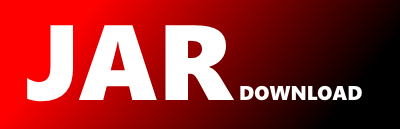
io.vertx.rxjava3.json.schema.SchemaRepository Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.json.schema;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A repository is a holder of dereferenced schemas, it can be used to create validator instances for a specific schema.
*
* This is to be used when multiple schema objects compose the global schema to be used for validation.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.json.schema.SchemaRepository original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.json.schema.SchemaRepository.class)
public class SchemaRepository {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SchemaRepository that = (SchemaRepository) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new SchemaRepository((io.vertx.json.schema.SchemaRepository) obj),
SchemaRepository::getDelegate
);
private final io.vertx.json.schema.SchemaRepository delegate;
public SchemaRepository(io.vertx.json.schema.SchemaRepository delegate) {
this.delegate = delegate;
}
public SchemaRepository(Object delegate) {
this.delegate = (io.vertx.json.schema.SchemaRepository)delegate;
}
public io.vertx.json.schema.SchemaRepository getDelegate() {
return delegate;
}
/**
* Create a repository with some initial configuration.
* @param options the initial configuration
* @return a repository
*/
public static io.vertx.rxjava3.json.schema.SchemaRepository create(io.vertx.json.schema.JsonSchemaOptions options) {
io.vertx.rxjava3.json.schema.SchemaRepository ret = io.vertx.rxjava3.json.schema.SchemaRepository.newInstance((io.vertx.json.schema.SchemaRepository)io.vertx.json.schema.SchemaRepository.create(options));
return ret;
}
/**
* Create a repository with some initial configuration.
* @param options the initial configuration
* @param jsonFormatValidator
* @return a repository
*/
public static io.vertx.rxjava3.json.schema.SchemaRepository create(io.vertx.json.schema.JsonSchemaOptions options, io.vertx.rxjava3.json.schema.JsonFormatValidator jsonFormatValidator) {
io.vertx.rxjava3.json.schema.SchemaRepository ret = io.vertx.rxjava3.json.schema.SchemaRepository.newInstance((io.vertx.json.schema.SchemaRepository)io.vertx.json.schema.SchemaRepository.create(options, jsonFormatValidator.getDelegate()));
return ret;
}
/**
* Dereferences a schema to the repository.
* @param schema a new schema to list
* @return a repository
*/
public io.vertx.rxjava3.json.schema.SchemaRepository dereference(io.vertx.rxjava3.json.schema.JsonSchema schema) {
delegate.dereference(schema.getDelegate());
return this;
}
/**
* Dereferences a schema to the repository.
* @param uri the source of the schema used for de-referencing, optionally relative to {@link io.vertx.json.schema.JsonSchemaOptions}.
* @param schema a new schema to list
* @return a repository
*/
public io.vertx.rxjava3.json.schema.SchemaRepository dereference(java.lang.String uri, io.vertx.rxjava3.json.schema.JsonSchema schema) {
delegate.dereference(uri, schema.getDelegate());
return this;
}
/**
* Preloads the repository with the meta schemas for the related @link {@link io.vertx.json.schema.Draft} version. The related draft version
* is determined from the {@link io.vertx.json.schema.JsonSchemaOptions}, in case that no draft is set in the options an
* {@link java.lang.IllegalStateException} is thrown.
* @param fs The Vert.x file system to load the related schema meta files from classpath
* @return a repository
*/
public io.vertx.rxjava3.json.schema.SchemaRepository preloadMetaSchema(io.vertx.rxjava3.core.file.FileSystem fs) {
delegate.preloadMetaSchema(fs.getDelegate());
return this;
}
/**
* Preloads the repository with the meta schemas for the related draft version.
* @param fs The Vert.x file system to load the related schema meta files from classpath
* @param draft The draft version of the meta files to load
* @return a repository
*/
public io.vertx.rxjava3.json.schema.SchemaRepository preloadMetaSchema(io.vertx.rxjava3.core.file.FileSystem fs, io.vertx.json.schema.Draft draft) {
delegate.preloadMetaSchema(fs.getDelegate(), draft);
return this;
}
/**
* A new validator instance using this repository options.
* @param schema the start validation schema
* @return the validator
*/
public io.vertx.rxjava3.json.schema.Validator validator(io.vertx.rxjava3.json.schema.JsonSchema schema) {
io.vertx.rxjava3.json.schema.Validator ret = io.vertx.rxjava3.json.schema.Validator.newInstance((io.vertx.json.schema.Validator)delegate.validator(schema.getDelegate()));
return ret;
}
/**
* A new validator instance using this repository options. This is the preferred way
* to create a validator as it avoids reparsing schemas and reuses the cache in the
* repository.
* @param ref the start validation reference in JSON pointer format
* @return the validator
*/
public io.vertx.rxjava3.json.schema.Validator validator(java.lang.String ref) {
io.vertx.rxjava3.json.schema.Validator ret = io.vertx.rxjava3.json.schema.Validator.newInstance((io.vertx.json.schema.Validator)delegate.validator(ref));
return ret;
}
/**
* A new validator instance overriding this repository options. This is the preferred way
* to create a validator as it avoids reparsing schemas and reuses the cache in the
* repository.
* @param ref the start validation reference in JSON pointer format
* @param options the options to be using on the validator instance
* @return the validator
*/
public io.vertx.rxjava3.json.schema.Validator validator(java.lang.String ref, io.vertx.json.schema.JsonSchemaOptions options) {
io.vertx.rxjava3.json.schema.Validator ret = io.vertx.rxjava3.json.schema.Validator.newInstance((io.vertx.json.schema.Validator)delegate.validator(ref, options));
return ret;
}
/**
* A new validator instance overriding this repository options.
* The given schema will not be referenced to the repository.
* @param schema the start validation schema
* @param options the options to be using on the validator instance
* @return the validator
*/
public io.vertx.rxjava3.json.schema.Validator validator(io.vertx.rxjava3.json.schema.JsonSchema schema, io.vertx.json.schema.JsonSchemaOptions options) {
io.vertx.rxjava3.json.schema.Validator ret = io.vertx.rxjava3.json.schema.Validator.newInstance((io.vertx.json.schema.Validator)delegate.validator(schema.getDelegate(), options));
return ret;
}
/**
* A new validator instance overriding this repository options.
* @param schema the start validation schema
* @param options the options to be using on the validator instance
* @param dereference if true the schema will be dereferenced before validation
* @return the validator
*/
public io.vertx.rxjava3.json.schema.Validator validator(io.vertx.rxjava3.json.schema.JsonSchema schema, io.vertx.json.schema.JsonSchemaOptions options, boolean dereference) {
io.vertx.rxjava3.json.schema.Validator ret = io.vertx.rxjava3.json.schema.Validator.newInstance((io.vertx.json.schema.Validator)delegate.validator(schema.getDelegate(), options, dereference));
return ret;
}
/**
* Resolve all $ref
in the given . The resolution algrithm is not aware of other
* specifications. When resolving OpenAPI documents (which only allow $ref
at specific locations) you
* should validate if the document is valid before performing a resolution.
*
* It is important to note that any sibling elements of a $ref
is ignored. This is because $ref
* works by replacing itself and everything on its level with the definition it is pointing at.
* @param schema the JSON object to resolve.
* @return a new JSON object with all the $ref
replaced by actual object references.
*/
public io.vertx.core.json.JsonObject resolve(io.vertx.core.json.JsonObject schema) {
io.vertx.core.json.JsonObject ret = delegate.resolve(schema);
return ret;
}
/**
* Tries to resolve all internal and repository local references. External references are not resolved.
*
* The result is an object where all references have been resolved. Resolution of circular references is shallow. This
* should normally not be a problem for this use case.
* @param ref the start resolution reference in JSON pointer format
* @return a new representing the schema with $ref
s replaced by their value.
*/
public io.vertx.core.json.JsonObject resolve(java.lang.String ref) {
io.vertx.core.json.JsonObject ret = delegate.resolve(ref);
return ret;
}
/**
* Tries to resolve all internal and repository local references. External references are not resolved.
*
* The result is an object where all references have been resolved. Resolution of circular references is shallow. This
* should normally not be a problem for this use case.
* @param schema
* @return a new representing the schema with $ref
s replaced by their value.
*/
public io.vertx.core.json.JsonObject resolve(io.vertx.rxjava3.json.schema.JsonSchema schema) {
io.vertx.core.json.JsonObject ret = delegate.resolve(schema.getDelegate());
return ret;
}
/**
* Look up a schema using a JSON pointer notation
* @param pointer the JSON pointer
* @return the schema
*/
public io.vertx.rxjava3.json.schema.JsonSchema find(java.lang.String pointer) {
io.vertx.rxjava3.json.schema.JsonSchema ret = io.vertx.rxjava3.json.schema.JsonSchema.newInstance((io.vertx.json.schema.JsonSchema)delegate.find(pointer));
return ret;
}
public static SchemaRepository newInstance(io.vertx.json.schema.SchemaRepository arg) {
return arg != null ? new SchemaRepository(arg) : null;
}
}