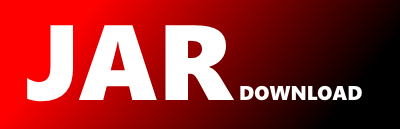
io.vertx.rxjava3.sqlclient.Pool Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava3.sqlclient;
import io.vertx.rxjava3.RxHelper;
import io.vertx.rxjava3.ObservableHelper;
import io.vertx.rxjava3.FlowableHelper;
import io.vertx.rxjava3.impl.AsyncResultMaybe;
import io.vertx.rxjava3.impl.AsyncResultSingle;
import io.vertx.rxjava3.impl.AsyncResultCompletable;
import io.vertx.rxjava3.WriteStreamObserver;
import io.vertx.rxjava3.WriteStreamSubscriber;
import java.util.Map;
import java.util.Set;
import java.util.List;
import java.util.Iterator;
import java.util.function.Function;
import java.util.stream.Collectors;
import io.vertx.core.Handler;
import io.vertx.core.AsyncResult;
import io.vertx.core.json.JsonObject;
import io.vertx.core.json.JsonArray;
import io.vertx.lang.rx.RxGen;
import io.vertx.lang.rx.TypeArg;
import io.vertx.lang.rx.MappingIterator;
/**
* A connection pool which reuses a number of SQL connections.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.sqlclient.Pool original} non RX-ified interface using Vert.x codegen.
*/
@RxGen(io.vertx.sqlclient.Pool.class)
public class Pool extends io.vertx.rxjava3.sqlclient.SqlClient {
@Override
public String toString() {
return delegate.toString();
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Pool that = (Pool) o;
return delegate.equals(that.delegate);
}
@Override
public int hashCode() {
return delegate.hashCode();
}
public static final TypeArg __TYPE_ARG = new TypeArg<>( obj -> new Pool((io.vertx.sqlclient.Pool) obj),
Pool::getDelegate
);
private final io.vertx.sqlclient.Pool delegate;
public Pool(io.vertx.sqlclient.Pool delegate) {
super(delegate);
this.delegate = delegate;
}
public Pool(Object delegate) {
super((io.vertx.sqlclient.Pool)delegate);
this.delegate = (io.vertx.sqlclient.Pool)delegate;
}
public io.vertx.sqlclient.Pool getDelegate() {
return delegate;
}
private static final TypeArg> TYPE_ARG_0 = new TypeArg>(o1 -> io.vertx.rxjava3.sqlclient.RowSet.newInstance((io.vertx.sqlclient.RowSet)o1, new TypeArg(o2 -> io.vertx.rxjava3.sqlclient.Row.newInstance((io.vertx.sqlclient.Row)o2), o2 -> o2.getDelegate())), o1 -> o1.getDelegate());
private static final TypeArg> TYPE_ARG_1 = new TypeArg>(o1 -> io.vertx.rxjava3.sqlclient.RowSet.newInstance((io.vertx.sqlclient.RowSet)o1, new TypeArg(o2 -> io.vertx.rxjava3.sqlclient.Row.newInstance((io.vertx.sqlclient.Row)o2), o2 -> o2.getDelegate())), o1 -> o1.getDelegate());
/**
* Like {@link io.vertx.rxjava3.sqlclient.Pool#pool} with default options.
* @param connectOptions
* @return
*/
public static io.vertx.rxjava3.sqlclient.Pool pool(io.vertx.sqlclient.SqlConnectOptions connectOptions) {
io.vertx.rxjava3.sqlclient.Pool ret = io.vertx.rxjava3.sqlclient.Pool.newInstance((io.vertx.sqlclient.Pool)io.vertx.sqlclient.Pool.pool(connectOptions));
return ret;
}
/**
* Like {@link io.vertx.rxjava3.sqlclient.Pool#pool} with a Vert.x instance created automatically.
* @param database
* @param options
* @return
*/
public static io.vertx.rxjava3.sqlclient.Pool pool(io.vertx.sqlclient.SqlConnectOptions database, io.vertx.sqlclient.PoolOptions options) {
io.vertx.rxjava3.sqlclient.Pool ret = io.vertx.rxjava3.sqlclient.Pool.newInstance((io.vertx.sqlclient.Pool)io.vertx.sqlclient.Pool.pool(database, options));
return ret;
}
/**
* Create a connection pool to the database
with the given options
.
*
* A will be selected among the drivers found on the classpath returning
* true
when applied to the first options
* of the list.
* @param vertx the Vertx instance to be used with the connection pool
* @param database the options used to create the connection pool, such as database hostname
* @param options the options for creating the pool
* @return the connection pool
*/
public static io.vertx.rxjava3.sqlclient.Pool pool(io.vertx.rxjava3.core.Vertx vertx, io.vertx.sqlclient.SqlConnectOptions database, io.vertx.sqlclient.PoolOptions options) {
io.vertx.rxjava3.sqlclient.Pool ret = io.vertx.rxjava3.sqlclient.Pool.newInstance((io.vertx.sqlclient.Pool)io.vertx.sqlclient.Pool.pool(vertx.getDelegate(), database, options));
return ret;
}
/**
* Get a connection from the pool.
* @return
*/
public io.reactivex.rxjava3.core.Single getConnection() {
io.reactivex.rxjava3.core.Single ret = rxGetConnection();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.SingleHelper.nullObserver());
return ret;
}
/**
* Get a connection from the pool.
* @return
*/
public io.reactivex.rxjava3.core.Single rxGetConnection() {
return AsyncResultSingle.toSingle( handler -> {
delegate.getConnection(new io.vertx.lang.rx.DelegatingHandler<>(handler, ar -> ar.map(event -> io.vertx.rxjava3.sqlclient.SqlConnection.newInstance((io.vertx.sqlclient.SqlConnection)event))));
});
}
/**
*
*
* A connection is borrowed from the connection pool when the query is executed and then immediately returned
* to the pool after it completes.
* @param sql
* @return
*/
public io.vertx.rxjava3.sqlclient.Query> query(java.lang.String sql) {
io.vertx.rxjava3.sqlclient.Query> ret = io.vertx.rxjava3.sqlclient.Query.newInstance((io.vertx.sqlclient.Query)delegate.query(sql), TYPE_ARG_0);
return ret;
}
/**
*
*
* A connection is borrowed from the connection pool when the query is executed and then immediately returned
* to the pool after it completes.
* @param sql
* @return
*/
public io.vertx.rxjava3.sqlclient.PreparedQuery> preparedQuery(java.lang.String sql) {
io.vertx.rxjava3.sqlclient.PreparedQuery> ret = io.vertx.rxjava3.sqlclient.PreparedQuery.newInstance((io.vertx.sqlclient.PreparedQuery)delegate.preparedQuery(sql), TYPE_ARG_1);
return ret;
}
/**
* Execute the given function
within a transaction.
*
* The function
is passed a client executing all operations within a transaction.
* When the future returned by the function
*
* - succeeds the transaction commits
* - fails the transaction rollbacks
*
*
* The handler
is given a success result when the function returns a succeeded futures and the transaction commits.
* Otherwise it is given a failure result.
* @param function the code to execute
* @return
*/
public io.reactivex.rxjava3.core.Maybe withTransaction(java.util.function.Function> function) {
io.reactivex.rxjava3.core.Maybe ret = rxWithTransaction(function);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Execute the given function
within a transaction.
*
* The function
is passed a client executing all operations within a transaction.
* When the future returned by the function
*
* - succeeds the transaction commits
* - fails the transaction rollbacks
*
*
* The handler
is given a success result when the function returns a succeeded futures and the transaction commits.
* Otherwise it is given a failure result.
* @param function the code to execute
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxWithTransaction(java.util.function.Function> function) {
return AsyncResultMaybe.toMaybe( handler -> {
delegate.withTransaction(new Function>() {
public io.vertx.core.Future apply(io.vertx.sqlclient.SqlConnection arg) {
io.reactivex.rxjava3.core.Maybe ret = function.apply(io.vertx.rxjava3.sqlclient.SqlConnection.newInstance((io.vertx.sqlclient.SqlConnection)arg));
return io.vertx.rxjava3.MaybeHelper.toFuture(ret, obj -> obj);
}
}, handler);
});
}
/**
* Like {@link io.vertx.rxjava3.sqlclient.Pool#withTransaction} but allows for setting the mode, defining how the acquired
* connection is managed during the execution of the function.
* @param txPropagation
* @param function
* @return
*/
public io.reactivex.rxjava3.core.Maybe withTransaction(io.vertx.sqlclient.TransactionPropagation txPropagation, java.util.function.Function> function) {
io.reactivex.rxjava3.core.Maybe ret = rxWithTransaction(txPropagation, function);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Like {@link io.vertx.rxjava3.sqlclient.Pool#withTransaction} but allows for setting the mode, defining how the acquired
* connection is managed during the execution of the function.
* @param txPropagation
* @param function
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxWithTransaction(io.vertx.sqlclient.TransactionPropagation txPropagation, java.util.function.Function> function) {
return AsyncResultMaybe.toMaybe( handler -> {
delegate.withTransaction(txPropagation, new Function>() {
public io.vertx.core.Future apply(io.vertx.sqlclient.SqlConnection arg) {
io.reactivex.rxjava3.core.Maybe ret = function.apply(io.vertx.rxjava3.sqlclient.SqlConnection.newInstance((io.vertx.sqlclient.SqlConnection)arg));
return io.vertx.rxjava3.MaybeHelper.toFuture(ret, obj -> obj);
}
}, handler);
});
}
/**
* Get a connection from the pool and execute the given function
.
*
* When the future returned by the function
completes, the connection is returned to the pool.
*
*
The handler
is given a success result when the function returns a succeeded futures.
* Otherwise it is given a failure result.
* @param function the code to execute
* @return
*/
public io.reactivex.rxjava3.core.Maybe withConnection(java.util.function.Function> function) {
io.reactivex.rxjava3.core.Maybe ret = rxWithConnection(function);
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.MaybeHelper.nullObserver());
return ret;
}
/**
* Get a connection from the pool and execute the given function
.
*
* When the future returned by the function
completes, the connection is returned to the pool.
*
*
The handler
is given a success result when the function returns a succeeded futures.
* Otherwise it is given a failure result.
* @param function the code to execute
* @return
*/
public io.reactivex.rxjava3.core.Maybe rxWithConnection(java.util.function.Function> function) {
return AsyncResultMaybe.toMaybe( handler -> {
delegate.withConnection(new Function>() {
public io.vertx.core.Future apply(io.vertx.sqlclient.SqlConnection arg) {
io.reactivex.rxjava3.core.Maybe ret = function.apply(io.vertx.rxjava3.sqlclient.SqlConnection.newInstance((io.vertx.sqlclient.SqlConnection)arg));
return io.vertx.rxjava3.MaybeHelper.toFuture(ret, obj -> obj);
}
}, handler);
});
}
/**
* Close the pool and release the associated resources.
* @return
*/
public io.reactivex.rxjava3.core.Completable close() {
io.reactivex.rxjava3.core.Completable ret = rxClose();
ret = ret.cache();
ret.subscribe(io.vertx.rxjava3.CompletableHelper.nullObserver());
return ret;
}
/**
* Close the pool and release the associated resources.
* @return
*/
public io.reactivex.rxjava3.core.Completable rxClose() {
return AsyncResultCompletable.toCompletable( handler -> {
delegate.close(handler);
});
}
/**
* Set an handler called when the pool has established a connection to the database.
*
* This handler allows interactions with the database before the connection is added to the pool.
*
*
When the handler has finished, it must call {@link io.vertx.rxjava3.sqlclient.SqlClient#close} to release the connection
* to the pool.
* @param handler the handler
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.sqlclient.Pool connectHandler(io.vertx.core.Handler handler) {
delegate.connectHandler(new io.vertx.lang.rx.DelegatingHandler<>(handler, event -> io.vertx.rxjava3.sqlclient.SqlConnection.newInstance((io.vertx.sqlclient.SqlConnection)event)));
return this;
}
/**
* Replace the default pool connection provider, the new provider
returns a future connection for a
* given .
*
* A {@link io.vertx.sqlclient.spi.ConnectionFactory} can be used as connection provider.
* @param provider the new connection provider
* @return a reference to this, so the API can be used fluently
*/
@Deprecated()
public io.vertx.rxjava3.sqlclient.Pool connectionProvider(java.util.function.Function> provider) {
delegate.connectionProvider(new Function>() {
public io.vertx.core.Future apply(io.vertx.core.Context arg) {
io.reactivex.rxjava3.core.Single ret = provider.apply(io.vertx.rxjava3.core.Context.newInstance((io.vertx.core.Context)arg));
return io.vertx.rxjava3.SingleHelper.toFuture(ret, obj -> obj.getDelegate());
}
});
return this;
}
/**
* @return the current pool size approximation
*/
public int size() {
int ret = delegate.size();
return ret;
}
public static Pool newInstance(io.vertx.sqlclient.Pool arg) {
return arg != null ? new Pool(arg) : null;
}
}