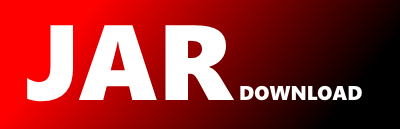
io.vertx.rxjava3.kafka.admin.KafkaAdminClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.kafka.admin;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Vert.x Kafka Admin client implementation
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.kafka.admin.KafkaAdminClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.kafka.admin.KafkaAdminClient.class)
public class KafkaAdminClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
KafkaAdminClient that = (KafkaAdminClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new KafkaAdminClient((io.vertx.kafka.admin.KafkaAdminClient) obj),
KafkaAdminClient::getDelegate
);
private final io.vertx.kafka.admin.KafkaAdminClient delegate;
public KafkaAdminClient(io.vertx.kafka.admin.KafkaAdminClient delegate) {
this.delegate = delegate;
}
public KafkaAdminClient(Object delegate) {
this.delegate = (io.vertx.kafka.admin.KafkaAdminClient)delegate;
}
public io.vertx.kafka.admin.KafkaAdminClient getDelegate() {
return delegate;
}
/**
* Create a new KafkaAdminClient instance
* @param vertx Vert.x instance to use
* @param config Kafka admin client configuration
* @return an instance of the KafkaAdminClient
*/
public static io.vertx.rxjava3.kafka.admin.KafkaAdminClient create(io.vertx.rxjava3.core.Vertx vertx, java.util.Map config) {
io.vertx.rxjava3.kafka.admin.KafkaAdminClient ret = io.vertx.rxjava3.kafka.admin.KafkaAdminClient.newInstance((io.vertx.kafka.admin.KafkaAdminClient)io.vertx.kafka.admin.KafkaAdminClient.create(vertx.getDelegate(), config));
return ret;
}
/**
* List the topics available in the cluster with the default options.
* @return
*/
public io.reactivex.rxjava3.core.Single> listTopics() {
io.reactivex.rxjava3.core.Single> ret = rxListTopics();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* List the topics available in the cluster with the default options.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxListTopics() {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.listTopics(completionHandler);
});
}
/**
* Describe some topics in the cluster, with the default options.
* @param topicNames the names of the topics to describe
* @return
*/
public io.reactivex.rxjava3.core.Single> describeTopics(java.util.List topicNames) {
io.reactivex.rxjava3.core.Single> ret = rxDescribeTopics(topicNames);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Describe some topics in the cluster, with the default options.
* @param topicNames the names of the topics to describe
* @return
*/
public io.reactivex.rxjava3.core.Single> rxDescribeTopics(java.util.List topicNames) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeTopics(topicNames, completionHandler);
});
}
/**
* Like {@link io.vertx.rxjava3.kafka.admin.KafkaAdminClient#describeTopics} but allows for customised otions
* @param topicNames
* @param options
* @return
*/
public io.reactivex.rxjava3.core.Single> describeTopics(java.util.List topicNames, io.vertx.kafka.admin.DescribeTopicsOptions options) {
io.reactivex.rxjava3.core.Single> ret = rxDescribeTopics(topicNames, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.kafka.admin.KafkaAdminClient#describeTopics} but allows for customised otions
* @param topicNames
* @param options
* @return
*/
public io.reactivex.rxjava3.core.Single> rxDescribeTopics(java.util.List topicNames, io.vertx.kafka.admin.DescribeTopicsOptions options) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeTopics(topicNames, options, completionHandler);
});
}
/**
* Creates a batch of new Kafka topics
* @param topics topics to create
* @return
*/
public io.reactivex.rxjava3.core.Completable createTopics(java.util.List topics) {
io.reactivex.rxjava3.core.Completable ret = rxCreateTopics(topics);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates a batch of new Kafka topics
* @param topics topics to create
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreateTopics(java.util.List topics) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.createTopics(topics, completionHandler);
});
}
/**
* Deletes a batch of Kafka topics
* @param topicNames the names of the topics to delete
* @return
*/
public io.reactivex.rxjava3.core.Completable deleteTopics(java.util.List topicNames) {
io.reactivex.rxjava3.core.Completable ret = rxDeleteTopics(topicNames);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Deletes a batch of Kafka topics
* @param topicNames the names of the topics to delete
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDeleteTopics(java.util.List topicNames) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.deleteTopics(topicNames, completionHandler);
});
}
/**
* Creates a batch of new partitions in the Kafka topic
* @param partitions partitions to create
* @return
*/
public io.reactivex.rxjava3.core.Completable createPartitions(java.util.Map partitions) {
io.reactivex.rxjava3.core.Completable ret = rxCreatePartitions(partitions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Creates a batch of new partitions in the Kafka topic
* @param partitions partitions to create
* @return
*/
public io.reactivex.rxjava3.core.Completable rxCreatePartitions(java.util.Map partitions) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.createPartitions(partitions, completionHandler);
});
}
/**
* Get the the consumer groups available in the cluster with the default options
* @return
*/
public io.reactivex.rxjava3.core.Single> listConsumerGroups() {
io.reactivex.rxjava3.core.Single> ret = rxListConsumerGroups();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get the the consumer groups available in the cluster with the default options
* @return
*/
public io.reactivex.rxjava3.core.Single> rxListConsumerGroups() {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.listConsumerGroups(completionHandler);
});
}
/**
* Describe some group ids in the cluster, with the default options
* @param groupIds the ids of the groups to describe
* @return
*/
public io.reactivex.rxjava3.core.Single> describeConsumerGroups(java.util.List groupIds) {
io.reactivex.rxjava3.core.Single> ret = rxDescribeConsumerGroups(groupIds);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Describe some group ids in the cluster, with the default options
* @param groupIds the ids of the groups to describe
* @return
*/
public io.reactivex.rxjava3.core.Single> rxDescribeConsumerGroups(java.util.List groupIds) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeConsumerGroups(groupIds, completionHandler);
});
}
/**
* Like {@link io.vertx.rxjava3.kafka.admin.KafkaAdminClient#describeConsumerGroups} but allows customized options
* @param groupIds
* @param options
* @return
*/
public io.reactivex.rxjava3.core.Single> describeConsumerGroups(java.util.List groupIds, io.vertx.kafka.admin.DescribeConsumerGroupsOptions options) {
io.reactivex.rxjava3.core.Single> ret = rxDescribeConsumerGroups(groupIds, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.kafka.admin.KafkaAdminClient#describeConsumerGroups} but allows customized options
* @param groupIds
* @param options
* @return
*/
public io.reactivex.rxjava3.core.Single> rxDescribeConsumerGroups(java.util.List groupIds, io.vertx.kafka.admin.DescribeConsumerGroupsOptions options) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeConsumerGroups(groupIds, options, completionHandler);
});
}
/**
* Describe the nodes in the cluster with the default options
* @return
*/
public io.reactivex.rxjava3.core.Single describeCluster() {
io.reactivex.rxjava3.core.Single ret = rxDescribeCluster();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Describe the nodes in the cluster with the default options
* @return
*/
public io.reactivex.rxjava3.core.Single rxDescribeCluster() {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeCluster(completionHandler);
});
}
/**
* Like {@link io.vertx.rxjava3.kafka.admin.KafkaAdminClient#describeCluster} but allows customized options.
* @param options
* @return
*/
public io.reactivex.rxjava3.core.Single describeCluster(io.vertx.kafka.admin.DescribeClusterOptions options) {
io.reactivex.rxjava3.core.Single ret = rxDescribeCluster(options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.kafka.admin.KafkaAdminClient#describeCluster} but allows customized options.
* @param options
* @return
*/
public io.reactivex.rxjava3.core.Single rxDescribeCluster(io.vertx.kafka.admin.DescribeClusterOptions options) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeCluster(options, completionHandler);
});
}
/**
* Delete consumer groups from the cluster.
* @param groupIds the ids of the groups to delete
* @return
*/
public io.reactivex.rxjava3.core.Completable deleteConsumerGroups(java.util.List groupIds) {
io.reactivex.rxjava3.core.Completable ret = rxDeleteConsumerGroups(groupIds);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Delete consumer groups from the cluster.
* @param groupIds the ids of the groups to delete
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDeleteConsumerGroups(java.util.List groupIds) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.deleteConsumerGroups(groupIds, completionHandler);
});
}
/**
* Delete committed offsets for a set of partitions in a consumer group. This will
* succeed at the partition level only if the group is not actively subscribed
* to the corresponding topic.
* @param groupId The group id of the group whose offsets will be deleted
* @param partitions The set of partitions in the consumer group whose offsets will be deleted
* @return
*/
public io.reactivex.rxjava3.core.Completable deleteConsumerGroupOffsets(java.lang.String groupId, java.util.Set partitions) {
io.reactivex.rxjava3.core.Completable ret = rxDeleteConsumerGroupOffsets(groupId, partitions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Delete committed offsets for a set of partitions in a consumer group. This will
* succeed at the partition level only if the group is not actively subscribed
* to the corresponding topic.
* @param groupId The group id of the group whose offsets will be deleted
* @param partitions The set of partitions in the consumer group whose offsets will be deleted
* @return
*/
public io.reactivex.rxjava3.core.Completable rxDeleteConsumerGroupOffsets(java.lang.String groupId, java.util.Set partitions) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.deleteConsumerGroupOffsets(groupId, partitions, completionHandler);
});
}
/**
* Close the admin client
* @return
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Close the admin client
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.close(handler);
});
}
/**
* Close the admin client
* @param timeout timeout to wait for closing
* @return
*/
public io.reactivex.rxjava3.core.Completable close(long timeout) {
io.reactivex.rxjava3.core.Completable ret = rxClose(timeout);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Close the admin client
* @param timeout timeout to wait for closing
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose(long timeout) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.close(timeout, handler);
});
}
/**
* Describe the ACL rules.
* @param aclBindingFilter The filter to use.
* @return
*/
public io.reactivex.rxjava3.core.Single> describeAcls(org.apache.kafka.common.acl.AclBindingFilter aclBindingFilter) {
io.reactivex.rxjava3.core.Single> ret = rxDescribeAcls(aclBindingFilter);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Describe the ACL rules.
* @param aclBindingFilter The filter to use.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxDescribeAcls(org.apache.kafka.common.acl.AclBindingFilter aclBindingFilter) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.describeAcls(aclBindingFilter, completionHandler);
});
}
/**
* Create the ACL rules.
* @param aclBindings The ACL to create.
* @return
*/
public io.reactivex.rxjava3.core.Single> createAcls(java.util.List aclBindings) {
io.reactivex.rxjava3.core.Single> ret = rxCreateAcls(aclBindings);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create the ACL rules.
* @param aclBindings The ACL to create.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxCreateAcls(java.util.List aclBindings) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.createAcls(aclBindings, completionHandler);
});
}
/**
* Delete the ACL rules.
* @param aclBindings The filter to delete matching ACLs.
* @return
*/
public io.reactivex.rxjava3.core.Single> deleteAcls(java.util.List aclBindings) {
io.reactivex.rxjava3.core.Single> ret = rxDeleteAcls(aclBindings);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Delete the ACL rules.
* @param aclBindings The filter to delete matching ACLs.
* @return
*/
public io.reactivex.rxjava3.core.Single> rxDeleteAcls(java.util.List aclBindings) {
return AsyncResultSingle.toSingle( completionHandler -> {
delegate.deleteAcls(aclBindings, completionHandler);
});
}
public static KafkaAdminClient newInstance(io.vertx.kafka.admin.KafkaAdminClient arg) {
return arg != null ? new KafkaAdminClient(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy