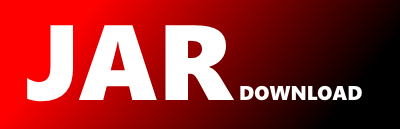
io.vertx.serviceproxy.HelperUtils Maven / Gradle / Ivy
/*
* Copyright 2021 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.serviceproxy;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
import io.vertx.core.eventbus.Message;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import java.util.*;
/**
* @author Francesco Guardiani @slinkydeveloper
*/
@SuppressWarnings({"unchecked", "rawtypes"})
public class HelperUtils {
public static Handler> createHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
if (res.result() != null && res.result().getClass().isEnum()) {
msg.reply(((Enum) res.result()).name());
} else {
msg.reply(res.result());
}
}
};
}
public static Handler>> createListHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
msg.reply(new JsonArray(res.result()));
}
};
}
public static Handler>> createSetHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
msg.reply(new JsonArray(new ArrayList<>(res.result())));
}
};
}
public static Handler>> createMapHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
msg.reply(new JsonObject(new HashMap<>(res.result())));
}
};
}
public static Handler>> createListCharHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
JsonArray arr = new JsonArray();
for (Character chr: res.result()) {
arr.add((int) chr);
}
msg.reply(arr);
}
};
}
public static Handler>> createSetCharHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
JsonArray arr = new JsonArray();
for (Character chr: res.result()) {
arr.add((int) chr);
}
msg.reply(arr);
}
};
}
public static Handler>> createMapCharHandler(Message msg, boolean includeDebugInfo) {
return res -> {
if (res.failed()) {
manageFailure(msg, res.cause(), includeDebugInfo);
} else {
JsonObject obj = new JsonObject();
for (Map.Entry chr: res.result().entrySet()) {
obj.put(chr.getKey(), (int) chr.getValue());
}
msg.reply(obj);
}
};
}
public static void manageFailure(Message msg, Throwable cause, boolean includeDebugInfo) {
if (cause instanceof ServiceException) {
msg.reply(cause);
} else {
if (includeDebugInfo)
msg.reply(new ServiceException(-1, cause.getMessage(), generateDebugInfo(cause)));
else
msg.reply(new ServiceException(-1, cause.getMessage()));
}
}
public static Map convertMap(Map map) {
return (Map)map;
}
public static List convertList(List list) {
return (List)list;
}
public static Set convertSet(List list) {
return new HashSet((List)list);
}
public static JsonObject generateDebugInfo(Throwable cause) {
if (cause == null) return null;
JsonObject obj = new JsonObject();
obj.put("causeName", cause.getClass().getCanonicalName());
obj.put("causeMessage", cause.getMessage());
obj.put("causeStackTrace", convertStackTrace(cause));
return obj;
}
public static JsonArray convertStackTrace(Throwable cause) {
if (cause == null || cause.getStackTrace() == null) return new JsonArray();
return Arrays
.stream(cause.getStackTrace())
.map(StackTraceElement::toString)
.collect(JsonArray::new, JsonArray::add, JsonArray::addAll);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy