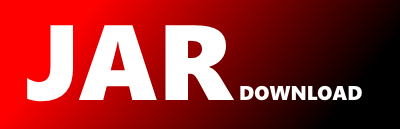
io.vertx.serviceproxy.ProxyUtils Maven / Gradle / Ivy
/*
* Copyright 2021 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*/
package io.vertx.serviceproxy;
import io.vertx.core.json.JsonArray;
import io.vertx.core.json.JsonObject;
import java.util.*;
import java.util.function.Function;
import java.util.stream.Collectors;
import java.util.stream.Stream;
/**
* @author Francesco Guardiani @slinkydeveloper
*/
@SuppressWarnings({"unchecked", "rawtypes"})
public class ProxyUtils {
public static List convertToListChar(JsonArray arr) {
return arr.stream().map(ProxyUtils::javaObjToChar).collect(Collectors.toList());
}
public static Set convertToSetChar(JsonArray arr) {
return arr.stream().map(ProxyUtils::javaObjToChar).collect(Collectors.toSet());
}
public static Map convertToMapChar(JsonObject obj) {
return obj.stream().collect(Collectors.toMap(Map.Entry::getKey, e -> javaObjToChar(e.getValue())));
}
public static Character javaObjToChar(Object obj) {
Integer jobj = (Integer)obj;
return (char)(int)jobj;
}
public static Map convertMap(Map map) {
if (map.isEmpty()) {
return (Map) map;
}
Object elem = map.values().stream().findFirst().get();
if (!(elem instanceof Map) && !(elem instanceof List)) {
return (Map) map;
} else {
Function
© 2015 - 2025 Weber Informatics LLC | Privacy Policy