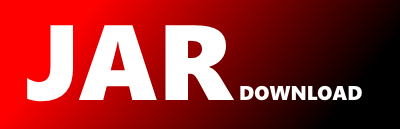
io.vertx.rxjava3.mssqlclient.MSSQLConnection Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.mssqlclient;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A connection to Microsoft SQL Server.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.mssqlclient.MSSQLConnection original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.mssqlclient.MSSQLConnection.class)
public class MSSQLConnection extends io.vertx.rxjava3.sqlclient.SqlConnection {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
MSSQLConnection that = (MSSQLConnection) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new MSSQLConnection((io.vertx.mssqlclient.MSSQLConnection) obj),
MSSQLConnection::getDelegate
);
private final io.vertx.mssqlclient.MSSQLConnection delegate;
public MSSQLConnection(io.vertx.mssqlclient.MSSQLConnection delegate) {
super(delegate);
this.delegate = delegate;
}
public MSSQLConnection(Object delegate) {
super((io.vertx.mssqlclient.MSSQLConnection)delegate);
this.delegate = (io.vertx.mssqlclient.MSSQLConnection)delegate;
}
public io.vertx.mssqlclient.MSSQLConnection getDelegate() {
return delegate;
}
/**
* Create a connection to SQL Server with the given connectOptions
.
* @param vertx the vertx instance
* @param connectOptions the options for the connection
* @return
*/
public static io.reactivex.rxjava3.core.Single connect(io.vertx.rxjava3.core.Vertx vertx, io.vertx.mssqlclient.MSSQLConnectOptions connectOptions) {
io.reactivex.rxjava3.core.Single ret = rxConnect(vertx, connectOptions);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create a connection to SQL Server with the given connectOptions
.
* @param vertx the vertx instance
* @param connectOptions the options for the connection
* @return
*/
public static io.reactivex.rxjava3.core.Single rxConnect(io.vertx.rxjava3.core.Vertx vertx, io.vertx.mssqlclient.MSSQLConnectOptions connectOptions) {
return AsyncResultSingle.toSingle( handler -> {
io.vertx.mssqlclient.MSSQLConnection.connect(vertx.getDelegate(), connectOptions, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.mssqlclient.MSSQLConnection.newInstance((io.vertx.mssqlclient.MSSQLConnection)event))));
});
}
/**
* Like {@link io.vertx.rxjava3.mssqlclient.MSSQLConnection#connect} with options built from connectionUri
.
* @param vertx
* @param connectionUri
* @return
*/
public static io.reactivex.rxjava3.core.Single connect(io.vertx.rxjava3.core.Vertx vertx, java.lang.String connectionUri) {
io.reactivex.rxjava3.core.Single ret = rxConnect(vertx, connectionUri);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.mssqlclient.MSSQLConnection#connect} with options built from connectionUri
.
* @param vertx
* @param connectionUri
* @return
*/
public static io.reactivex.rxjava3.core.Single rxConnect(io.vertx.rxjava3.core.Vertx vertx, java.lang.String connectionUri) {
return AsyncResultSingle.toSingle( handler -> {
io.vertx.mssqlclient.MSSQLConnection.connect(vertx.getDelegate(), connectionUri, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.mssqlclient.MSSQLConnection.newInstance((io.vertx.mssqlclient.MSSQLConnection)event))));
});
}
/**
*
* @param s
* @return
*/
public io.reactivex.rxjava3.core.Single prepare(java.lang.String s) {
io.reactivex.rxjava3.core.Single ret = rxPrepare(s);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
*
* @param s
* @return
*/
public io.reactivex.rxjava3.core.Single rxPrepare(java.lang.String s) {
return AsyncResultSingle.toSingle( handler -> {
delegate.prepare(s, new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.sqlclient.PreparedStatement.newInstance((io.vertx.sqlclient.PreparedStatement)event))));
});
}
/**
*
* @param handler
* @return
*/
public io.vertx.rxjava3.mssqlclient.MSSQLConnection exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
/**
*
* @param handler
* @return
*/
public io.vertx.rxjava3.mssqlclient.MSSQLConnection closeHandler(io.vertx.core.Handler handler) {
delegate.closeHandler(handler);
return this;
}
/**
* Set a handler called when the connection receives an informational message from the server.
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
public io.vertx.rxjava3.mssqlclient.MSSQLConnection infoHandler(io.vertx.core.Handler handler) {
delegate.infoHandler(handler);
return this;
}
/**
* Cast a to {@link io.vertx.rxjava3.mssqlclient.MSSQLConnection}.
*
* This is mostly useful for Vert.x generated APIs like RxJava/Mutiny.
* @param sqlConnection the connection to cast
* @return a {@link io.vertx.rxjava3.mssqlclient.MSSQLConnection instance}
*/
public static io.vertx.rxjava3.mssqlclient.MSSQLConnection cast(io.vertx.rxjava3.sqlclient.SqlConnection sqlConnection) {
io.vertx.rxjava3.mssqlclient.MSSQLConnection ret = io.vertx.rxjava3.mssqlclient.MSSQLConnection.newInstance((io.vertx.mssqlclient.MSSQLConnection)io.vertx.mssqlclient.MSSQLConnection.cast(sqlConnection.getDelegate()));
return ret;
}
public static MSSQLConnection newInstance(io.vertx.mssqlclient.MSSQLConnection arg) {
return arg != null ? new MSSQLConnection(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy