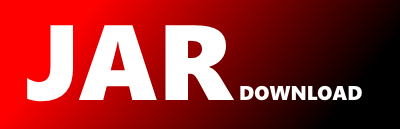
io.vertx.rxjava3.servicediscovery.ServiceDiscovery Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.servicediscovery;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* Service Discovery main entry point.
*
* The service discovery is an infrastructure that let you publish and find `services`. A `service` is a discoverable
* functionality. It can be qualified by its type, metadata, and location. So a `service` can be a database, a
* service proxy, a HTTP endpoint. It does not have to be a vert.x entity, but can be anything. Each service is
* described by a {@link io.vertx.servicediscovery.Record}.
*
* The service discovery implements the interactions defined in the service-oriented computing. And to some extend,
* also provides the dynamic service-oriented computing interaction. So, application can react to arrival and
* departure of services.
*
* A service provider can:
*
* * publish a service record
* * un-publish a published record
* * update the status of a published service (down, out of service...)
*
* A service consumer can:
*
* * lookup for services
* * bind to a selected service (it gets a {@link io.vertx.rxjava3.servicediscovery.ServiceReference}) and use it
* * release the service once the consumer is done with it
* * listen for arrival, departure and modification of services.
*
* Consumer would 1) lookup for service record matching their need, 2) retrieve the {@link io.vertx.rxjava3.servicediscovery.ServiceReference} that give access
* to the service, 3) get a service object to access the service, 4) release the service object once done.
*
* A state above, the central piece of information shared by the providers and consumers are {@link io.vertx.servicediscovery.Record}.
*
* Providers and consumers must create their own {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery} instance. These instances are collaborating
* in background (distributed structure) to keep the set of services in sync.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.servicediscovery.ServiceDiscovery original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.servicediscovery.ServiceDiscovery.class)
public class ServiceDiscovery {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
ServiceDiscovery that = (ServiceDiscovery) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new ServiceDiscovery((io.vertx.servicediscovery.ServiceDiscovery) obj),
ServiceDiscovery::getDelegate
);
private final io.vertx.servicediscovery.ServiceDiscovery delegate;
public ServiceDiscovery(io.vertx.servicediscovery.ServiceDiscovery delegate) {
this.delegate = delegate;
}
public ServiceDiscovery(Object delegate) {
this.delegate = (io.vertx.servicediscovery.ServiceDiscovery)delegate;
}
public io.vertx.servicediscovery.ServiceDiscovery getDelegate() {
return delegate;
}
private static final TypeArg TYPE_ARG_0 = new TypeArg(o1 -> io.vertx.rxjava3.servicediscovery.ServiceReference.newInstance((io.vertx.servicediscovery.ServiceReference)o1), o1 -> o1.getDelegate());
/**
* Creates an instance of {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery}.
* @param vertx the vert.x instance
* @param options the discovery options
* @return the created service discovery instance.
*/
public static io.vertx.rxjava3.servicediscovery.ServiceDiscovery create(io.vertx.rxjava3.core.Vertx vertx, io.vertx.servicediscovery.ServiceDiscoveryOptions options) {
io.vertx.rxjava3.servicediscovery.ServiceDiscovery ret = io.vertx.rxjava3.servicediscovery.ServiceDiscovery.newInstance((io.vertx.servicediscovery.ServiceDiscovery)io.vertx.servicediscovery.ServiceDiscovery.create(vertx.getDelegate(), options));
return ret;
}
/**
* Creates a new instance of {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery} using the default configuration.
* @param vertx the vert.x instance
* @return the created instance
*/
public static io.vertx.rxjava3.servicediscovery.ServiceDiscovery create(io.vertx.rxjava3.core.Vertx vertx) {
io.vertx.rxjava3.servicediscovery.ServiceDiscovery ret = io.vertx.rxjava3.servicediscovery.ServiceDiscovery.newInstance((io.vertx.servicediscovery.ServiceDiscovery)io.vertx.servicediscovery.ServiceDiscovery.create(vertx.getDelegate()));
return ret;
}
/**
* Gets a service reference from the given record.
* @param record the chosen record
* @return the service reference, that allows retrieving the service object. Once called the service reference is cached, and need to be released.
*/
public io.vertx.rxjava3.servicediscovery.ServiceReference getReference(io.vertx.servicediscovery.Record record) {
io.vertx.rxjava3.servicediscovery.ServiceReference ret = io.vertx.rxjava3.servicediscovery.ServiceReference.newInstance((io.vertx.servicediscovery.ServiceReference)delegate.getReference(record));
return ret;
}
/**
* Gets a service reference from the given record, the reference is configured with the given json object.
* @param record the chosen record
* @param configuration the configuration
* @return the service reference, that allows retrieving the service object. Once called the service reference is cached, and need to be released.
*/
public io.vertx.rxjava3.servicediscovery.ServiceReference getReferenceWithConfiguration(io.vertx.servicediscovery.Record record, io.vertx.core.json.JsonObject configuration) {
io.vertx.rxjava3.servicediscovery.ServiceReference ret = io.vertx.rxjava3.servicediscovery.ServiceReference.newInstance((io.vertx.servicediscovery.ServiceReference)delegate.getReferenceWithConfiguration(record, configuration));
return ret;
}
/**
* Releases the service reference.
* @param reference the reference to release, must not be null
* @return whether or not the reference has been released.
*/
public boolean release(io.vertx.rxjava3.servicediscovery.ServiceReference reference) {
boolean ret = delegate.release(reference.getDelegate());
return ret;
}
/**
* Registers a discovery service importer. Importers let you integrate other discovery technologies in this service
* discovery.
* @param importer the service importer
* @param configuration the optional configuration
* @return the current {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery}
*/
public io.reactivex.rxjava3.core.Completable registerServiceImporter(io.vertx.rxjava3.servicediscovery.spi.ServiceImporter importer, io.vertx.core.json.JsonObject configuration) {
io.reactivex.rxjava3.core.Completable ret = rxRegisterServiceImporter(importer, configuration);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Registers a discovery service importer. Importers let you integrate other discovery technologies in this service
* discovery.
* @param importer the service importer
* @param configuration the optional configuration
* @return the current {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery}
*/
public io.reactivex.rxjava3.core.Completable rxRegisterServiceImporter(io.vertx.rxjava3.servicediscovery.spi.ServiceImporter importer, io.vertx.core.json.JsonObject configuration) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.registerServiceImporter(importer.getDelegate(), configuration, completionHandler);
});
}
/**
* Registers a discovery bridge. Exporters let you integrate other discovery technologies in this service
* discovery.
* @param exporter the service exporter
* @param configuration the optional configuration
* @return the current {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery}
*/
public io.reactivex.rxjava3.core.Completable registerServiceExporter(io.vertx.rxjava3.servicediscovery.spi.ServiceExporter exporter, io.vertx.core.json.JsonObject configuration) {
io.reactivex.rxjava3.core.Completable ret = rxRegisterServiceExporter(exporter, configuration);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Registers a discovery bridge. Exporters let you integrate other discovery technologies in this service
* discovery.
* @param exporter the service exporter
* @param configuration the optional configuration
* @return the current {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery}
*/
public io.reactivex.rxjava3.core.Completable rxRegisterServiceExporter(io.vertx.rxjava3.servicediscovery.spi.ServiceExporter exporter, io.vertx.core.json.JsonObject configuration) {
return AsyncResultCompletable.toCompletable( completionHandler -> {
delegate.registerServiceExporter(exporter.getDelegate(), configuration, completionHandler);
});
}
/**
* Closes the service discovery
*/
public void close() {
delegate.close();
}
/**
* Publishes a record.
* @param record the record
* @return
*/
public io.reactivex.rxjava3.core.Single publish(io.vertx.servicediscovery.Record record) {
io.reactivex.rxjava3.core.Single ret = rxPublish(record);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Publishes a record.
* @param record the record
* @return
*/
public io.reactivex.rxjava3.core.Single rxPublish(io.vertx.servicediscovery.Record record) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.publish(record, resultHandler);
});
}
/**
* Un-publishes a record.
* @param id the registration id
* @return
*/
public io.reactivex.rxjava3.core.Completable unpublish(java.lang.String id) {
io.reactivex.rxjava3.core.Completable ret = rxUnpublish(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Un-publishes a record.
* @param id the registration id
* @return
*/
public io.reactivex.rxjava3.core.Completable rxUnpublish(java.lang.String id) {
return AsyncResultCompletable.toCompletable( resultHandler -> {
delegate.unpublish(id, resultHandler);
});
}
/**
* Lookups for a single record.
*
* Filters are expressed using a Json object. Each entry of the given filter will be checked against the record.
* All entry must match exactly the record. The entry can use the special "*" value to denotes a requirement on the
* key, but not on the value.
*
* Let's take some example:
*
* { "name" = "a" } => matches records with name set fo "a"
* { "color" = "*" } => matches records with "color" set
* { "color" = "red" } => only matches records with "color" set to "red"
* { "color" = "red", "name" = "a"} => only matches records with name set to "a", and color set to "red"
*
*
* If the filter is not set (null
or empty), it accepts all records.
*
* This method returns the first matching record.
* @param filter the filter.
* @return
*/
public io.reactivex.rxjava3.core.Maybe getRecord(io.vertx.core.json.JsonObject filter) {
io.reactivex.rxjava3.core.Maybe ret = rxGetRecord(filter);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Lookups for a single record.
*
* Filters are expressed using a Json object. Each entry of the given filter will be checked against the record.
* All entry must match exactly the record. The entry can use the special "*" value to denotes a requirement on the
* key, but not on the value.
*
* Let's take some example:
*
* { "name" = "a" } => matches records with name set fo "a"
* { "color" = "*" } => matches records with "color" set
* { "color" = "red" } => only matches records with "color" set to "red"
* { "color" = "red", "name" = "a"} => only matches records with name set to "a", and color set to "red"
*
*
* If the filter is not set (null
or empty), it accepts all records.
*
* This method returns the first matching record.
* @param filter the filter.
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxGetRecord(io.vertx.core.json.JsonObject filter) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.getRecord(filter, resultHandler);
});
}
/**
* Looks up for a single record by its registration id
.
*
* When there are no matching record, the operation succeeds, but the async result has no result (null
).
* @param id the registration id
* @return
*/
public io.reactivex.rxjava3.core.Maybe getRecord(java.lang.String id) {
io.reactivex.rxjava3.core.Maybe ret = rxGetRecord(id);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Looks up for a single record by its registration id
.
*
* When there are no matching record, the operation succeeds, but the async result has no result (null
).
* @param id the registration id
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxGetRecord(java.lang.String id) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.getRecord(id, resultHandler);
});
}
/**
* Lookups for a single record.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* This method only looks for records with a UP
status.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @return
*/
public io.reactivex.rxjava3.core.Maybe getRecord(java.util.function.Function filter) {
io.reactivex.rxjava3.core.Maybe ret = rxGetRecord(filter);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Lookups for a single record.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* This method only looks for records with a UP
status.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxGetRecord(java.util.function.Function filter) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.getRecord(filter, resultHandler);
});
}
/**
* Lookups for a single record.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method may accept records with a OUT OF SERVICE
* status, if the includeOutOfService
parameter is set to true
.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @param includeOutOfService whether or not the filter accepts OUT OF SERVICE
records
* @return
*/
public io.reactivex.rxjava3.core.Maybe getRecord(java.util.function.Function filter, boolean includeOutOfService) {
io.reactivex.rxjava3.core.Maybe ret = rxGetRecord(filter, includeOutOfService);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Lookups for a single record.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method may accept records with a OUT OF SERVICE
* status, if the includeOutOfService
parameter is set to true
.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @param includeOutOfService whether or not the filter accepts OUT OF SERVICE
records
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxGetRecord(java.util.function.Function filter, boolean includeOutOfService) {
return AsyncResultMaybe.toMaybe( resultHandler -> {
delegate.getRecord(filter, includeOutOfService, resultHandler);
});
}
/**
* Lookups for a set of records. Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method returns all matching
* records.
* @param filter the filter - see {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}
* @return
*/
public io.reactivex.rxjava3.core.Single> getRecords(io.vertx.core.json.JsonObject filter) {
io.reactivex.rxjava3.core.Single> ret = rxGetRecords(filter);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Lookups for a set of records. Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method returns all matching
* records.
* @param filter the filter - see {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetRecords(io.vertx.core.json.JsonObject filter) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getRecords(filter, resultHandler);
});
}
/**
* Lookups for a set of records. Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method returns all matching
* records.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* This method only looks for records with a UP
status.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @return
*/
public io.reactivex.rxjava3.core.Single> getRecords(java.util.function.Function filter) {
io.reactivex.rxjava3.core.Single> ret = rxGetRecords(filter);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Lookups for a set of records. Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method returns all matching
* records.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* This method only looks for records with a UP
status.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetRecords(java.util.function.Function filter) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getRecords(filter, resultHandler);
});
}
/**
* Lookups for a set of records. Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method returns all matching
* records.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecords}, this method may accept records with a OUT OF SERVICE
* status, if the includeOutOfService
parameter is set to true
.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @param includeOutOfService whether or not the filter accepts OUT OF SERVICE
records
* @return
*/
public io.reactivex.rxjava3.core.Single> getRecords(java.util.function.Function filter, boolean includeOutOfService) {
io.reactivex.rxjava3.core.Single> ret = rxGetRecords(filter, includeOutOfService);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Lookups for a set of records. Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecord}, this method returns all matching
* records.
*
* The filter is a taking a {@link io.vertx.servicediscovery.Record} as argument and returning a boolean. You should see it
* as an accept
method of a filter. This method return a record passing the filter.
*
* Unlike {@link io.vertx.rxjava3.servicediscovery.ServiceDiscovery#getRecords}, this method may accept records with a OUT OF SERVICE
* status, if the includeOutOfService
parameter is set to true
.
* @param filter the filter, must not be null
. To return all records, use a function accepting all records
* @param includeOutOfService whether or not the filter accepts OUT OF SERVICE
records
* @return
*/
public io.reactivex.rxjava3.core.Single> rxGetRecords(java.util.function.Function filter, boolean includeOutOfService) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.getRecords(filter, includeOutOfService, resultHandler);
});
}
/**
* Updates the given record. The record must has been published, and has it's registration id set.
* @param record the updated record
* @return
*/
public io.reactivex.rxjava3.core.Single update(io.vertx.servicediscovery.Record record) {
io.reactivex.rxjava3.core.Single ret = rxUpdate(record);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Updates the given record. The record must has been published, and has it's registration id set.
* @param record the updated record
* @return
*/
public io.reactivex.rxjava3.core.Single rxUpdate(io.vertx.servicediscovery.Record record) {
return AsyncResultSingle.toSingle( resultHandler -> {
delegate.update(record, resultHandler);
});
}
/**
* @return the set of service references retrieved by this service discovery.
*/
public java.util.Set bindings() {
java.util.Set ret = delegate.bindings().stream().map(elt -> io.vertx.rxjava3.servicediscovery.ServiceReference.newInstance((io.vertx.servicediscovery.ServiceReference)elt)).collect(Collectors.toSet());
return ret;
}
/**
* @return the discovery options. Modifying the returned object would not update the discovery service configuration. This object should be considered as read-only.
*/
public io.vertx.servicediscovery.ServiceDiscoveryOptions options() {
io.vertx.servicediscovery.ServiceDiscoveryOptions ret = delegate.options();
return ret;
}
/**
* Release the service object retrieved using get
methods from the service type interface.
* It searches for the reference associated with the given object and release it.
* @param discovery the service discovery
* @param svcObject the service object
*/
public static void releaseServiceObject(io.vertx.rxjava3.servicediscovery.ServiceDiscovery discovery, java.lang.Object svcObject) {
io.vertx.servicediscovery.ServiceDiscovery.releaseServiceObject(discovery.getDelegate(), svcObject);
}
/**
* Constant for type field of usage events.
*/
public static final java.lang.String EVENT_TYPE = io.vertx.servicediscovery.ServiceDiscovery.EVENT_TYPE;
/**
* Constant for event type `Bind`.
*/
public static final java.lang.String EVENT_TYPE_BIND = io.vertx.servicediscovery.ServiceDiscovery.EVENT_TYPE_BIND;
/**
* Constant for event type `Release`.
*/
public static final java.lang.String EVENT_TYPE_RELEASE = io.vertx.servicediscovery.ServiceDiscovery.EVENT_TYPE_RELEASE;
/**
* Constant for record field of usage events.
*/
public static final java.lang.String EVENT_RECORD = io.vertx.servicediscovery.ServiceDiscovery.EVENT_RECORD;
/**
* Constant for id field of usage events.
*/
public static final java.lang.String EVENT_ID = io.vertx.servicediscovery.ServiceDiscovery.EVENT_ID;
public static ServiceDiscovery newInstance(io.vertx.servicediscovery.ServiceDiscovery arg) {
return arg != null ? new ServiceDiscovery(arg) : null;
}
}