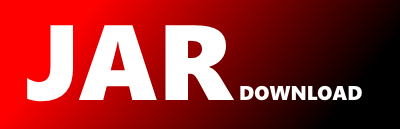
io.vertx.groovy.ext.web.RoutingContext.groovy Maven / Gradle / Ivy
/*
* Copyright 2014 Red Hat, Inc.
*
* Red Hat licenses this file to you under the Apache License, version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at:
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations
* under the License.
*/
package io.vertx.groovy.ext.web;
import groovy.transform.CompileStatic
import io.vertx.lang.groovy.InternalHelper
import io.vertx.core.json.JsonObject
import io.vertx.groovy.core.http.HttpServerRequest
import io.vertx.groovy.core.Vertx
import java.util.Set
import io.vertx.core.json.JsonArray
import java.util.List
import io.vertx.groovy.ext.auth.User
import io.vertx.groovy.core.buffer.Buffer
import io.vertx.groovy.core.http.HttpServerResponse
import io.vertx.core.http.HttpMethod
import java.util.Map
import io.vertx.core.json.JsonObject
import io.vertx.core.Handler
/**
* Represents the context for the handling of a request in Vert.x-Web.
*
* A new instance is created for each HTTP request that is received in the
* {@link io.vertx.groovy.ext.web.Router#accept} of the router.
*
* The same instance is passed to any matching request or failure handlers during the routing of the request or
* failure.
*
* The context provides access to the and
* and allows you to maintain arbitrary data that lives for the lifetime of the context. Contexts are discarded once they
* have been routed to the handler for the request.
*
* The context also provides access to the {@link io.vertx.groovy.ext.web.Session}, cookies and body for the request, given the correct handlers
* in the application.
*/
@CompileStatic
public class RoutingContext {
private final def io.vertx.ext.web.RoutingContext delegate;
public RoutingContext(Object delegate) {
this.delegate = (io.vertx.ext.web.RoutingContext) delegate;
}
public Object getDelegate() {
return delegate;
}
/**
* @return the HTTP request object
* @return
*/
public HttpServerRequest request() {
if (cached_0 != null) {
return cached_0;
}
def ret = InternalHelper.safeCreate(delegate.request(), io.vertx.groovy.core.http.HttpServerRequest.class);
cached_0 = ret;
return ret;
}
/**
* @return the HTTP response object
* @return
*/
public HttpServerResponse response() {
if (cached_1 != null) {
return cached_1;
}
def ret = InternalHelper.safeCreate(delegate.response(), io.vertx.groovy.core.http.HttpServerResponse.class);
cached_1 = ret;
return ret;
}
/**
* Tell the router to route this context to the next matching route (if any).
* This method, if called, does not need to be called during the execution of the handler, it can be called
* some arbitrary time later, if required.
*
* If next is not called for a handler then the handler should make sure it ends the response or no response
* will be sent.
*/
public void next() {
delegate.next();
}
/**
* Fail the context with the specified status code.
*
* This will cause the router to route the context to any matching failure handlers for the request. If no failure handlers
* match a default failure response will be sent.
* @param statusCode the HTTP status code
*/
public void fail(int statusCode) {
delegate.fail(statusCode);
}
/**
* Fail the context with the specified throwable.
*
* This will cause the router to route the context to any matching failure handlers for the request. If no failure handlers
* match a default failure response with status code 500 will be sent.
* @param throwable a throwable representing the failure
*/
public void fail(Throwable throwable) {
delegate.fail(throwable);
}
/**
* Put some arbitrary data in the context. This will be available in any handlers that receive the context.
* @param key the key for the data
* @param obj the data
* @return a reference to this, so the API can be used fluently
*/
public RoutingContext put(String key, Object obj) {
delegate.put(key, obj != null ? InternalHelper.unwrapObject(obj) : null);
return this;
}
/**
* Get some data from the context. The data is available in any handlers that receive the context.
* @param key the key for the data
* @return the data
*/
public T get(String key) {
def ret = (T) InternalHelper.wrapObject(delegate.get(key));
return ret;
}
/**
* @return the Vert.x instance associated to the initiating {@link io.vertx.groovy.ext.web.Router} for this context
* @return
*/
public Vertx vertx() {
def ret = InternalHelper.safeCreate(delegate.vertx(), io.vertx.groovy.core.Vertx.class);
return ret;
}
/**
* @return the mount point for this router. It will be null for a top level router. For a sub-router it will be the path
* at which the subrouter was mounted.
* @return
*/
public String mountPoint() {
def ret = delegate.mountPoint();
return ret;
}
/**
* @return the current route this context is being routed through.
* @return
*/
public Route currentRoute() {
def ret = InternalHelper.safeCreate(delegate.currentRoute(), io.vertx.groovy.ext.web.Route.class);
return ret;
}
/**
* Return the normalised path for the request.
*
* The normalised path is where the URI path has been decoded, i.e. any unicode or other illegal URL characters that
* were encoded in the original URL with `%` will be returned to their original form. E.g. `%20` will revert to a space.
* Also `+` reverts to a space in a query.
*
* The normalised path will also not contain any `..` character sequences to prevent resources being accessed outside
* of the permitted area.
*
* It's recommended to always use the normalised path as opposed to
* if accessing server resources requested by a client.
* @return the normalised path
*/
public String normalisedPath() {
def ret = delegate.normalisedPath();
return ret;
}
/**
* Get the cookie with the specified name. The context must have first been routed to a {@link io.vertx.groovy.ext.web.handler.CookieHandler}
* for this to work.
* @param name the cookie name
* @return the cookie
*/
public Cookie getCookie(String name) {
def ret = InternalHelper.safeCreate(delegate.getCookie(name), io.vertx.groovy.ext.web.Cookie.class);
return ret;
}
/**
* Add a cookie. This will be sent back to the client in the response. The context must have first been routed
* to a {@link io.vertx.groovy.ext.web.handler.CookieHandler} for this to work.
* @param cookie the cookie
* @return a reference to this, so the API can be used fluently
*/
public RoutingContext addCookie(Cookie cookie) {
delegate.addCookie(cookie != null ? (io.vertx.ext.web.Cookie)cookie.getDelegate() : null);
return this;
}
/**
* Remove a cookie. The context must have first been routed to a {@link io.vertx.groovy.ext.web.handler.CookieHandler}
* for this to work.
* @param name the name of the cookie
* @return the cookie, if it existed, or null
*/
public Cookie removeCookie(String name) {
def ret = InternalHelper.safeCreate(delegate.removeCookie(name), io.vertx.groovy.ext.web.Cookie.class);
return ret;
}
/**
* @return the number of cookies. The context must have first been routed to a {@link io.vertx.groovy.ext.web.handler.CookieHandler}
* for this to work.
* @return
*/
public int cookieCount() {
def ret = delegate.cookieCount();
return ret;
}
/**
* @return a set of all the cookies. The context must have first been routed to a {@link io.vertx.groovy.ext.web.handler.CookieHandler}
* for this to be populated.
* @return
*/
public Set cookies() {
def ret = (Set)delegate.cookies()?.collect({InternalHelper.safeCreate(it, io.vertx.groovy.ext.web.Cookie.class)}) as Set;
return ret;
}
/**
* @return the entire HTTP request body as a string, assuming UTF-8 encoding. The context must have first been routed to a
* {@link io.vertx.groovy.ext.web.handler.BodyHandler} for this to be populated.
* @return
*/
public String getBodyAsString() {
def ret = delegate.getBodyAsString();
return ret;
}
/**
* Get the entire HTTP request body as a string, assuming the specified encoding. The context must have first been routed to a
* {@link io.vertx.groovy.ext.web.handler.BodyHandler} for this to be populated.
* @param encoding the encoding, e.g. "UTF-16"
* @return the body
*/
public String getBodyAsString(String encoding) {
def ret = delegate.getBodyAsString(encoding);
return ret;
}
/**
* @return Get the entire HTTP request body as a . The context must have first been routed to a
* {@link io.vertx.groovy.ext.web.handler.BodyHandler} for this to be populated.
* @return
*/
public Map getBodyAsJson() {
def ret = (Map)InternalHelper.wrapObject(delegate.getBodyAsJson());
return ret;
}
/**
* @return Get the entire HTTP request body as a . The context must have first been routed to a
* {@link io.vertx.groovy.ext.web.handler.BodyHandler} for this to be populated.
* @return
*/
public List