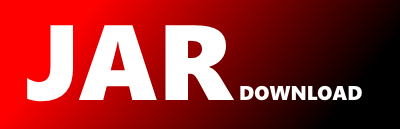
io.vertx.rxjava3.json.schema.SchemaRouter Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.json.schema;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Represents a pool where parsed schemas are addressed and cached.
*
* It also contains a cache of including on top or inner level some json schemas that could eventually parsed later.
*
* You should not share this object between different threads
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.json.schema.SchemaRouter original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.json.schema.SchemaRouter.class)
public class SchemaRouter {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
SchemaRouter that = (SchemaRouter) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new SchemaRouter((io.vertx.json.schema.SchemaRouter) obj),
SchemaRouter::getDelegate
);
private final io.vertx.json.schema.SchemaRouter delegate;
public SchemaRouter(io.vertx.json.schema.SchemaRouter delegate) {
this.delegate = delegate;
}
public SchemaRouter(Object delegate) {
this.delegate = (io.vertx.json.schema.SchemaRouter)delegate;
}
public io.vertx.json.schema.SchemaRouter getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.json.schema.Schema.newInstance((io.vertx.json.schema.Schema)o1), o1 -> o1.getDelegate());
/**
* Resolve cached schema based on refPointer. If a schema isn't cached, it returns null
* @param refPointer
* @param schemaScope
* @param parser
* @return the resolved schema, or null if no schema was found
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.Schema resolveCachedSchema(io.vertx.rxjava3.core.json.pointer.JsonPointer refPointer, io.vertx.rxjava3.core.json.pointer.JsonPointer schemaScope, io.vertx.rxjava3.json.schema.SchemaParser parser) {
io.vertx.rxjava3.json.schema.Schema ret = io.vertx.rxjava3.json.schema.Schema.newInstance((io.vertx.json.schema.Schema)delegate.resolveCachedSchema(refPointer.getDelegate(), schemaScope.getDelegate(), parser.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava3.json.schema.SchemaRouter#resolveRef} but with a direct callback.
* @param pointer
* @param scope
* @param schemaParser
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single resolveRef(io.vertx.rxjava3.core.json.pointer.JsonPointer pointer, io.vertx.rxjava3.core.json.pointer.JsonPointer scope, io.vertx.rxjava3.json.schema.SchemaParser schemaParser) {
io.reactivex.rxjava3.core.Single ret = rxResolveRef(pointer, scope, schemaParser);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.json.schema.SchemaRouter#resolveRef} but with a direct callback.
* @param pointer
* @param scope
* @param schemaParser
* @return
*/
@Deprecated()
public io.reactivex.rxjava3.core.Single rxResolveRef(io.vertx.rxjava3.core.json.pointer.JsonPointer pointer, io.vertx.rxjava3.core.json.pointer.JsonPointer scope, io.vertx.rxjava3.json.schema.SchemaParser schemaParser) {
return AsyncResultSingle.toSingle( handler -> {
delegate.resolveRef(pointer.getDelegate(), scope.getDelegate(), schemaParser.getDelegate(), new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.json.schema.Schema.newInstance((io.vertx.json.schema.Schema)event))));
});
}
/**
* Add a parsed schema to this router. When a schema is added to the cache, a new entry is created for the provided scope,
* but NOT for {@link io.vertx.rxjava3.json.schema.Schema#getScope}. This may be useful to register links to singleton schemas.
* This method is automatically called by {@link io.vertx.rxjava3.json.schema.SchemaParser} when a new schema is parsed
* @param schema schema to add
* @param scope
* @return a reference to this
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaRouter addSchemaWithScope(io.vertx.rxjava3.json.schema.Schema schema, io.vertx.rxjava3.core.json.pointer.JsonPointer scope) {
delegate.addSchemaWithScope(schema.getDelegate(), scope.getDelegate());
return this;
}
/**
* Add an alias to a schema already registered in this router (this alias can be solved only from schema scope).
* @param schema schema to add
* @param alias the schema alias
* @return a reference to this
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaRouter addSchemaAlias(io.vertx.rxjava3.json.schema.Schema schema, java.lang.String alias) {
delegate.addSchemaAlias(schema.getDelegate(), alias);
return this;
}
/**
* Add one or more json documents including schemas on top or inner levels. This method doesn't trigger the schema parsing
*
* You can use this schema if you have externally loaded some json document and you want to register to the schema router.
* You can later parse and retrieve a schema from this json structure using ,
* providing the correct refPointer
* @param uri
* @param object
* @return a reference to this
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaRouter addJson(java.lang.String uri, io.vertx.core.json.JsonObject object) {
delegate.addJson(uri, object);
return this;
}
/**
* @return a list of all registered schemas
*/
@Deprecated()
public java.util.List registeredSchemas() {
java.util.List ret = delegate.registeredSchemas().stream().map(elt -> io.vertx.rxjava3.json.schema.Schema.newInstance((io.vertx.json.schema.Schema)elt)).collect(Collectors.toList());
return ret;
}
/**
* Create a new {@link io.vertx.rxjava3.json.schema.SchemaRouter}
* @param vertx
* @param schemaRouterOptions
* @return
*/
@Deprecated()
public static io.vertx.rxjava3.json.schema.SchemaRouter create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.json.schema.SchemaRouterOptions schemaRouterOptions) {
io.vertx.rxjava3.json.schema.SchemaRouter ret = io.vertx.rxjava3.json.schema.SchemaRouter.newInstance((io.vertx.json.schema.SchemaRouter)io.vertx.json.schema.SchemaRouter.create(vertx.getDelegate(), schemaRouterOptions));
return ret;
}
/**
* Create a new {@link io.vertx.rxjava3.json.schema.SchemaRouter}
* @param vertx
* @param client
* @param fs
* @param schemaRouterOptions
* @return
*/
@Deprecated()
public static io.vertx.rxjava3.json.schema.SchemaRouter create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.rxjava3.core.http.HttpClient client, io.vertx.rxjava3.core.file.FileSystem fs, io.vertx.json.schema.SchemaRouterOptions schemaRouterOptions) {
io.vertx.rxjava3.json.schema.SchemaRouter ret = io.vertx.rxjava3.json.schema.SchemaRouter.newInstance((io.vertx.json.schema.SchemaRouter)io.vertx.json.schema.SchemaRouter.create(vertx.getDelegate(), client.getDelegate(), fs.getDelegate(), schemaRouterOptions));
return ret;
}
/**
* Add a parsed schema to this router. When a schema is added to the cache, a new entry is created for {@link io.vertx.rxjava3.json.schema.Schema#getScope} and,
* if you provide additional aliasScopes, this method register links to this schema with these scopes.
* This method is automatically called by {@link io.vertx.rxjava3.json.schema.SchemaParser} when a new schema is parsed
* @param schema schema to add
* @param aliasScopes
* @return a reference to this
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaRouter addSchema(io.vertx.rxjava3.json.schema.Schema schema, io.vertx.core.json.pointer.JsonPointer[] aliasScopes) {
delegate.addSchema(schema.getDelegate(), aliasScopes);
return this;
}
/**
* Add one or more json documents including schemas on top or inner levels. This method doesn't trigger the schema parsing
*
* You can use this schema if you have externally loaded some json document and you want to register to the schema router.
* You can later parse and retrieve a schema from this json structure using ,
* providing the correct refPointer
* @param uri
* @param object
* @return a reference to this
*/
@Deprecated()
public io.vertx.rxjava3.json.schema.SchemaRouter addJson(java.net.URI uri, io.vertx.core.json.JsonObject object) {
delegate.addJson(uri, object);
return this;
}
public static SchemaRouter newInstance(io.vertx.json.schema.SchemaRouter arg) {
return arg != null ? new SchemaRouter(arg) : null;
}
}