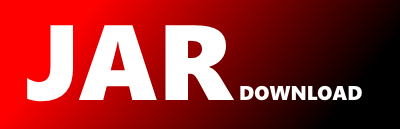
io.vertx.ext.shell.system.Process Maven / Gradle / Ivy
/*
* Copyright 2015 Red Hat, Inc.
*
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*
*
* Copyright (c) 2015 The original author or authors
* ------------------------------------------------------
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* and Apache License v2.0 which accompanies this distribution.
*
* The Eclipse Public License is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* The Apache License v2.0 is available at
* http://www.opensource.org/licenses/apache2.0.php
*
* You may elect to redistribute this code under either of these licenses.
*
*/
package io.vertx.ext.shell.system;
import io.vertx.codegen.annotations.CacheReturn;
import io.vertx.codegen.annotations.Fluent;
import io.vertx.codegen.annotations.VertxGen;
import io.vertx.core.Handler;
import io.vertx.ext.shell.term.Tty;
import io.vertx.ext.shell.session.Session;
/**
* A process managed by the shell.
*
* @author Julien Viet
*/
@VertxGen
public interface Process {
/**
* @return the current process status
*/
ExecStatus status();
/**
* @return the process exit code when the status is {@link ExecStatus#TERMINATED} otherwise {@code null}
*/
Integer exitCode();
/**
* Set the process tty.
*
* @param tty the process tty
* @return this object
*/
@Fluent
Process setTty(Tty tty);
/**
* @return the process tty
*/
@CacheReturn
Tty getTty();
/**
* Set the process session
*
* @param session the process session
* @return this object
*/
@Fluent
Process setSession(Session session);
/**
* @return the process session
*/
@CacheReturn
Session getSession();
/**
* Set an handler for being notified when the process terminates.
*
* @param handler the handler called when the process terminates.
* @return this object
*/
@Fluent
Process terminatedHandler(Handler handler);
/**
* Run the process.
*
*/
default void run() {
run(true);
}
/**
* Run the process.
*
*/
void run(boolean foregraound);
/**
* Attempt to interrupt the process.
*
* @return true if the process caught the signal
*/
default boolean interrupt() {
return interrupt(null);
}
/**
* Attempt to interrupt the process.
*
* @param completionHandler handler called after interrupt callback
* @return true if the process caught the signal
*/
boolean interrupt(Handler completionHandler);
/**
* Suspend the process.
*/
default void resume() {
resume(null);
}
/**
* Suspend the process.
*/
default void resume(boolean foreground) {
resume(foreground, null);
}
/**
* Suspend the process.
*
* @param completionHandler handler called after resume callback
*/
default void resume(Handler completionHandler) {
resume(true, completionHandler);
}
/**
* Suspend the process.
*
* @param completionHandler handler called after resume callback
*/
void resume(boolean foreground, Handler completionHandler);
/**
* Resume the process.
*/
default void suspend() {
suspend(null);
}
/**
* Resume the process.
*
* @param completionHandler handler called after suspend callback
*/
void suspend(Handler completionHandler);
/**
* Terminate the process.
*/
default void terminate() {
terminate(null);
}
/**
* Terminate the process.
*
* @param completionHandler handler called after end callback
*/
void terminate(Handler completionHandler);
/**
* Set the process in background.
*/
default void toBackground() {
toBackground(null);
}
/**
* Set the process in background.
*
* @param completionHandler handler called after background callback
*/
void toBackground(Handler completionHandler);
/**
* Set the process in foreground.
*/
default void toForeground() {
toForeground(null);
}
/**
* Set the process in foreground.
*
* @param completionHandler handler called after foreground callback
*/
void toForeground(Handler completionHandler);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy