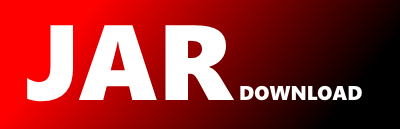
io.vertx.rxjava3.rabbitmq.RabbitMQClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.rabbitmq;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.rabbitmq.RabbitMQClient original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.rabbitmq.RabbitMQClient.class)
public class RabbitMQClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RabbitMQClient that = (RabbitMQClient) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new RabbitMQClient((io.vertx.rabbitmq.RabbitMQClient) obj),
RabbitMQClient::getDelegate
);
private final io.vertx.rabbitmq.RabbitMQClient delegate;
public RabbitMQClient(io.vertx.rabbitmq.RabbitMQClient delegate) {
this.delegate = delegate;
}
public RabbitMQClient(Object delegate) {
this.delegate = (io.vertx.rabbitmq.RabbitMQClient)delegate;
}
public io.vertx.rabbitmq.RabbitMQClient getDelegate() {
return delegate;
}
/**
* Create and return a client configured with the default options.
* @param vertx the vertx instance
* @return the client
*/
public static io.vertx.rxjava3.rabbitmq.RabbitMQClient create(io.vertx.rxjava3.core.Vertx vertx) {
io.vertx.rxjava3.rabbitmq.RabbitMQClient ret = io.vertx.rxjava3.rabbitmq.RabbitMQClient.newInstance((io.vertx.rabbitmq.RabbitMQClient)io.vertx.rabbitmq.RabbitMQClient.create(vertx.getDelegate()));
return ret;
}
/**
* Create and return a client.
* @param vertx the vertx instance
* @param config the client config
* @return the client
*/
public static io.vertx.rxjava3.rabbitmq.RabbitMQClient create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.rabbitmq.RabbitMQOptions config) {
io.vertx.rxjava3.rabbitmq.RabbitMQClient ret = io.vertx.rxjava3.rabbitmq.RabbitMQClient.newInstance((io.vertx.rabbitmq.RabbitMQClient)io.vertx.rabbitmq.RabbitMQClient.create(vertx.getDelegate(), config));
return ret;
}
/**
* Acknowledge one or several received messages. Supply the deliveryTag from the AMQP.Basic.GetOk or AMQP.Basic.Deliver
* method containing the received message being acknowledged.
* @param deliveryTag
* @param multiple
* @return
*/
public io.reactivex.rxjava3.core.Completable basicAck(long deliveryTag, boolean multiple) {
io.reactivex.rxjava3.core.Completable ret = rxBasicAck(deliveryTag, multiple);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Acknowledge one or several received messages. Supply the deliveryTag from the AMQP.Basic.GetOk or AMQP.Basic.Deliver
* method containing the received message being acknowledged.
* @param deliveryTag
* @param multiple
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicAck(long deliveryTag, boolean multiple) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicAck(deliveryTag, multiple, resultHandler);
});
}
/**
* Reject one or several received messages.
* @param deliveryTag
* @param multiple
* @param requeue
* @return
*/
public io.reactivex.rxjava3.core.Completable basicNack(long deliveryTag, boolean multiple, boolean requeue) {
io.reactivex.rxjava3.core.Completable ret = rxBasicNack(deliveryTag, multiple, requeue);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Reject one or several received messages.
* @param deliveryTag
* @param multiple
* @param requeue
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicNack(long deliveryTag, boolean multiple, boolean requeue) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicNack(deliveryTag, multiple, requeue, resultHandler);
});
}
/**
* Retrieve a message from a queue using AMQP.Basic.Get
* @param queue
* @param autoAck
* @return
*/
public io.reactivex.rxjava3.core.Single basicGet(java.lang.String queue, boolean autoAck) {
io.reactivex.rxjava3.core.Single ret = rxBasicGet(queue, autoAck);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Retrieve a message from a queue using AMQP.Basic.Get
* @param queue
* @param autoAck
* @return
*/
public io.reactivex.rxjava3.core.Single rxBasicGet(java.lang.String queue, boolean autoAck) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.basicGet(queue, autoAck, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.rabbitmq.RabbitMQMessage.newInstance((io.vertx.rabbitmq.RabbitMQMessage)event))));
});
}
/**
* @param queue
* @return
*/
public io.reactivex.rxjava3.core.Single basicConsumer(java.lang.String queue) {
io.reactivex.rxjava3.core.Single ret = rxBasicConsumer(queue);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* @param queue
* @return
*/
public io.reactivex.rxjava3.core.Single rxBasicConsumer(java.lang.String queue) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.basicConsumer(queue, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.rabbitmq.RabbitMQConsumer.newInstance((io.vertx.rabbitmq.RabbitMQConsumer)event))));
});
}
/**
* Create a consumer with the given options
.
* @param queue the name of a queue
* @param options options for queue
* @return
*/
public io.reactivex.rxjava3.core.Single basicConsumer(java.lang.String queue, io.vertx.rabbitmq.QueueOptions options) {
io.reactivex.rxjava3.core.Single ret = rxBasicConsumer(queue, options);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Create a consumer with the given options
.
* @param queue the name of a queue
* @param options options for queue
* @return
*/
public io.reactivex.rxjava3.core.Single rxBasicConsumer(java.lang.String queue, io.vertx.rabbitmq.QueueOptions options) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.basicConsumer(queue, options, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.rabbitmq.RabbitMQConsumer.newInstance((io.vertx.rabbitmq.RabbitMQConsumer)event))));
});
}
/**
* Publish a message. Publishing to a non-existent exchange will result in a channel-level protocol exception,
* which closes the channel. Invocations of Channel#basicPublish will eventually block if a resource-driven alarm is in effect.
* @param exchange
* @param routingKey
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Completable basicPublish(java.lang.String exchange, java.lang.String routingKey, io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Completable ret = rxBasicPublish(exchange, routingKey, body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Publish a message. Publishing to a non-existent exchange will result in a channel-level protocol exception,
* which closes the channel. Invocations of Channel#basicPublish will eventually block if a resource-driven alarm is in effect.
* @param exchange
* @param routingKey
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicPublish(java.lang.String exchange, java.lang.String routingKey, io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicPublish(exchange, routingKey, body.getDelegate(), resultHandler);
});
}
/**
* Add a Confirm Listener to the channel.
* Note that this will automatically call confirmSelect, it is not necessary to call that too.
* @param maxQueueSize maximum size of the queue of confirmations
* @return
*/
public io.reactivex.rxjava3.core.Single> addConfirmListener(int maxQueueSize) {
io.reactivex.rxjava3.core.Single> ret = rxAddConfirmListener(maxQueueSize);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Add a Confirm Listener to the channel.
* Note that this will automatically call confirmSelect, it is not necessary to call that too.
* @param maxQueueSize maximum size of the queue of confirmations
* @return
*/
public io.reactivex.rxjava3.core.Single> rxAddConfirmListener(int maxQueueSize) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.addConfirmListener(maxQueueSize, new io.vertx.lang.rx.DelegatingHandler<>(resultHandler, ar -> ar.map(event -> io.vertx.rxjava3.core.streams.ReadStream.newInstance((io.vertx.core.streams.ReadStream)event, TypeArg.unknown()))));
});
}
/**
* Enables publisher acknowledgements on this channel. Can be called once during client initialisation. Calls to basicPublish()
* will have to be confirmed.
* @return
*/
public io.reactivex.rxjava3.core.Completable confirmSelect() {
io.reactivex.rxjava3.core.Completable ret = rxConfirmSelect();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Enables publisher acknowledgements on this channel. Can be called once during client initialisation. Calls to basicPublish()
* will have to be confirmed.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxConfirmSelect() {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.confirmSelect(resultHandler);
});
}
/**
* Wait until all messages published since the last call have been either ack'd or nack'd by the broker.
* This will incur slight performance loss at the expense of higher write consistency.
* If desired, multiple calls to basicPublish() can be batched before confirming.
* @return
*/
public io.reactivex.rxjava3.core.Completable waitForConfirms() {
io.reactivex.rxjava3.core.Completable ret = rxWaitForConfirms();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Wait until all messages published since the last call have been either ack'd or nack'd by the broker.
* This will incur slight performance loss at the expense of higher write consistency.
* If desired, multiple calls to basicPublish() can be batched before confirming.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWaitForConfirms() {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.waitForConfirms(resultHandler);
});
}
/**
* Wait until all messages published since the last call have been either ack'd or nack'd by the broker; or until timeout elapses. If the timeout expires a TimeoutException is thrown.
* @param timeout
* @return
*/
public io.reactivex.rxjava3.core.Completable waitForConfirms(long timeout) {
io.reactivex.rxjava3.core.Completable ret = rxWaitForConfirms(timeout);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Wait until all messages published since the last call have been either ack'd or nack'd by the broker; or until timeout elapses. If the timeout expires a TimeoutException is thrown.
* @param timeout
* @return
*/
public io.reactivex.rxjava3.core.Completable rxWaitForConfirms(long timeout) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.waitForConfirms(timeout, resultHandler);
});
}
/**
* Request a specific prefetchCount "quality of service" settings
* for this channel.
* @param prefetchCount maximum number of messages that the server will deliver, 0 if unlimited
* @return
*/
public io.reactivex.rxjava3.core.Completable basicQos(int prefetchCount) {
io.reactivex.rxjava3.core.Completable ret = rxBasicQos(prefetchCount);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Request a specific prefetchCount "quality of service" settings
* for this channel.
* @param prefetchCount maximum number of messages that the server will deliver, 0 if unlimited
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicQos(int prefetchCount) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicQos(prefetchCount, resultHandler);
});
}
/**
* Request a specific prefetchCount "quality of service" settings
* for this channel.
* @param prefetchCount maximum number of messages that the server will deliver, 0 if unlimited
* @param global true if the settings should be applied to the entire channel rather than each consumer
* @return
*/
public io.reactivex.rxjava3.core.Completable basicQos(int prefetchCount, boolean global) {
io.reactivex.rxjava3.core.Completable ret = rxBasicQos(prefetchCount, global);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Request a specific prefetchCount "quality of service" settings
* for this channel.
* @param prefetchCount maximum number of messages that the server will deliver, 0 if unlimited
* @param global true if the settings should be applied to the entire channel rather than each consumer
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicQos(int prefetchCount, boolean global) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicQos(prefetchCount, global, resultHandler);
});
}
/**
* Request specific "quality of service" settings.
*
* These settings impose limits on the amount of data the server
* will deliver to consumers before requiring acknowledgements.
* Thus they provide a means of consumer-initiated flow control.
* @param prefetchSize maximum amount of content (measured in octets) that the server will deliver, 0 if unlimited
* @param prefetchCount maximum number of messages that the server will deliver, 0 if unlimited
* @param global true if the settings should be applied to the entire channel rather than each consumer
* @return
*/
public io.reactivex.rxjava3.core.Completable basicQos(int prefetchSize, int prefetchCount, boolean global) {
io.reactivex.rxjava3.core.Completable ret = rxBasicQos(prefetchSize, prefetchCount, global);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Request specific "quality of service" settings.
*
* These settings impose limits on the amount of data the server
* will deliver to consumers before requiring acknowledgements.
* Thus they provide a means of consumer-initiated flow control.
* @param prefetchSize maximum amount of content (measured in octets) that the server will deliver, 0 if unlimited
* @param prefetchCount maximum number of messages that the server will deliver, 0 if unlimited
* @param global true if the settings should be applied to the entire channel rather than each consumer
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicQos(int prefetchSize, int prefetchCount, boolean global) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicQos(prefetchSize, prefetchCount, global, resultHandler);
});
}
/**
* Declare an exchange.
* @param exchange
* @param type
* @param durable
* @param autoDelete
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeDeclare(java.lang.String exchange, java.lang.String type, boolean durable, boolean autoDelete) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeDeclare(exchange, type, durable, autoDelete);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Declare an exchange.
* @param exchange
* @param type
* @param durable
* @param autoDelete
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeDeclare(java.lang.String exchange, java.lang.String type, boolean durable, boolean autoDelete) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeDeclare(exchange, type, durable, autoDelete, resultHandler);
});
}
/**
* Declare an exchange with additional parameters such as dead lettering, an alternate exchange or TTL.
* @param exchange
* @param type
* @param durable
* @param autoDelete
* @param config
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeDeclare(java.lang.String exchange, java.lang.String type, boolean durable, boolean autoDelete, io.vertx.core.json.JsonObject config) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeDeclare(exchange, type, durable, autoDelete, config);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Declare an exchange with additional parameters such as dead lettering, an alternate exchange or TTL.
* @param exchange
* @param type
* @param durable
* @param autoDelete
* @param config
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeDeclare(java.lang.String exchange, java.lang.String type, boolean durable, boolean autoDelete, io.vertx.core.json.JsonObject config) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeDeclare(exchange, type, durable, autoDelete, config, resultHandler);
});
}
/**
* Delete an exchange, without regard for whether it is in use or not.
* @param exchange
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeDelete(java.lang.String exchange) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeDelete(exchange);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Delete an exchange, without regard for whether it is in use or not.
* @param exchange
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeDelete(java.lang.String exchange) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeDelete(exchange, resultHandler);
});
}
/**
* Bind an exchange to an exchange.
* @param destination
* @param source
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeBind(java.lang.String destination, java.lang.String source, java.lang.String routingKey) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeBind(destination, source, routingKey);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Bind an exchange to an exchange.
* @param destination
* @param source
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeBind(java.lang.String destination, java.lang.String source, java.lang.String routingKey) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeBind(destination, source, routingKey, resultHandler);
});
}
/**
* Bind an exchange to an exchange.
* @param destination
* @param source
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeBind(java.lang.String destination, java.lang.String source, java.lang.String routingKey, java.util.Map arguments) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeBind(destination, source, routingKey, arguments);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Bind an exchange to an exchange.
* @param destination
* @param source
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeBind(java.lang.String destination, java.lang.String source, java.lang.String routingKey, java.util.Map arguments) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeBind(destination, source, routingKey, arguments, resultHandler);
});
}
/**
* Unbind an exchange from an exchange.
* @param destination
* @param source
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeUnbind(java.lang.String destination, java.lang.String source, java.lang.String routingKey) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeUnbind(destination, source, routingKey);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Unbind an exchange from an exchange.
* @param destination
* @param source
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeUnbind(java.lang.String destination, java.lang.String source, java.lang.String routingKey) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeUnbind(destination, source, routingKey, resultHandler);
});
}
/**
* Unbind an exchange from an exchange.
* @param destination
* @param source
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable exchangeUnbind(java.lang.String destination, java.lang.String source, java.lang.String routingKey, java.util.Map arguments) {
io.reactivex.rxjava3.core.Completable ret = rxExchangeUnbind(destination, source, routingKey, arguments);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Unbind an exchange from an exchange.
* @param destination
* @param source
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable rxExchangeUnbind(java.lang.String destination, java.lang.String source, java.lang.String routingKey, java.util.Map arguments) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.exchangeUnbind(destination, source, routingKey, arguments, resultHandler);
});
}
/**
* Actively declare a server-named exclusive, autodelete, non-durable queue.
* @return
*/
public io.reactivex.rxjava3.core.Single queueDeclareAuto() {
io.reactivex.rxjava3.core.Single ret = rxQueueDeclareAuto();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Actively declare a server-named exclusive, autodelete, non-durable queue.
* @return
*/
public io.reactivex.rxjava3.core.Single rxQueueDeclareAuto() {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.queueDeclareAuto(resultHandler);
});
}
/**
* Bind a queue to an exchange
* @param queue
* @param exchange
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable queueBind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey) {
io.reactivex.rxjava3.core.Completable ret = rxQueueBind(queue, exchange, routingKey);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Bind a queue to an exchange
* @param queue
* @param exchange
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable rxQueueBind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.queueBind(queue, exchange, routingKey, resultHandler);
});
}
/**
* Bind a queue to an exchange
* @param queue
* @param exchange
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable queueBind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey, java.util.Map arguments) {
io.reactivex.rxjava3.core.Completable ret = rxQueueBind(queue, exchange, routingKey, arguments);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Bind a queue to an exchange
* @param queue
* @param exchange
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable rxQueueBind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey, java.util.Map arguments) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.queueBind(queue, exchange, routingKey, arguments, resultHandler);
});
}
/**
* Unbind a queue from an exchange
* @param queue
* @param exchange
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable queueUnbind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey) {
io.reactivex.rxjava3.core.Completable ret = rxQueueUnbind(queue, exchange, routingKey);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Unbind a queue from an exchange
* @param queue
* @param exchange
* @param routingKey
* @return
*/
public io.reactivex.rxjava3.core.Completable rxQueueUnbind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.queueUnbind(queue, exchange, routingKey, resultHandler);
});
}
/**
* Unbind a queue from an exchange
* @param queue
* @param exchange
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable queueUnbind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey, java.util.Map arguments) {
io.reactivex.rxjava3.core.Completable ret = rxQueueUnbind(queue, exchange, routingKey, arguments);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Unbind a queue from an exchange
* @param queue
* @param exchange
* @param routingKey
* @param arguments
* @return
*/
public io.reactivex.rxjava3.core.Completable rxQueueUnbind(java.lang.String queue, java.lang.String exchange, java.lang.String routingKey, java.util.Map arguments) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.queueUnbind(queue, exchange, routingKey, arguments, resultHandler);
});
}
/**
* Returns the number of messages in a queue ready to be delivered.
* @param queue
* @return
*/
public io.reactivex.rxjava3.core.Single messageCount(java.lang.String queue) {
io.reactivex.rxjava3.core.Single ret = rxMessageCount(queue);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Returns the number of messages in a queue ready to be delivered.
* @param queue
* @return
*/
public io.reactivex.rxjava3.core.Single rxMessageCount(java.lang.String queue) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.messageCount(queue, resultHandler);
});
}
/**
* Start the rabbitMQ client. Create the connection and the channel.
* @return
*/
public io.reactivex.rxjava3.core.Completable start() {
io.reactivex.rxjava3.core.Completable ret = rxStart();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Start the rabbitMQ client. Create the connection and the channel.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxStart() {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.start(resultHandler);
});
}
/**
* Stop the rabbitMQ client. Close the connection and its channel.
* @return
*/
public io.reactivex.rxjava3.core.Completable stop() {
io.reactivex.rxjava3.core.Completable ret = rxStop();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Stop the rabbitMQ client. Close the connection and its channel.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxStop() {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.stop(resultHandler);
});
}
/**
* Check if a connection is open
* @return true when the connection is open, false otherwise
*/
public boolean isConnected() {
boolean ret = delegate.isConnected();
return ret;
}
/**
* restart the rabbitMQ connect.
* @param attempts number of attempts
* @return
*/
public io.reactivex.rxjava3.core.Completable restartConnect(int attempts) {
io.reactivex.rxjava3.core.Completable ret = rxRestartConnect(attempts);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* restart the rabbitMQ connect.
* @param attempts number of attempts
* @return
*/
public io.reactivex.rxjava3.core.Completable rxRestartConnect(int attempts) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.restartConnect(attempts, resultHandler);
});
}
/**
* Check if a channel is open
* @return true when the connection is open, false otherwise
*/
public boolean isOpenChannel() {
boolean ret = delegate.isOpenChannel();
return ret;
}
/**
* Publish a message. Publishing to a non-existent exchange will result in a channel-level protocol exception,
* which closes the channel. Invocations of Channel#basicPublish will eventually block if a resource-driven alarm is in effect.
* @param exchange
* @param routingKey
* @param properties
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Completable basicPublish(java.lang.String exchange, java.lang.String routingKey, com.rabbitmq.client.BasicProperties properties, io.vertx.rxjava3.core.buffer.Buffer body) {
io.reactivex.rxjava3.core.Completable ret = rxBasicPublish(exchange, routingKey, properties, body);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Publish a message. Publishing to a non-existent exchange will result in a channel-level protocol exception,
* which closes the channel. Invocations of Channel#basicPublish will eventually block if a resource-driven alarm is in effect.
* @param exchange
* @param routingKey
* @param properties
* @param body
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicPublish(java.lang.String exchange, java.lang.String routingKey, com.rabbitmq.client.BasicProperties properties, io.vertx.rxjava3.core.buffer.Buffer body) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicPublish(exchange, routingKey, properties, body.getDelegate(), resultHandler);
});
}
/**
* Publish a message. Publishing to a non-existent exchange will result in a channel-level protocol exception,
* which closes the channel. Invocations of Channel#basicPublish will eventually block if a resource-driven alarm is in effect.
*
* The deliveryTagHandler will be called before the message is sent, which is necessary because the confirmation may arrive
* asynchronously before the resultHandler is called.
* @param exchange
* @param routingKey
* @param properties
* @param body
* @param deliveryTagHandler callback to capture the deliveryTag for this message. Note that this will be called synchronously in the context of the client before the result is known.
* @return
*/
public io.reactivex.rxjava3.core.Completable basicPublishWithDeliveryTag(java.lang.String exchange, java.lang.String routingKey, com.rabbitmq.client.BasicProperties properties, io.vertx.rxjava3.core.buffer.Buffer body, io.vertx.core.Handler deliveryTagHandler) {
io.reactivex.rxjava3.core.Completable ret = rxBasicPublishWithDeliveryTag(exchange, routingKey, properties, body, deliveryTagHandler);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Publish a message. Publishing to a non-existent exchange will result in a channel-level protocol exception,
* which closes the channel. Invocations of Channel#basicPublish will eventually block if a resource-driven alarm is in effect.
*
* The deliveryTagHandler will be called before the message is sent, which is necessary because the confirmation may arrive
* asynchronously before the resultHandler is called.
* @param exchange
* @param routingKey
* @param properties
* @param body
* @param deliveryTagHandler callback to capture the deliveryTag for this message. Note that this will be called synchronously in the context of the client before the result is known.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxBasicPublishWithDeliveryTag(java.lang.String exchange, java.lang.String routingKey, com.rabbitmq.client.BasicProperties properties, io.vertx.rxjava3.core.buffer.Buffer body, io.vertx.core.Handler deliveryTagHandler) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.basicPublishWithDeliveryTag(exchange, routingKey, properties, body.getDelegate(), deliveryTagHandler, resultHandler);
});
}
/**
* Declare a queue
* @param queue
* @param durable
* @param exclusive
* @param autoDelete
* @return
*/
public io.reactivex.rxjava3.core.Single queueDeclare(java.lang.String queue, boolean durable, boolean exclusive, boolean autoDelete) {
io.reactivex.rxjava3.core.Single ret = rxQueueDeclare(queue, durable, exclusive, autoDelete);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Declare a queue
* @param queue
* @param durable
* @param exclusive
* @param autoDelete
* @return
*/
public io.reactivex.rxjava3.core.Single rxQueueDeclare(java.lang.String queue, boolean durable, boolean exclusive, boolean autoDelete) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.queueDeclare(queue, durable, exclusive, autoDelete, resultHandler);
});
}
/**
* Declare a queue with config options
* @param queue
* @param durable
* @param exclusive
* @param autoDelete
* @param config
* @return
*/
public io.reactivex.rxjava3.core.Single queueDeclare(java.lang.String queue, boolean durable, boolean exclusive, boolean autoDelete, io.vertx.core.json.JsonObject config) {
io.reactivex.rxjava3.core.Single ret = rxQueueDeclare(queue, durable, exclusive, autoDelete, config);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Declare a queue with config options
* @param queue
* @param durable
* @param exclusive
* @param autoDelete
* @param config
* @return
*/
public io.reactivex.rxjava3.core.Single rxQueueDeclare(java.lang.String queue, boolean durable, boolean exclusive, boolean autoDelete, io.vertx.core.json.JsonObject config) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.queueDeclare(queue, durable, exclusive, autoDelete, config, resultHandler);
});
}
/**
* Delete a queue, without regard for whether it is in use or has messages on it
* @param queue
* @return
*/
public io.reactivex.rxjava3.core.Single queueDelete(java.lang.String queue) {
io.reactivex.rxjava3.core.Single ret = rxQueueDelete(queue);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Delete a queue, without regard for whether it is in use or has messages on it
* @param queue
* @return
*/
public io.reactivex.rxjava3.core.Single rxQueueDelete(java.lang.String queue) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.queueDelete(queue, resultHandler);
});
}
/**
* Delete a queue
* @param queue
* @param ifUnused
* @param ifEmpty
* @return
*/
public io.reactivex.rxjava3.core.Single queueDeleteIf(java.lang.String queue, boolean ifUnused, boolean ifEmpty) {
io.reactivex.rxjava3.core.Single ret = rxQueueDeleteIf(queue, ifUnused, ifEmpty);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Delete a queue
* @param queue
* @param ifUnused
* @param ifEmpty
* @return
*/
public io.reactivex.rxjava3.core.Single rxQueueDeleteIf(java.lang.String queue, boolean ifUnused, boolean ifEmpty) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.queueDeleteIf(queue, ifUnused, ifEmpty, resultHandler);
});
}
public static RabbitMQClient newInstance(io.vertx.rabbitmq.RabbitMQClient arg) {
return arg != null ? new RabbitMQClient(arg) : null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy