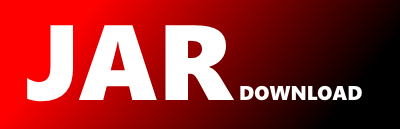
io.vertx.rxjava3.grpc.client.GrpcClientRequest Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.grpc.client;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A request to a gRPC server.
*
* You interact with the remote service with gRPC generated messages or protobuf encoded messages.
*
*
Before sending a request you need to set {@link io.vertx.rxjava3.grpc.client.GrpcClientRequest #serviceName)} and {@link io.vertx.rxjava3.grpc.client.GrpcClientRequest #methodName)} or
* alternatively the service {@link io.vertx.rxjava3.grpc.client.GrpcClientRequest#fullMethodName}.
*
*
Writing a request message will send the request to the service:
*
*
* - To send a unary request, just call
* - To send a streaming request, call any time you need and then {@link io.vertx.rxjava3.core.streams.WriteStream#end}
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.grpc.client.GrpcClientRequest original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.grpc.client.GrpcClientRequest.class)
public class GrpcClientRequest extends io.vertx.rxjava3.grpc.common.GrpcWriteStream {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
GrpcClientRequest that = (GrpcClientRequest) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new GrpcClientRequest((io.vertx.grpc.client.GrpcClientRequest) obj),
GrpcClientRequest::getDelegate
);
private final io.vertx.grpc.client.GrpcClientRequest delegate;
public final TypeArg __typeArg_0;
public final TypeArg __typeArg_1;
public GrpcClientRequest(io.vertx.grpc.client.GrpcClientRequest delegate) {
super(delegate);
this.delegate = delegate;
this.__typeArg_0 = TypeArg.unknown(); this.__typeArg_1 = TypeArg.unknown(); }
public GrpcClientRequest(Object delegate, TypeArg typeArg_0, TypeArg typeArg_1) {
super((io.vertx.grpc.client.GrpcClientRequest)delegate);
this.delegate = (io.vertx.grpc.client.GrpcClientRequest)delegate;
this.__typeArg_0 = typeArg_0;
this.__typeArg_1 = typeArg_1;
}
public io.vertx.grpc.client.GrpcClientRequest getDelegate() {
return delegate;
}
private WriteStreamObserver observer;
private WriteStreamSubscriber subscriber;
public synchronized WriteStreamObserver toObserver() {
if (observer == null) {
Function conv = (Function) __typeArg_0.unwrap;
observer = RxHelper.toObserver(getDelegate(), conv);
}
return observer;
}
public synchronized WriteStreamSubscriber toSubscriber() {
if (subscriber == null) {
Function conv = (Function) __typeArg_0.unwrap;
subscriber = RxHelper.toSubscriber(getDelegate(), conv);
}
return subscriber;
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable write(Req data) {
io.reactivex.rxjava3.core.Completable ret = rxWrite(data);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWrite(Req data) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.write(__typeArg_0.unwrap(data), handler);
});
}
/**
* Same as {@link io.vertx.rxjava3.core.streams.WriteStream#end} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Completable end() {
io.reactivex.rxjava3.core.Completable ret = rxEnd();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as {@link io.vertx.rxjava3.core.streams.WriteStream#end} but with an handler
called when the operation completes
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(handler);
});
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable end(Req data) {
io.reactivex.rxjava3.core.Completable ret = rxEnd(data);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Same as but with an handler
called when the operation completes
* @param data
* @return
*/
public io.reactivex.rxjava3.core.Completable rxEnd(Req data) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.end(__typeArg_0.unwrap(data), handler);
});
}
/**
* This will return true
if there are more bytes in the write queue than the value set using {@link io.vertx.rxjava3.grpc.client.GrpcClientRequest#setWriteQueueMaxSize}
* @return true
if write queue is full
*/
public boolean writeQueueFull() {
boolean ret = delegate.writeQueueFull();
return ret;
}
public io.vertx.rxjava3.grpc.client.GrpcClientRequest encoding(java.lang.String encoding) {
delegate.encoding(encoding);
return this;
}
/**
* Set the full method name to call, it must follow the format package-name + '.' + service-name + '/' + method-name
* or an IllegalArgumentException
is thrown.
*
* It must be called before sending the request otherwise an IllegalStateException
is thrown.
* @param fullMethodName the full method name to call
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.grpc.client.GrpcClientRequest fullMethodName(java.lang.String fullMethodName) {
delegate.fullMethodName(fullMethodName);
return this;
}
/**
* Set the service name to call.
*
* It must be called before sending the request otherwise an IllegalStateException
is thrown.
* @param serviceName the service name to call
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.grpc.client.GrpcClientRequest serviceName(io.vertx.rxjava3.grpc.common.ServiceName serviceName) {
delegate.serviceName(serviceName.getDelegate());
return this;
}
/**
* Set the method name to call.
*
* It must be called before sending the request otherwise an IllegalStateException
is thrown.
* @param methodName the method name to call
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.grpc.client.GrpcClientRequest methodName(java.lang.String methodName) {
delegate.methodName(methodName);
return this;
}
/**
* @return the gRPC response
*/
public io.reactivex.rxjava3.core.Single> response() {
io.reactivex.rxjava3.core.Single> ret = rxResponse();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* @return the gRPC response
*/
public io.reactivex.rxjava3.core.Single> rxResponse() {
return AsyncResultSingle.toSingle(delegate.response(), __value -> io.vertx.rxjava3.grpc.client.GrpcClientResponse.newInstance((io.vertx.grpc.client.GrpcClientResponse)__value, __typeArg_0, __typeArg_1));
}
public io.vertx.rxjava3.grpc.client.GrpcClientRequest exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
public io.vertx.rxjava3.grpc.client.GrpcClientRequest setWriteQueueMaxSize(int maxSize) {
delegate.setWriteQueueMaxSize(maxSize);
return this;
}
public io.vertx.rxjava3.grpc.client.GrpcClientRequest drainHandler(io.vertx.core.Handler handler) {
delegate.drainHandler(handler);
return this;
}
/**
* Sets the amount of time after which, if the request does not return any data within the timeout period,
* the request/response is cancelled and the related futures.
* @param timeout the amount of time in milliseconds.
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.grpc.client.GrpcClientRequest idleTimeout(long timeout) {
delegate.idleTimeout(timeout);
return this;
}
/**
* @return the underlying HTTP connection
*/
public io.vertx.rxjava3.core.http.HttpConnection connection() {
io.vertx.rxjava3.core.http.HttpConnection ret = io.vertx.rxjava3.core.http.HttpConnection.newInstance((io.vertx.core.http.HttpConnection)delegate.connection());
return ret;
}
public io.reactivex.rxjava3.core.Single> send(Req item) {
io.reactivex.rxjava3.core.Single> ret = rxSend(item);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single> rxSend(Req item) {
return AsyncResultSingle.toSingle(delegate.send(__typeArg_0.unwrap(item)), __value -> io.vertx.rxjava3.grpc.client.GrpcClientResponse.newInstance((io.vertx.grpc.client.GrpcClientResponse)__value, __typeArg_0, __typeArg_1));
}
public io.reactivex.rxjava3.core.Single> send(io.reactivex.rxjava3.core.Flowable body) {
io.reactivex.rxjava3.core.Single> ret = rxSend(body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
public io.reactivex.rxjava3.core.Single> rxSend(io.reactivex.rxjava3.core.Flowable body) {
return AsyncResultSingle.toSingle(delegate.send(io.vertx.rxjava3.impl.ReadStreamSubscriber.asReadStream(body, obj -> __typeArg_0.unwrap(obj)).resume()), __value -> io.vertx.rxjava3.grpc.client.GrpcClientResponse.newInstance((io.vertx.grpc.client.GrpcClientResponse)__value, __typeArg_0, __typeArg_1));
}
public static GrpcClientRequest newInstance(io.vertx.grpc.client.GrpcClientRequest arg) {
return arg != null ? new GrpcClientRequest(arg) : null;
}
public static GrpcClientRequest newInstance(io.vertx.grpc.client.GrpcClientRequest arg, TypeArg __typeArg_Req, TypeArg __typeArg_Resp) {
return arg != null ? new GrpcClientRequest(arg, __typeArg_Req, __typeArg_Resp) : null;
}
}