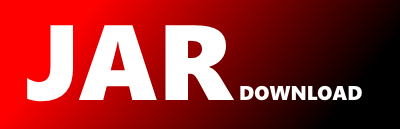
io.vertx.rxjava.ext.web.client.WebClient Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.web.client;
import java.util.Map;
import rx.Observable;
import rx.Single;
import io.vertx.ext.web.client.WebClientOptions;
import io.vertx.rxjava.core.buffer.Buffer;
import io.vertx.core.http.HttpMethod;
import io.vertx.rxjava.core.Vertx;
import io.vertx.core.http.RequestOptions;
import io.vertx.rxjava.core.http.HttpClient;
/**
* An asynchronous HTTP / HTTP/2 client called WebClient
.
*
* The web client makes easy to do HTTP request/response interactions with a web server, and provides advanced
* features like:
*
* - Json body encoding / decoding
* - request/response pumping
* - error handling
*
*
* The web client does not deprecate the , it is actually based on it and therefore inherits
* its configuration and great features like pooling. The HttpClient
should be used when fine grained control over the HTTP
* requests/response is necessary.
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.client.WebClient original} non RX-ified interface using Vert.x codegen.
*/
@io.vertx.lang.rxjava.RxGen(io.vertx.ext.web.client.WebClient.class)
public class WebClient {
public static final io.vertx.lang.rxjava.TypeArg __TYPE_ARG = new io.vertx.lang.rxjava.TypeArg<>(
obj -> new WebClient((io.vertx.ext.web.client.WebClient) obj),
WebClient::getDelegate
);
private final io.vertx.ext.web.client.WebClient delegate;
public WebClient(io.vertx.ext.web.client.WebClient delegate) {
this.delegate = delegate;
}
public io.vertx.ext.web.client.WebClient getDelegate() {
return delegate;
}
/**
* Create a web client using the provided vertx
instance and default options.
* @param vertx the vertx instance
* @return the created web client
*/
public static WebClient create(Vertx vertx) {
WebClient ret = WebClient.newInstance(io.vertx.ext.web.client.WebClient.create(vertx.getDelegate()));
return ret;
}
/**
* Create a web client using the provided vertx
instance.
* @param vertx the vertx instance
* @param options the Web Client options
* @return the created web client
*/
public static WebClient create(Vertx vertx, WebClientOptions options) {
WebClient ret = WebClient.newInstance(io.vertx.ext.web.client.WebClient.create(vertx.getDelegate(), options));
return ret;
}
/**
* Wrap an httpClient
with a web client and default options.
* @param httpClient the to wrap
* @return the web client
*/
public static WebClient wrap(HttpClient httpClient) {
WebClient ret = WebClient.newInstance(io.vertx.ext.web.client.WebClient.wrap(httpClient.getDelegate()));
return ret;
}
/**
* Wrap an httpClient
with a web client and default options.
*
* Only the specific web client portion of the options
is used, the {@link io.vertx.core.http.HttpClientOptions}
* of the httpClient
is reused.
* @param httpClient the to wrap
* @param options the Web Client options
* @return the web client
*/
public static WebClient wrap(HttpClient httpClient, WebClientOptions options) {
WebClient ret = WebClient.newInstance(io.vertx.ext.web.client.WebClient.wrap(httpClient.getDelegate(), options));
return ret;
}
/**
* Create an HTTP request to send to the server at the specified host and port.
* @param method the HTTP method
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest request(HttpMethod method, int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.request(method, port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP request to send to the server at the specified host and default port.
* @param method the HTTP method
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest request(HttpMethod method, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.request(method, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP request to send to the server at the default host and port.
* @param method the HTTP method
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest request(HttpMethod method, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.request(method, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP request to send to the server at the specified host and port.
* @param method the HTTP method
* @param options the request options
* @return an HTTP client request object
*/
public HttpRequest request(HttpMethod method, RequestOptions options) {
HttpRequest ret = HttpRequest.newInstance(delegate.request(method, options), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP request to send to the server using an absolute URI
* @param method the HTTP method
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest requestAbs(HttpMethod method, String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.requestAbs(method, absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP GET request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest get(String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.get(requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP GET request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest get(int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.get(port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP GET request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest get(String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.get(host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP GET request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest getAbs(String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.getAbs(absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP POST request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest post(String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.post(requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP POST request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest post(int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.post(port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP POST request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest post(String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.post(host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP POST request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest postAbs(String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.postAbs(absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PUT request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest put(String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.put(requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PUT request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest put(int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.put(port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PUT request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest put(String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.put(host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PUT request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest putAbs(String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.putAbs(absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest delete(String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.delete(requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest delete(int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.delete(port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest delete(String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.delete(host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP DELETE request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest deleteAbs(String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.deleteAbs(absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest patch(String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.patch(requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest patch(int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.patch(port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest patch(String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.patch(host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP PATCH request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest patchAbs(String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.patchAbs(absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server at the default host and port.
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest head(String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.head(requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server at the specified host and port.
* @param port the port
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest head(int port, String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.head(port, host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server at the specified host and default port.
* @param host the host
* @param requestURI the relative URI
* @return an HTTP client request object
*/
public HttpRequest head(String host, String requestURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.head(host, requestURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Create an HTTP HEAD request to send to the server using an absolute URI, specifying a response handler to receive
* the response
* @param absoluteURI the absolute URI
* @return an HTTP client request object
*/
public HttpRequest headAbs(String absoluteURI) {
HttpRequest ret = HttpRequest.newInstance(delegate.headAbs(absoluteURI), io.vertx.rxjava.core.buffer.Buffer.__TYPE_ARG);
return ret;
}
/**
* Close the client. Closing will close down any pooled connections.
* Clients should always be closed after use.
*/
public void close() {
delegate.close();
}
public static WebClient newInstance(io.vertx.ext.web.client.WebClient arg) {
return arg != null ? new WebClient(arg) : null;
}
}