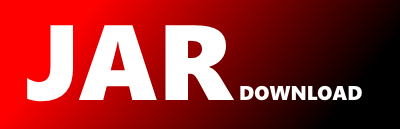
io.vertx.rxjava.ext.web.client.HttpRequest Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.rxjava.ext.web.client;
import java.util.Map;
import rx.Observable;
import rx.Single;
import io.vertx.rxjava.core.buffer.Buffer;
import io.vertx.core.http.HttpMethod;
import io.vertx.rxjava.ext.web.codec.BodyCodec;
import io.vertx.rxjava.core.MultiMap;
import io.vertx.rxjava.core.streams.ReadStream;
import io.vertx.core.json.JsonObject;
import io.vertx.core.AsyncResult;
import io.vertx.core.Handler;
/**
* A client-side HTTP request.
*
* Instances are created by an {@link io.vertx.rxjava.ext.web.client.WebClient} instance, via one of the methods corresponding to the specific
* HTTP methods such as {@link io.vertx.rxjava.ext.web.client.WebClient#get}, etc...
*
* The request shall be configured prior sending, the request is immutable and when a mutator method
* is called, a new request is returned allowing to expose the request in a public API and apply further customization.
*
* After the request has been configured, the methods
*
* - {@link io.vertx.rxjava.ext.web.client.HttpRequest#send}
* - {@link io.vertx.rxjava.ext.web.client.HttpRequest#sendStream}
* - {@link io.vertx.rxjava.ext.web.client.HttpRequest#sendJson} ()}
* - {@link io.vertx.rxjava.ext.web.client.HttpRequest#sendForm}
*
* can be called.
* The sendXXX
methods perform the actual request, they can be called multiple times to perform the same HTTP
* request at different points in time.
*
* The handler is called back with
*
* - an {@link io.vertx.rxjava.ext.web.client.HttpResponse} instance when the HTTP response has been received
* - a failure when the HTTP request failed (like a connection error) or when the HTTP response could
* not be obtained (like connection or unmarshalling errors)
*
*
* Most of the time, this client will buffer the HTTP response fully unless a specific is used
* such as .
*
*
* NOTE: This class has been automatically generated from the {@link io.vertx.ext.web.client.HttpRequest original} non RX-ified interface using Vert.x codegen.
*/
@io.vertx.lang.rxjava.RxGen(io.vertx.ext.web.client.HttpRequest.class)
public class HttpRequest {
public static final io.vertx.lang.rxjava.TypeArg __TYPE_ARG = new io.vertx.lang.rxjava.TypeArg<>(
obj -> new HttpRequest((io.vertx.ext.web.client.HttpRequest) obj),
HttpRequest::getDelegate
);
private final io.vertx.ext.web.client.HttpRequest delegate;
public final io.vertx.lang.rxjava.TypeArg __typeArg_0;
public HttpRequest(io.vertx.ext.web.client.HttpRequest delegate) {
this.delegate = delegate;
this.__typeArg_0 = io.vertx.lang.rxjava.TypeArg.unknown();
}
public HttpRequest(io.vertx.ext.web.client.HttpRequest delegate, io.vertx.lang.rxjava.TypeArg typeArg_0) {
this.delegate = delegate;
this.__typeArg_0 = typeArg_0;
}
public io.vertx.ext.web.client.HttpRequest getDelegate() {
return delegate;
}
/**
* Configure the request to use a new method value
.
* @param value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest method(HttpMethod value) {
delegate.method(value);
return this;
}
/**
* Configure the request to use a new port value
.
* @param value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest port(int value) {
delegate.port(value);
return this;
}
/**
* Configure the request to decode the response with the responseCodec
.
* @param responseCodec the response codec
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest as(BodyCodec responseCodec) {
HttpRequest ret = HttpRequest.newInstance(delegate.as(responseCodec.getDelegate()), responseCodec.__typeArg_0);
return ret;
}
/**
* Configure the request to use a new host value
.
* @param value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest host(String value) {
delegate.host(value);
return this;
}
/**
* Configure the request to use a new request URI value
.
*
* When the uri has query parameters, they are set in the {@link io.vertx.rxjava.ext.web.client.HttpRequest#queryParams} multimap, overwritting
* any parameters previously set.
* @param value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest uri(String value) {
delegate.uri(value);
return this;
}
/**
* Configure the request to add a new HTTP header.
* @param name the header name
* @param value the header value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest putHeader(String name, String value) {
delegate.putHeader(name, value);
return this;
}
/**
* @return The HTTP headers
*/
public MultiMap headers() {
if (cached_0 != null) {
return cached_0;
}
MultiMap ret = MultiMap.newInstance(delegate.headers());
cached_0 = ret;
return ret;
}
public HttpRequest ssl(boolean value) {
delegate.ssl(value);
return this;
}
/**
* Configures the amount of time in milliseconds after which if the request does not return any data within the timeout
* period an {@link java.util.concurrent.TimeoutException} fails the request.
*
* Setting zero or a negative value
disables the timeout.
* @param value The quantity of time in milliseconds.
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest timeout(long value) {
delegate.timeout(value);
return this;
}
/**
* Add a query parameter to the request.
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest addQueryParam(String paramName, String paramValue) {
delegate.addQueryParam(paramName, paramValue);
return this;
}
/**
* Set a query parameter to the request.
* @param paramName the param name
* @param paramValue the param value
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest setQueryParam(String paramName, String paramValue) {
delegate.setQueryParam(paramName, paramValue);
return this;
}
/**
* Set wether or not to follow the directs for the request.
* @param value true if redirections should be followed
* @return a reference to this, so the API can be used fluently
*/
public HttpRequest followRedirects(boolean value) {
delegate.followRedirects(value);
return this;
}
/**
* Return the current query parameters.
* @return the current query parameters
*/
public MultiMap queryParams() {
MultiMap ret = MultiMap.newInstance(delegate.queryParams());
return ret;
}
/**
* Copy this request
* @return a copy of this request
*/
public HttpRequest copy() {
HttpRequest ret = HttpRequest.newInstance(delegate.copy(), __typeArg_0);
return ret;
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
stream.
* @param body the body
* @param handler
*/
public void sendStream(ReadStream body, Handler>> handler) {
delegate.sendStream(body.getDelegate(), new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
stream.
* @param body the body
* @return
*/
public Single> rxSendStream(ReadStream body) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
sendStream(body, fut);
}));
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
buffer.
* @param body the body
* @param handler
*/
public void sendBuffer(Buffer body, Handler>> handler) {
delegate.sendBuffer(body.getDelegate(), new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
buffer.
* @param body the body
* @return
*/
public Single> rxSendBuffer(Buffer body) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
sendBuffer(body, fut);
}));
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @param handler
*/
public void sendJsonObject(JsonObject body, Handler>> handler) {
delegate.sendJsonObject(body, new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @return
*/
public Single> rxSendJsonObject(JsonObject body) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
sendJsonObject(body, fut);
}));
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @param handler
*/
public void sendJson(Object body, Handler>> handler) {
delegate.sendJson(body, new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
object encoded as json and the content type
* set to application/json
.
* @param body the body
* @return
*/
public Single> rxSendJson(Object body) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
sendJson(body, fut);
}));
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to application/x-www-form-urlencoded
.
*
* When the content type header is previously set to multipart/form-data
it will be used instead.
* @param body the body
* @param handler
*/
public void sendForm(MultiMap body, Handler>> handler) {
delegate.sendForm(body.getDelegate(), new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
multimap encoded as form and the content type
* set to application/x-www-form-urlencoded
.
*
* When the content type header is previously set to multipart/form-data
it will be used instead.
* @param body the body
* @return
*/
public Single> rxSendForm(MultiMap body) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
sendForm(body, fut);
}));
}
/**
* Send a request, the handler
will receive the response as an {@link io.vertx.rxjava.ext.web.client.HttpResponse}.
* @param handler
*/
public void send(Handler>> handler) {
delegate.send(new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Send a request, the handler
will receive the response as an {@link io.vertx.rxjava.ext.web.client.HttpResponse}.
* @return
*/
public Single> rxSend() {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
send(fut);
}));
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
stream.
* @param body the body
* @param handler
*/
public void sendStream(Observable body, Handler>> handler) {
delegate.sendStream(io.vertx.rx.java.ReadStreamSubscriber.asReadStream(body,obj -> (io.vertx.core.buffer.Buffer)obj.getDelegate()).resume(), new Handler>>() {
public void handle(AsyncResult> ar) {
if (ar.succeeded()) {
handler.handle(io.vertx.core.Future.succeededFuture(HttpResponse.newInstance(ar.result(), __typeArg_0)));
} else {
handler.handle(io.vertx.core.Future.failedFuture(ar.cause()));
}
}
});
}
/**
* Like {@link io.vertx.rxjava.ext.web.client.HttpRequest#send} but with an HTTP request body
stream.
* @param body the body
* @return
*/
public Single> rxSendStream(Observable body) {
return Single.create(new io.vertx.rx.java.SingleOnSubscribeAdapter<>(fut -> {
sendStream(body, fut);
}));
}
private MultiMap cached_0;
public static HttpRequest newInstance(io.vertx.ext.web.client.HttpRequest arg) {
return arg != null ? new HttpRequest (arg) : null;
}
public static HttpRequest newInstance(io.vertx.ext.web.client.HttpRequest arg, io.vertx.lang.rxjava.TypeArg __typeArg_T) {
return arg != null ? new HttpRequest (arg, __typeArg_T) : null;
}
}