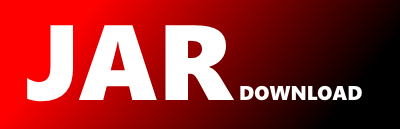
io.vertx.rxjava3.redis.client.RedisConnection Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.redis.client;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A simple Redis client.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.redis.client.RedisConnection original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.redis.client.RedisConnection.class)
public class RedisConnection implements io.vertx.rxjava3.core.streams.ReadStream {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
RedisConnection that = (RedisConnection) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new RedisConnection((io.vertx.redis.client.RedisConnection) obj),
RedisConnection::getDelegate
);
private final io.vertx.redis.client.RedisConnection delegate;
public RedisConnection(io.vertx.redis.client.RedisConnection delegate) {
this.delegate = delegate;
}
public RedisConnection(Object delegate) {
this.delegate = (io.vertx.redis.client.RedisConnection)delegate;
}
public io.vertx.redis.client.RedisConnection getDelegate() {
return delegate;
}
private io.reactivex.rxjava3.core.Observable observable;
private io.reactivex.rxjava3.core.Flowable flowable;
public synchronized io.reactivex.rxjava3.core.Observable toObservable() {
if (observable == null) {
Function conv = io.vertx.rxjava3.redis.client.Response::newInstance;
observable = ObservableHelper.toObservable(delegate, conv);
}
return observable;
}
public synchronized io.reactivex.rxjava3.core.Flowable toFlowable() {
if (flowable == null) {
Function conv = io.vertx.rxjava3.redis.client.Response::newInstance;
flowable = FlowableHelper.toFlowable(delegate, conv);
}
return flowable;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
/**
* Pause this stream and return a to transfer the elements of this stream to a destination .
*
* The stream will be resumed when the pipe will be wired to a WriteStream
.
* @return a pipe
*/
public io.vertx.rxjava3.core.streams.Pipe pipe() {
io.vertx.rxjava3.core.streams.Pipe ret = io.vertx.rxjava3.core.streams.Pipe.newInstance((io.vertx.core.streams.Pipe)delegate.pipe(), TYPE_ARG_0);
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
*
* Once this stream has ended or failed, the write stream will be ended and the handler
will be
* called with the result.
* @param dst the destination write stream
* @return
*/
public io.reactivex.rxjava3.core.Completable pipeTo(io.vertx.rxjava3.core.streams.WriteStream dst) {
io.reactivex.rxjava3.core.Completable ret = rxPipeTo(dst);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Pipe this ReadStream
to the WriteStream
.
*
* Elements emitted by this stream will be written to the write stream until this stream ends or fails.
*
* Once this stream has ended or failed, the write stream will be ended and the handler
will be
* called with the result.
* @param dst the destination write stream
* @return
*/
public io.reactivex.rxjava3.core.Completable rxPipeTo(io.vertx.rxjava3.core.streams.WriteStream dst) {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.pipeTo(dst.getDelegate(), handler);
});
}
/**
*
* @param handler
* @return
*/
public io.vertx.rxjava3.redis.client.RedisConnection exceptionHandler(io.vertx.core.Handler handler) {
delegate.exceptionHandler(handler);
return this;
}
/**
*
* @param handler
* @return
*/
public io.vertx.rxjava3.redis.client.RedisConnection handler(io.vertx.core.Handler handler) {
delegate.handler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)event)));
return this;
}
/**
*
* @return
*/
public io.vertx.rxjava3.redis.client.RedisConnection pause() {
delegate.pause();
return this;
}
/**
*
* @return
*/
public io.vertx.rxjava3.redis.client.RedisConnection resume() {
delegate.resume();
return this;
}
/**
*
* @param amount
* @return
*/
public io.vertx.rxjava3.redis.client.RedisConnection fetch(long amount) {
delegate.fetch(amount);
return this;
}
/**
*
* @param endHandler
* @return
*/
public io.vertx.rxjava3.redis.client.RedisConnection endHandler(io.vertx.core.Handler endHandler) {
delegate.endHandler(endHandler);
return this;
}
/**
* Send the given command to the redis server or cluster.
* @param command the command to send
* @return fluent self.
*/
public io.reactivex.rxjava3.core.Maybe send(io.vertx.rxjava3.redis.client.Request command) {
io.reactivex.rxjava3.core.Maybe ret = rxSend(command);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Send the given command to the redis server or cluster.
* @param command the command to send
* @return fluent self.
*/
public io.reactivex.rxjava3.core.Maybe rxSend(io.vertx.rxjava3.redis.client.Request command) {
return AsyncResultMaybe.toMaybe( onSend -> {
delegate.send(command.getDelegate(), new io.vertx.lang.rx.DelegatingHandler<>(onSend, ar -> ar.map(event -> io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)event))));
});
}
/**
* Sends a list of commands in a single IO operation, this prevents any inter twinning to happen from other
* client users.
* @param commands list of command to send
* @return fluent self.
*/
public io.reactivex.rxjava3.core.Single> batch(java.util.List commands) {
io.reactivex.rxjava3.core.Single> ret = rxBatch(commands);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Sends a list of commands in a single IO operation, this prevents any inter twinning to happen from other
* client users.
* @param commands list of command to send
* @return fluent self.
*/
public io.reactivex.rxjava3.core.Single> rxBatch(java.util.List commands) {
return AsyncResultSingle.toSingle( onSend -> {
delegate.batch(commands.stream().map(elt -> elt.getDelegate()).collect(Collectors.toList()), new io.vertx.lang.rx.DelegatingHandler<>(onSend, ar -> ar.map(event -> event.stream().map(elt -> io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)elt)).collect(Collectors.toList()))));
});
}
/**
* Closes the connection or returns to the pool.
* @return
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Closes the connection or returns to the pool.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( onClose -> {
delegate.close(onClose);
});
}
/**
* Flag to notify if the pending message queue (commands in transit) is full.
*
* When the pending message queue is full and a new send command is issued it will result in a exception to be thrown.
* Checking this flag before sending can allow the application to wait before sending the next message.
* @return true is queue is full.
*/
public boolean pendingQueueFull() {
boolean ret = delegate.pendingQueueFull();
return ret;
}
public static RedisConnection newInstance(io.vertx.redis.client.RedisConnection arg) {
return arg != null ? new RedisConnection(arg) : null;
}
}