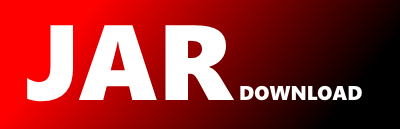
org.antlr.v4.tool.templates.codegen.Go.Go.stg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of virtdata-lib-realer Show documentation
Show all versions of virtdata-lib-realer Show documentation
With inspiration from other libraries
fileHeader(grammarFileName, ANTLRVersion) ::= <<
// Code generated from by ANTLR . DO NOT EDIT.
>>
ParserFile(file, parser, namedActions, contextSuperClass) ::= <<
package //
package parser //
import (
"fmt"
"reflect"
"strconv"
"github.com/antlr/antlr4/runtime/Go/antlr"
)
// Suppress unused import errors
var _ = fmt.Printf
var _ = reflect.Copy
var _ = strconv.Itoa
>>
ListenerFile(file, header, namedActions) ::= <<
package //
package parser //
import "github.com/antlr/antlr4/runtime/Go/antlr"
// Listener is a complete listener for a parse tree produced by .
type Listener interface {
antlr.ParseTreeListener
is called when entering the production.
Enter(c *Context)}; separator="\n\n">
is called when exiting the production.
Exit(c *Context)}; separator="\n\n">
}
>>
BaseListenerFile(file, header, namedActions) ::= <<
package //
package parser //
import "github.com/antlr/antlr4/runtime/Go/antlr"
// BaseListener is a complete listener for a parse tree produced by .
type BaseListener struct{}
var _ Listener = &BaseListener{}
// VisitTerminal is called when a terminal node is visited.
func (s *BaseListener) VisitTerminal(node antlr.TerminalNode) {}
// VisitErrorNode is called when an error node is visited.
func (s *BaseListener) VisitErrorNode(node antlr.ErrorNode) {}
// EnterEveryRule is called when any rule is entered.
func (s *BaseListener) EnterEveryRule(ctx antlr.ParserRuleContext) {}
// ExitEveryRule is called when any rule is exited.
func (s *BaseListener) ExitEveryRule(ctx antlr.ParserRuleContext) {}
is called when production is entered.
func (s *BaseListener) Enter(ctx *Context) {\}
// Exit is called when production is exited.
func (s *BaseListener) Exit(ctx *Context) {\}}; separator="\n\n">
>>
VisitorFile(file, header, namedActions) ::= <<
package //
package parser //
import "github.com/antlr/antlr4/runtime/Go/antlr"
// A complete Visitor for a parse tree produced by .
type Visitor interface {
antlr.ParseTreeVisitor
#.
Visit(ctx *Context) interface{\}
}; separator="\n">
}
>>
BaseVisitorFile(file, header, namedActions) ::= <<
package //
package parser //
import "github.com/antlr/antlr4/runtime/Go/antlr"
type BaseVisitor struct {
*antlr.BaseParseTreeVisitor
}
Visitor) Visit(ctx *Context) interface{\} {
return v.VisitChildren(ctx)
\}}; separator="\n\n">
>>
Parser(parser, funcs, atn, sempredFuncs, superClass) ::= <<
var parserATN =
var parserATN []uint16
var deserializer = antlr.NewATNDeserializer(nil)
var deserializedATN = deserializer.DeserializeFromUInt16(parserATN)
var literalNames = []string{
,
}
var literalNames []string
var symbolicNames = []string{
,
}
var symbolicNames []string
var ruleNames = []string{
"}; separator=", ", wrap>,
}
var ruleNames []string
var decisionToDFA = make([]*antlr.DFA, len(deserializedATN.DecisionToState))
func init() {
for index, ds := range deserializedATN.DecisionToState {
decisionToDFA[index] = antlr.NewDFA(ds, index)
}
}
type struct {
}
func New(input antlr.TokenStream) * {
this := new()
this.BaseParser = antlr.NewBaseParser(input)
this.Interpreter = antlr.NewParserATNSimulator(this, deserializedATN, decisionToDFA, antlr.NewPredictionContextCache())
this.RuleNames = ruleNames
this.LiteralNames = literalNames
this.SymbolicNames = symbolicNames
this.GrammarFileName = ""
return this
}
// tokens.
const (
EOF = antlr.TokenEOF
= }; separator="\n">
)
// EOF is the token.
const EOF = antlr.TokenEOF
// rules.
const (
RULE_ = }; separator="\n">
)
// RULE_ is the rule.
const RULE_ =
func (p *) Sempred(localctx antlr.RuleContext, ruleIndex, predIndex int) bool {
switch ruleIndex {
:
var t *Context = nil
if localctx != nil { t = localctx.(*Context) \}
return p._Sempred(t, predIndex)}; separator="\n\n">
default:
panic("No predicate with index: " + fmt.Sprint(ruleIndex))
}
}
>>
dumpActions(recog, argFuncs, actionFuncs, sempredFuncs) ::= <<
func (l *) Action(localctx antlr.RuleContext, ruleIndex, actionIndex int) {
switch ruleIndex {
:
l._Action(localctx, actionIndex)
var t *Context = nil
if localctx != nil { t = localctx.(*) \}
l._Action(t, actionIndex)
}; separator="\n\n">
default:
panic("No registered action for: " + fmt.Sprint(ruleIndex))
}
}
func (l *) Sempred(localctx antlr.RuleContext, ruleIndex, predIndex int) bool {
switch ruleIndex {
:
return l._Sempred(localctx, predIndex)
var t *Context = nil
if localctx != nil { t = localctx.(*) \}
return l._Sempred(t, predIndex)
}; separator="\n\n">
default:
panic("No registered predicate for: " + fmt.Sprint(ruleIndex))
}
}
>>
/* This generates a private method since the actionIndex is generated, making an
* overriding implementation impossible to maintain.
*/
RuleActionFunction(r, actions) ::= <<
func (l *) _Action(localctx antlr.RuleContext*, actionIndex int) {
switch actionIndex {
:
}; separator="\n\n">
default:
panic("No registered action for: " + fmt.Sprint(actionIndex))
}
}
>>
/* This generates a private method since the predIndex is generated, making an
* overriding implementation impossible to maintain.
*/
RuleSempredFunction(r, actions) ::= <<
func (p *) _Sempred(localctx antlr.RuleContext, predIndex int) bool {
switch predIndex {
:
return }; separator="\n\n">
default:
panic("No predicate with index: " + fmt.Sprint(predIndex))
}
}
>>
RuleFunction(currentRule, args, code, locals, ruleCtx, altLabelCtxs, namedActions, finallyAction, postamble, exceptions) ::= <<
}; separator="\n\n">
func (p *) ( }; separator=", ">) (localctx I) {
localctx = New(p, p.GetParserRuleContext(), p.GetState()}>)
p.EnterRule(localctx, , RULE_)
}; separator="\n">
defer func() {
p.ExitRule()
}()
defer func() {
if err := recover(); err != nil {
if v, ok := err.(antlr.RecognitionException); ok {
localctx.SetException(v)
p.GetErrorHandler().ReportError(p, v)
p.GetErrorHandler().Recover(p, v)
} else {
panic(err)
}
}
}()
var _alt int
return localctx
}
>>
LeftRecursiveRuleFunction(currentRule, args, code, locals, ruleCtx, altLabelCtxs, namedActions, finallyAction, postamble) ::= <<
}; separator="\n\n">
func (p *) ( }; separator=", ">) (localctx I