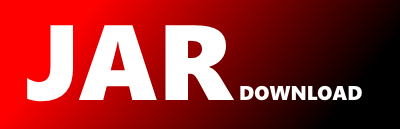
io.virtdata.reflection.DeferredConstructor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of virtdata-lib-realer Show documentation
Show all versions of virtdata-lib-realer Show documentation
With inspiration from other libraries
package io.virtdata.reflection;
import org.apache.commons.lang3.reflect.ConstructorUtils;
import java.util.Arrays;
import java.util.stream.Collectors;
public class DeferredConstructor {
private Class classToConstruct;
private Object[] args;
public DeferredConstructor(Class classToConstruct, Object... args) {
this.classToConstruct = classToConstruct;
this.args = args;
}
public DeferredConstructor prefixArgs(Object... prefixArgs) {
Object[] fullArgs = new Object[ prefixArgs.length + args.length ];
System.arraycopy(prefixArgs,0,fullArgs,0,prefixArgs.length);
System.arraycopy(args,0,fullArgs,prefixArgs.length,args.length);
return new DeferredConstructor(classToConstruct, fullArgs);
}
public T construct() {
T constructed = null;
try {
constructed = ConstructorUtils.invokeConstructor(classToConstruct, args);
} catch (Exception e) {
throw new RuntimeException("Error invoking constructor for:" + this.toString());
}
return constructed;
}
public String toString() {
return "class:" + classToConstruct.getName() + ", args:" +
Arrays.stream(args)
.map(String::valueOf)
.collect(Collectors.joining("[", ",", "]"));
}
public void validate() {
try {
construct();
} catch (Exception e) {
throw new RuntimeException("Error while validating DeferredConstructor for " + this.toString(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy