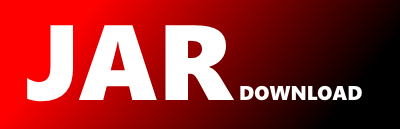
io.vertx.rxjava3.redis.client.Response Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.redis.client;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* The response received from the REDIS server. Redis responses can have several representations:
*
*
* - simple string - C string
* - integer - 64bit integer value
* - bulk - byte array
* - multi - list
*
*
* Due to the dynamic nature the response object will try to cast the received response to the desired type. A special
* case should be noted that multi responses are also handled by the response object as it implements the iterable
* interface. So in this case constructs like for loops on the response will give you access to the underlying elements.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.redis.client.Response original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.redis.client.Response.class)
public class Response implements Iterable {
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Response that = (Response) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
@Override
public Iterator iterator() {
Function conv = io.vertx.rxjava3.redis.client.Response::newInstance;
return new MappingIterator<>(delegate.iterator(), conv);
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new Response((io.vertx.redis.client.Response) obj),
Response::getDelegate
);
private final io.vertx.redis.client.Response delegate;
public Response(io.vertx.redis.client.Response delegate) {
this.delegate = delegate;
}
public Response(Object delegate) {
this.delegate = (io.vertx.redis.client.Response)delegate;
}
public io.vertx.redis.client.Response getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)o1), o1 -> o1.getDelegate());
/**
* The response return type.
* @return the type.
*/
public io.vertx.redis.client.ResponseType type() {
io.vertx.redis.client.ResponseType ret = delegate.type();
return ret;
}
/**
* RESP3 responses may include attributes
* @return the a key value map of attributes to this response.
*/
public java.util.Map attributes() {
java.util.Map ret = delegate.attributes().entrySet().stream().collect(Collectors.toMap(_e -> _e.getKey(), _e -> io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)_e.getValue())));
return ret;
}
/**
* Get this response as a String.
* @return string value
*/
public java.lang.String toString() {
java.lang.String ret = delegate.toString();
return ret;
}
/**
* RESP3 Verbatim Bulk message include a 3 character format.
* @return
*/
public java.lang.String format() {
java.lang.String ret = delegate.format();
return ret;
}
/**
* Get this response as a Double.
* @return double value.
*/
public java.lang.Double toDouble() {
java.lang.Double ret = delegate.toDouble();
return ret;
}
/**
* Get this response as a Float.
* @return double value.
*/
public java.lang.Float toFloat() {
java.lang.Float ret = delegate.toFloat();
return ret;
}
/**
* Get this response as a Long.
* @return long value.
*/
public java.lang.Long toLong() {
java.lang.Long ret = delegate.toLong();
return ret;
}
/**
* Get this response as a Integer.
* @return int value.
*/
public java.lang.Integer toInteger() {
java.lang.Integer ret = delegate.toInteger();
return ret;
}
/**
* Get this response as a Short.
* @return short value.
*/
public java.lang.Short toShort() {
java.lang.Short ret = delegate.toShort();
return ret;
}
/**
* Get this response as a Byte.
* @return byte value.
*/
public java.lang.Byte toByte() {
java.lang.Byte ret = delegate.toByte();
return ret;
}
/**
* Get this response as a Boolean.
* @return boolean value.
*/
public java.lang.Boolean toBoolean() {
java.lang.Boolean ret = delegate.toBoolean();
return ret;
}
/**
* Get this response as Buffer.
* @return buffer value.
*/
public io.vertx.rxjava3.core.buffer.Buffer toBuffer() {
io.vertx.rxjava3.core.buffer.Buffer ret = io.vertx.rxjava3.core.buffer.Buffer.newInstance((io.vertx.core.buffer.Buffer)delegate.toBuffer());
return ret;
}
/**
* Get this multi response value at a numerical index.
* @param index the required index.
* @return Response value.
*/
public io.vertx.rxjava3.redis.client.Response get(int index) {
io.vertx.rxjava3.redis.client.Response ret = io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)delegate.get(index));
return ret;
}
/**
* Get this multi response value at a string key. Note that REDIS does not support strings as keys but by convention
* it encodes hashes in lists where index i is the key, and index i+1 is the value.
* @param key the required key.
* @return Response value.
*/
public io.vertx.rxjava3.redis.client.Response get(java.lang.String key) {
io.vertx.rxjava3.redis.client.Response ret = io.vertx.rxjava3.redis.client.Response.newInstance((io.vertx.redis.client.Response)delegate.get(key));
return ret;
}
/**
* Does this multi response contains a string key. Note that REDIS does not support strings as keys but by convention
* it encodes hashes in lists where index i is the key, and index i+1 is the value.
* @param key the required key.
* @return Response value.
*/
public boolean containsKey(java.lang.String key) {
boolean ret = delegate.containsKey(key);
return ret;
}
/**
* Get this multi response keys from a hash. Note that REDIS does not support strings as keys but by convention
* it encodes hashes in lists where index i is the key, and index i+1 is the value.
* @return the set of keys.
*/
public java.util.Set getKeys() {
java.util.Set ret = delegate.getKeys();
return ret;
}
/**
* Get this size of this multi response.
* @return the size of the multi.
*/
public int size() {
int ret = delegate.size();
return ret;
}
/**
* Returns whether this multi response is an array and hence {@link io.vertx.rxjava3.redis.client.Response#get} can be called.
*
* Returns true
also for and .
* @return whether this multi response is an array
*/
public boolean isArray() {
boolean ret = delegate.isArray();
return ret;
}
/**
* Returns whether this multi response is a map and hence {@link io.vertx.rxjava3.redis.client.Response#get},
* {@link io.vertx.rxjava3.redis.client.Response#containsKey} and {@link io.vertx.rxjava3.redis.client.Response#getKeys} may be called.
*
* Returns true
also for .
* @return whether this multi response is a map
*/
public boolean isMap() {
boolean ret = delegate.isMap();
return ret;
}
/**
* Get this response as a Number. In contrast to other numeric getters, this will not
* perform any conversion if the underlying type is not numeric.
* @return number value
*/
public java.lang.Number toNumber() {
java.lang.Number ret = delegate.toNumber();
return ret;
}
/**
* Get this response as a BigInteger.
* @return long value.
*/
public java.math.BigInteger toBigInteger() {
java.math.BigInteger ret = delegate.toBigInteger();
return ret;
}
/**
* Get this response as a String encoded with the given charset.
* @param encoding
* @return String value.
*/
public java.lang.String toString(java.nio.charset.Charset encoding) {
java.lang.String ret = delegate.toString(encoding);
return ret;
}
/**
* Get this response as a byte[].
* @return byte[] value.
*/
public byte[] toBytes() {
byte[] ret = delegate.toBytes();
return ret;
}
public static Response newInstance(io.vertx.redis.client.Response arg) {
return arg != null ? new Response(arg) : null;
}
}