // Do something with failure handler
* });
* Router router = routerBuilder.createRouter();
* }
* });
* }
*
*
* Handlers are loaded in this order:
*
* - Body handler (Customizable with
* - Custom global handlers configurable with
* - Global security handlers defined in upper spec level
* - Operation specific security handlers
* - Generated validation handler
* - User handlers or "Not implemented" handler
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.openapi.RouterBuilder original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.ext.web.openapi.RouterBuilder.class)
public class RouterBuilder {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RouterBuilder that = (RouterBuilder) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new RouterBuilder((io.vertx.ext.web.openapi.RouterBuilder) obj),
RouterBuilder::getDelegate
);
private final io.vertx.ext.web.openapi.RouterBuilder delegate;
public RouterBuilder(io.vertx.ext.web.openapi.RouterBuilder delegate) {
this.delegate = delegate;
}
public RouterBuilder(Object delegate) {
this.delegate = (io.vertx.ext.web.openapi.RouterBuilder)delegate;
}
public io.vertx.ext.web.openapi.RouterBuilder getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.ext.web.openapi.Operation.newInstance((io.vertx.ext.web.openapi.Operation)o1), o1 -> o1.getDelegate());
/**
* Access to an operation defined in the contract with operationId
* @param operationId the id of the operation
* @return the requested operation
*/
public io.vertx.rxjava3.ext.web.openapi.Operation operation(java.lang.String operationId) {
io.vertx.rxjava3.ext.web.openapi.Operation ret = io.vertx.rxjava3.ext.web.openapi.Operation.newInstance((io.vertx.ext.web.openapi.Operation)delegate.operation(operationId));
return ret;
}
/**
* @return all operations defined in the contract
*/
public java.util.List operations() {
java.util.List ret = delegate.operations().stream().map(elt -> io.vertx.rxjava3.ext.web.openapi.Operation.newInstance((io.vertx.ext.web.openapi.Operation)elt)).collect(Collectors.toList());
return ret;
}
/**
* Supply your own BodyHandler if you would like to control body limit, uploads directory and deletion of uploaded
* files.
* If you provide a null body handler, you won't be able to validate request bodies
* @param bodyHandler
* @return self
*/
@Deprecated()
public io.vertx.rxjava3.ext.web.openapi.RouterBuilder bodyHandler(io.vertx.rxjava3.ext.web.handler.BodyHandler bodyHandler) {
delegate.bodyHandler(bodyHandler.getDelegate());
return this;
}
/**
* Add global handler to be applied prior to being generated.
* Please note that you should not add a body handler inside that list. If you want to modify the body handler,
* please use {@link io.vertx.rxjava3.ext.web.openapi.RouterBuilder#bodyHandler}
* @param rootHandler
* @return self
*/
public io.vertx.rxjava3.ext.web.openapi.RouterBuilder rootHandler(io.vertx.core.Handler rootHandler) {
delegate.rootHandler(new io.vertx.lang.rx.DelegatingHandler<>(rootHandler, event -> io.vertx.rxjava3.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)event)));
return this;
}
/**
* Introspect the OpenAPI spec to mount handlers for all operations that specifies a x-vertx-event-bus annotation.
* Please give a look at
* vertx-web-api-service documentation
* for more informations
* @return self
*/
public io.vertx.rxjava3.ext.web.openapi.RouterBuilder mountServicesFromExtensions() {
delegate.mountServicesFromExtensions();
return this;
}
/**
* Set options of router builder. For more info {@link io.vertx.ext.web.openapi.RouterBuilderOptions}
* @param options
* @return self
*/
public io.vertx.rxjava3.ext.web.openapi.RouterBuilder setOptions(io.vertx.ext.web.openapi.RouterBuilderOptions options) {
delegate.setOptions(options);
return this;
}
/**
* @return options of router builder. For more info {@link io.vertx.ext.web.openapi.RouterBuilderOptions}
*/
public io.vertx.ext.web.openapi.RouterBuilderOptions getOptions() {
io.vertx.ext.web.openapi.RouterBuilderOptions ret = delegate.getOptions();
return ret;
}
/**
* @return holder used by self to process the OpenAPI. You can use it to resolve $ref
s
*/
public io.vertx.rxjava3.ext.web.openapi.OpenAPIHolder getOpenAPI() {
io.vertx.rxjava3.ext.web.openapi.OpenAPIHolder ret = io.vertx.rxjava3.ext.web.openapi.OpenAPIHolder.newInstance((io.vertx.ext.web.openapi.OpenAPIHolder)delegate.getOpenAPI());
return ret;
}
/**
* @return schema router used by self to internally manage all {@link io.vertx.rxjava3.json.schema.Schema} instances
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaRouter getSchemaRouter() {
io.vertx.rxjava3.json.schema.SchemaRouter ret = io.vertx.rxjava3.json.schema.SchemaRouter.newInstance((io.vertx.json.schema.SchemaRouter)delegate.getSchemaRouter());
return ret;
}
/**
* @return schema parser used by self to parse all {@link io.vertx.rxjava3.json.schema.Schema}
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaParser getSchemaParser() {
io.vertx.rxjava3.json.schema.SchemaParser ret = io.vertx.rxjava3.json.schema.SchemaParser.newInstance((io.vertx.json.schema.SchemaParser)delegate.getSchemaParser());
return ret;
}
/**
* When set, this function is called while creating the payload of
* @param serviceExtraPayloadMapper
* @return self
*/
public io.vertx.rxjava3.ext.web.openapi.RouterBuilder serviceExtraPayloadMapper(java.util.function.Function serviceExtraPayloadMapper) {
delegate.serviceExtraPayloadMapper(new Function() {
public io.vertx.core.json.JsonObject apply(io.vertx.ext.web.RoutingContext arg) {
io.vertx.core.json.JsonObject ret = serviceExtraPayloadMapper.apply(io.vertx.rxjava3.ext.web.RoutingContext.newInstance((io.vertx.ext.web.RoutingContext)arg));
return ret;
}
});
return this;
}
/**
* Creates a new security scheme for the required .
* @param securitySchemeName
* @return a security scheme.
*/
public io.vertx.rxjava3.ext.web.openapi.SecurityScheme securityHandler(java.lang.String securitySchemeName) {
io.vertx.rxjava3.ext.web.openapi.SecurityScheme ret = io.vertx.rxjava3.ext.web.openapi.SecurityScheme.newInstance((io.vertx.ext.web.openapi.SecurityScheme)delegate.securityHandler(securitySchemeName));
return ret;
}
/**
* Construct a new router based on spec. It will fail if you are trying to mount a spec with security schemes
* without assigned handlers
*
* Note: Router is built when this function is called and the path definition ordering in contract is respected.
* @return
*/
public io.vertx.rxjava3.ext.web.Router createRouter() {
io.vertx.rxjava3.ext.web.Router ret = io.vertx.rxjava3.ext.web.Router.newInstance((io.vertx.ext.web.Router)delegate.createRouter());
return ret;
}
/**
* Like
* @param vertx
* @param url
* @return
*/
public static io.reactivex.rxjava3.core.Single create(io.vertx.rxjava3.core.Vertx vertx, java.lang.String url) {
io.reactivex.rxjava3.core.Single ret = rxCreate(vertx, url);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like
* @param vertx
* @param url
* @return
*/
public static io.reactivex.rxjava3.core.Single rxCreate(io.vertx.rxjava3.core.Vertx vertx, java.lang.String url) {
return AsyncResultSingle.toSingle( handler -> {
io.vertx.ext.web.openapi.RouterBuilder.create(vertx.getDelegate(), url, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.openapi.RouterBuilder.newInstance((io.vertx.ext.web.openapi.RouterBuilder)event))));
});
}
/**
* Like
* @param vertx
* @param url
* @param options
* @return
*/
public static io.reactivex.rxjava3.core.Single create(io.vertx.rxjava3.core.Vertx vertx, java.lang.String url, io.vertx.ext.web.openapi.OpenAPILoaderOptions options) {
io.reactivex.rxjava3.core.Single ret = rxCreate(vertx, url, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like
* @param vertx
* @param url
* @param options
* @return
*/
public static io.reactivex.rxjava3.core.Single rxCreate(io.vertx.rxjava3.core.Vertx vertx, java.lang.String url, io.vertx.ext.web.openapi.OpenAPILoaderOptions options) {
return AsyncResultSingle.toSingle( handler -> {
io.vertx.ext.web.openapi.RouterBuilder.create(vertx.getDelegate(), url, options, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.ext.web.openapi.RouterBuilder.newInstance((io.vertx.ext.web.openapi.RouterBuilder)event))));
});
}
/**
* Mount to paths that have to follow a security schema a security handler. This method will not perform any
* validation weather or not the given securitySchemeName
is present in the OpenAPI document.
*
* For must use cases the method {@link io.vertx.rxjava3.ext.web.openapi.RouterBuilder#securityHandler} should be used.
* @param securitySchemeName the components security scheme id
* @param handler the authentication handler
* @return self
*/
public io.vertx.rxjava3.ext.web.openapi.RouterBuilder securityHandler(java.lang.String securitySchemeName, io.vertx.rxjava3.ext.web.handler.AuthenticationHandler handler) {
delegate.securityHandler(securitySchemeName, handler.getDelegate());
return this;
}
public static RouterBuilder newInstance(io.vertx.ext.web.openapi.RouterBuilder arg) {
return arg != null ? new RouterBuilder(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy