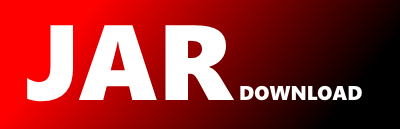
org.antlr.v4.tool.templates.codegen.CSharp.CSharp.stg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of virtdata-lib-realer Show documentation
Show all versions of virtdata-lib-realer Show documentation
With inspiration from other libraries
/*
* [The "BSD license"]
* Copyright (c) 2013 Terence Parr
* Copyright (c) 2013 Sam Harwell
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
// args must be ,
ParserFile(file, parser, namedActions, contextSuperClass) ::= <<
namespace {
using System;
using System.IO;
using System.Text;
using System.Diagnostics;
using System.Collections.Generic;
using Antlr4.Runtime;
using Antlr4.Runtime.Atn;
using Antlr4.Runtime.Misc;
using Antlr4.Runtime.Tree;
using DFA = Antlr4.Runtime.Dfa.DFA;
} // namespace
>>
ListenerFile(file, header, namedActions) ::= <<
namespace {
using Antlr4.Runtime.Misc;
using IParseTreeListener = Antlr4.Runtime.Tree.IParseTreeListener;
using IToken = Antlr4.Runtime.IToken;
/// \
/// This interface defines a complete listener for a parse tree produced by
/// \ .
/// \
[System.CodeDom.Compiler.GeneratedCode("ANTLR", "")]
[System.CLSCompliant(false)]
public interface IListener : IParseTreeListener {
/// Enter a parse tree produced by the \\
/// labeled alternative in \ .
/// Enter a parse tree produced by \ .
/// \
/// \The parse tree.\
void Enter([NotNull] .Context context);
/// \
/// Exit a parse tree produced by the \\
/// labeled alternative in \ .
/// Exit a parse tree produced by \ .
/// \
/// \The parse tree.\
void Exit([NotNull] .Context context);}; separator="\n">
}
} // namespace
>>
BaseListenerFile(file, header, namedActions) ::= <<
namespace {
using Antlr4.Runtime.Misc;
using IErrorNode = Antlr4.Runtime.Tree.IErrorNode;
using ITerminalNode = Antlr4.Runtime.Tree.ITerminalNode;
using IToken = Antlr4.Runtime.IToken;
using ParserRuleContext = Antlr4.Runtime.ParserRuleContext;
/// \
/// This class provides an empty implementation of \ ,
/// which can be extended to create a listener which only needs to handle a subset
/// of the available methods.
/// \
[System.CodeDom.Compiler.GeneratedCode("ANTLR", "")]
[System.CLSCompliant(false)]
public partial class BaseListener : IListener {
/// Enter a parse tree produced by the \\
/// labeled alternative in \ .
/// Enter a parse tree produced by \ .
/// \The default implementation does nothing.\
/// \
/// \The parse tree.\
public virtual void Enter([NotNull] .Context context) { \}
/// \
/// Exit a parse tree produced by the \\
/// labeled alternative in \ .
/// Exit a parse tree produced by \ .
/// \The default implementation does nothing.\
/// \
/// \The parse tree.\
public virtual void Exit([NotNull] .Context context) { \}}; separator="\n">
/// \
/// \The default implementation does nothing.\
public virtual void EnterEveryRule([NotNull] ParserRuleContext context) { }
/// \
/// \The default implementation does nothing.\
public virtual void ExitEveryRule([NotNull] ParserRuleContext context) { }
/// \
/// \The default implementation does nothing.\
public virtual void VisitTerminal([NotNull] ITerminalNode node) { }
/// \
/// \The default implementation does nothing.\
public virtual void VisitErrorNode([NotNull] IErrorNode node) { }
}
} // namespace
>>
VisitorFile(file, header, namedActions) ::= <<
namespace {
using Antlr4.Runtime.Misc;
using Antlr4.Runtime.Tree;
using IToken = Antlr4.Runtime.IToken;
/// \
/// This interface defines a complete generic visitor for a parse tree produced
/// by \ .
/// \
/// \The return type of the visit operation.\
[System.CodeDom.Compiler.GeneratedCode("ANTLR", "")]
[System.CLSCompliant(false)]
public interface IVisitor\ : IParseTreeVisitor\ {
/// Visit a parse tree produced by the \\
/// labeled alternative in \ .
/// Visit a parse tree produced by \ .
/// \
/// \The parse tree.\
/// \The visitor result.\
Result Visit([NotNull] .Context context);}; separator="\n">
}
} // namespace
>>
BaseVisitorFile(file, header, namedActions) ::= <<
namespace {
using Antlr4.Runtime.Misc;
using Antlr4.Runtime.Tree;
using IToken = Antlr4.Runtime.IToken;
using ParserRuleContext = Antlr4.Runtime.ParserRuleContext;
/// \
/// This class provides an empty implementation of \ ,
/// which can be extended to create a visitor which only needs to handle a subset
/// of the available methods.
/// \
/// \The return type of the visit operation.\
[System.CodeDom.Compiler.GeneratedCode("ANTLR", "")]
[System.CLSCompliant(false)]
public partial class BaseVisitor\ : AbstractParseTreeVisitor\, IVisitor\ {
/// Visit a parse tree produced by the \\
/// labeled alternative in \ .
/// Visit a parse tree produced by \ .
/// \
/// The default implementation returns the result of calling \
/// on \ .
/// \
/// \
/// \The parse tree.\
/// \The visitor result.\
public virtual Result Visit([NotNull] .Context context) { return VisitChildren(context); \}}; separator="\n">
}
} // namespace
>>
fileHeader(grammarFileName, ANTLRVersion) ::= <<
//------------------------------------------------------------------------------
// \
// This code was generated by a tool.
// ANTLR Version:
//
// Changes to this file may cause incorrect behavior and will be lost if
// the code is regenerated.
// \
//------------------------------------------------------------------------------
// Generated from by ANTLR
// Unreachable code detected
#pragma warning disable 0162
// The variable '...' is assigned but its value is never used
#pragma warning disable 0219
// Missing XML comment for publicly visible type or member '...'
#pragma warning disable 1591
// Ambiguous reference in cref attribute
#pragma warning disable 419
>>
Parser(parser, funcs, atn, sempredFuncs, superClass) ::= <<
>>
Parser_(parser, funcs, atn, sempredFuncs, ctor, superClass) ::= <<
[System.CodeDom.Compiler.GeneratedCode("ANTLR", "")]
[System.CLSCompliant(false)]
public partial class : {
protected static DFA[] decisionToDFA;
protected static PredictionContextCache sharedContextCache = new PredictionContextCache();
public const int
=}; separator=", ", wrap, anchor>;
public const int
= }; separator=", ", wrap, anchor>;
public static readonly string[] ruleNames = {
"}; separator=", ", wrap, anchor>
};
public override string GrammarFileName { get { return ""; } }
public override string[] RuleNames { get { return ruleNames; } }
public override string SerializedAtn { get { return new string(_serializedATN); } }
static () {
decisionToDFA = new DFA[_ATN.NumberOfDecisions];
for (int i = 0; i \< _ATN.NumberOfDecisions; i++) {
decisionToDFA[i] = new DFA(_ATN.GetDecisionState(i), i);
}
}
public override bool Sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
: return _sempred(()_localctx, predIndex);}; separator="\n">
}
return true;
}
}
>>
vocabulary(literalNames, symbolicNames) ::= <<
private static readonly string[] _LiteralNames = {
}; null="null", separator=", ", wrap, anchor>
};
private static readonly string[] _SymbolicNames = {
}; null="null", separator=", ", wrap, anchor>
};
public static readonly IVocabulary DefaultVocabulary = new Vocabulary(_LiteralNames, _SymbolicNames);
[NotNull]
public override IVocabulary Vocabulary
{
get
{
return DefaultVocabulary;
}
}
>>
dumpActions(recog, argFuncs, actionFuncs, sempredFuncs) ::= <<
public override void Action(RuleContext _localctx, int ruleIndex, int actionIndex) {
switch (ruleIndex) {
: _action(()_localctx, actionIndex); break;}; separator="\n">
}
}
public override bool Sempred(RuleContext _localctx, int ruleIndex, int predIndex) {
switch (ruleIndex) {
: return _sempred(()_localctx, predIndex);}; separator="\n">
}
return true;
}
>>
parser_ctor(parser) ::= <<
public (ITokenStream input) : this(input, Console.Out, Console.Error) { }
public (ITokenStream input, TextWriter output, TextWriter errorOutput)
: base(input, output, errorOutput)
{
Interpreter = new ParserATNSimulator(this, _ATN, decisionToDFA, sharedContextCache);
}
>>
/* This generates a private method since the actionIndex is generated, making an
* overriding implementation impossible to maintain.
*/
RuleActionFunction(r, actions) ::= <<
private void _action( _localctx, int actionIndex) {
switch (actionIndex) {
: break;}; separator="\n">
}
}
>>
/* This generates a private method since the predIndex is generated, making an
* overriding implementation impossible to maintain.
*/
RuleSempredFunction(r, actions) ::= <<
private bool _sempred( _localctx, int predIndex) {
switch (predIndex) {
: return ;}; separator="\n">
}
return true;
}
>>
RuleFunction(currentRule,args,code,locals,ruleCtx,altLabelCtxs,namedActions,finallyAction,postamble,exceptions) ::= <<
}; separator="\n">
[RuleVersion()]
}>public () {
_localctx = new (Context, State}>);
EnterRule(_localctx, , RULE_);
try {
int _alt;
}
catch (RecognitionException re) {
_localctx.exception = re;
ErrorHandler.ReportError(this, re);
ErrorHandler.Recover(this, re);
}
finally {
ExitRule();
}
return _localctx;
}
>>
LeftFactoredRuleFunction(currentRule,args,code,locals,namedActions,finallyAction,postamble) ::=
<<
}>private () {
_localctx = new (Context, State}>);
EnterLeftFactoredRule(_localctx, , RULE_);
try {
int _alt;
}
catch (RecognitionException re) {
_localctx.exception = re;
ErrorHandler.ReportError(this, re);
ErrorHandler.Recover(this, re);
}
finally {
ExitRule();
}
return _localctx;
}
>>
// This behaves similar to RuleFunction (enterRule is called, and no adjustments
// are made to the parse tree), but since it's still a variant no context class
// needs to be generated.
LeftUnfactoredRuleFunction(currentRule,args,code,locals,namedActions,finallyAction,postamble) ::=
<<
}>private () {
_localctx = new (Context, State}>);
EnterRule(_localctx, , RULE_);
try {
int _alt;
}
catch (RecognitionException re) {
_localctx.exception = re;
ErrorHandler.ReportError(this, re);
ErrorHandler.Recover(this, re);
}
finally {
ExitRule();
}
return _localctx;
}
>>
LeftRecursiveRuleFunction(currentRule,args,code,locals,ruleCtx,altLabelCtxs,
namedActions,finallyAction,postamble) ::=
<<
}; separator="\n">
[RuleVersion()]
}>public () {
return (0}>);
}
private (int _p}>) {
ParserRuleContext _parentctx = Context;
int _parentState = State;
_localctx = new (Context, _parentState}>);
_prevctx = _localctx;
int _startState = ;
EnterRecursionRule(_localctx, , RULE_, _p);
try {
int _alt;
}
catch (RecognitionException re) {
_localctx.exception = re;
ErrorHandler.ReportError(this, re);
ErrorHandler.Recover(this, re);
}
finally {
UnrollRecursionContexts(_parentctx);
}
return _localctx;
}
>>
CodeBlockForOuterMostAlt(currentOuterMostAltCodeBlock, locals, preamble, ops) ::= <<
_localctx = new Context(_localctx);
EnterOuterAlt(_localctx, );
>>
CodeBlockForAlt(currentAltCodeBlock, locals, preamble, ops) ::= <<
{