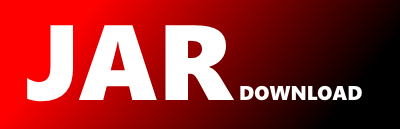
io.vertx.rxjava3.openapi.validation.RequestValidator Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.openapi.validation;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* The {@link io.vertx.rxjava3.openapi.validation.RequestValidator} requires the {@link io.vertx.rxjava3.openapi.validation.ValidatableRequest parameters} in a specific format to be able to
* parse and validate them. This is especially true for exploded parameters. The following table shows how the
* value of a parameter of a request must be stored in a {@link io.vertx.rxjava3.openapi.validation.ValidatableRequest} object. For these examples the key
* of those values is always color.
*
* These are the initial values for each type:
*
* - primitive (string) -> "blue"
* - array -> ["blue","black","brown"]
* - object -> { "R": 100, "G": 200, "B": 150 }
*
* For cookie parameters {@link io.vertx.rxjava3.openapi.validation.ValidatedRequest#getCookies}
*
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
* | style | explode | empty | primitive | array | object |
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
* | form | false | | blue | blue,black,brown | R,100,G,200,B,150 |
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
* | form | true | | blue | color=blue&color=black&color=brown | R=100&G=200&B=150 |
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
*
* For header parameters {@link io.vertx.rxjava3.openapi.validation.ValidatedRequest#getHeaders}
*
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
* | style | explode | empty | primitive | array | object |
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
* | simple | false | | blue | blue,black,brown | R,100,G,200,B,150 |
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
* | simple | true | | blue | blue,black,brown | R=100,G=200,B=150 |
* +--------+---------+-------+-----------+------------------------------------+-------------------------+
*
* For path parameters {@link io.vertx.rxjava3.openapi.validation.ValidatedRequest#getPathParameters}
*
* +--------+---------+--------+-------------+-------------------------------------+--------------------------+
* | style | explode | empty | primitive | array | object |
* +--------+---------+--------+-------------+-------------------------------------+--------------------------+
* | simple | false | | blue | blue,black,brown | R,100,G,200,B,150 |
* +--------+---------+--------+-------------+-------------------------------------+--------------------------+
* | simple | true | | blue | blue,black,brown | R=100,G=200,B=150 |
* +--------+---------+--------+------ ------+-------------------------------------+--------------------------+
* | label | false | . | .blue | .blue,black,brown | .R,100,G,200,B,150 |
* +--------+---------+--------+-------------+-------------------------------------+--------------------------+
* | label | true | . | .blue | .blue.black.brown | .R=100.G=200.B=150 |
* +--------+---------+--------+-------------+-------------------------------------+--------------------------+
* | matrix | false | ;color | ;color=blue | ;color=blue,black,brown | ;color=R,100,G,200,B,150 |
* +--------+---------+-------+--------+-------------------------------------------+--------------------------+
* | matrix | true | ;color | ;color=blue | ;color=blue;color=black;color=brown | ;R=100;G=200;B=150 |
* +--------+---------+--------+-------------+-------------------------------------+--------------------------+
*
* For query parameters {@link io.vertx.rxjava3.openapi.validation.ValidatedRequest#getQuery}
*
* +----------------+---------+-------+------------+-----------------------------------+--------------------------+
* | style | explode | empty | primitive | array | object |
* +----------------+---------+-------+----------+-------------------------------------+--------------------------+
* | form | false | | blue | blue,black,brown | R,100,G,200,B,150 |
* +----------------+---------+-------+-----------+------------------------------------+--------------------------+
* | form | true | | blue | color=blue&color=black&color=brown | R=100&G=200&B=150 |
* +----------------+---------+-------+------ ----+------------------------------------+--------------------------+
* | spaceDelimited | false | not yet supported /
* +----------------+---------+-------+------ ----+------------------------------------+--------------------------+
* | spaceDelimited | true | not yet supported /
* +----------------+---------+-------+------ ----+------------------------------------+--------------------------+
* | pipeDelimited | false | not yet supported /
* +----------------+---------+-------+------ ----+------------------------------------+--------------------------+
* | pipeDelimited | true | not yet supported /
* +----------------+---------+-------+------ ----+------------------------------------+--------------------------+
* | spaceDelimited | true | not yet supported /
* +----------------+---------+-------+------ ----+------------------------------------+--------------------------+
*
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.openapi.validation.RequestValidator original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.openapi.validation.RequestValidator.class)
public class RequestValidator {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RequestValidator that = (RequestValidator) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new RequestValidator((io.vertx.openapi.validation.RequestValidator) obj),
RequestValidator::getDelegate
);
private final io.vertx.openapi.validation.RequestValidator delegate;
public RequestValidator(io.vertx.openapi.validation.RequestValidator delegate) {
this.delegate = delegate;
}
public RequestValidator(Object delegate) {
this.delegate = (io.vertx.openapi.validation.RequestValidator)delegate;
}
public io.vertx.openapi.validation.RequestValidator getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.openapi.validation.ValidatedRequest.newInstance((io.vertx.openapi.validation.ValidatedRequest)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_1 = new TypeArg(o1 -> io.vertx.rxjava3.openapi.validation.ValidatedRequest.newInstance((io.vertx.openapi.validation.ValidatedRequest)o1), o1 -> o1.getDelegate());
private static final TypeArg TYPE_ARG_2 = new TypeArg(o1 -> io.vertx.rxjava3.openapi.validation.ValidatedRequest.newInstance((io.vertx.openapi.validation.ValidatedRequest)o1), o1 -> o1.getDelegate());
/**
* Create a new {@link io.vertx.rxjava3.openapi.validation.RequestValidator}.
* @param vertx the related Vert.x instance
* @param contract the related
* @return an instance of {@link io.vertx.rxjava3.openapi.validation.RequestValidator}.
*/
public static io.vertx.rxjava3.openapi.validation.RequestValidator create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.rxjava3.openapi.contract.OpenAPIContract contract) {
io.vertx.rxjava3.openapi.validation.RequestValidator ret = io.vertx.rxjava3.openapi.validation.RequestValidator.newInstance((io.vertx.openapi.validation.RequestValidator)io.vertx.openapi.validation.RequestValidator.create(vertx.getDelegate(), contract.getDelegate()));
return ret;
}
/**
* Like {@link io.vertx.rxjava3.openapi.validation.RequestValidator#validate}, but the operationId and {@link io.vertx.rxjava3.openapi.validation.ValidatableRequest} are
* determined from the passed request.
*
* Note: Determining the operationId is expensive. If possible use {@link io.vertx.rxjava3.openapi.validation.RequestValidator#validate}.
* @param request the request to validate
* @return A succeeded Future with the parsed and validated request parameters, or a failed Future containing ValidationException.
*/
public io.reactivex.rxjava3.core.Single validate(io.vertx.rxjava3.core.http.HttpServerRequest request) {
io.reactivex.rxjava3.core.Single ret = rxValidate(request);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.openapi.validation.RequestValidator#validate}, but the operationId and {@link io.vertx.rxjava3.openapi.validation.ValidatableRequest} are
* determined from the passed request.
*
* Note: Determining the operationId is expensive. If possible use {@link io.vertx.rxjava3.openapi.validation.RequestValidator#validate}.
* @param request the request to validate
* @return A succeeded Future with the parsed and validated request parameters, or a failed Future containing ValidationException.
*/
public io.reactivex.rxjava3.core.Single rxValidate(io.vertx.rxjava3.core.http.HttpServerRequest request) {
return AsyncResultSingle.toSingle(delegate.validate(request.getDelegate()), __value -> io.vertx.rxjava3.openapi.validation.ValidatedRequest.newInstance((io.vertx.openapi.validation.ValidatedRequest)__value));
}
/**
* Like {@link io.vertx.rxjava3.openapi.validation.RequestValidator#validate}, but {@link io.vertx.rxjava3.openapi.validation.ValidatableRequest} are directly extracted from the passed request.
* @param request the request to validate
* @param operationId the id of the related operation.
* @return A succeeded Future with the parsed and validated request parameters, or a failed Future containing ValidationException.
*/
public io.reactivex.rxjava3.core.Single validate(io.vertx.rxjava3.core.http.HttpServerRequest request, java.lang.String operationId) {
io.reactivex.rxjava3.core.Single ret = rxValidate(request, operationId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.openapi.validation.RequestValidator#validate}, but {@link io.vertx.rxjava3.openapi.validation.ValidatableRequest} are directly extracted from the passed request.
* @param request the request to validate
* @param operationId the id of the related operation.
* @return A succeeded Future with the parsed and validated request parameters, or a failed Future containing ValidationException.
*/
public io.reactivex.rxjava3.core.Single rxValidate(io.vertx.rxjava3.core.http.HttpServerRequest request, java.lang.String operationId) {
return AsyncResultSingle.toSingle(delegate.validate(request.getDelegate(), operationId), __value -> io.vertx.rxjava3.openapi.validation.ValidatedRequest.newInstance((io.vertx.openapi.validation.ValidatedRequest)__value));
}
/**
* Validates the passed request parameters against the operation defined in the related OpenAPI contract.
* @param params the request parameters to validate.
* @param operationId the id of the related operation.
* @return A succeeded Future with the parsed and validated request parameters, or a failed Future containing ValidationException.
*/
public io.reactivex.rxjava3.core.Single validate(io.vertx.rxjava3.openapi.validation.ValidatableRequest params, java.lang.String operationId) {
io.reactivex.rxjava3.core.Single ret = rxValidate(params, operationId);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Validates the passed request parameters against the operation defined in the related OpenAPI contract.
* @param params the request parameters to validate.
* @param operationId the id of the related operation.
* @return A succeeded Future with the parsed and validated request parameters, or a failed Future containing ValidationException.
*/
public io.reactivex.rxjava3.core.Single rxValidate(io.vertx.rxjava3.openapi.validation.ValidatableRequest params, java.lang.String operationId) {
return AsyncResultSingle.toSingle(delegate.validate(params.getDelegate(), operationId), __value -> io.vertx.rxjava3.openapi.validation.ValidatedRequest.newInstance((io.vertx.openapi.validation.ValidatedRequest)__value));
}
public static RequestValidator newInstance(io.vertx.openapi.validation.RequestValidator arg) {
return arg != null ? new RequestValidator(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy