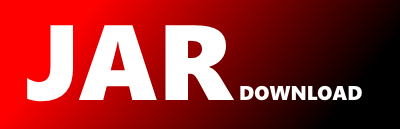
org.antlr.v4.tool.templates.codegen.JavaScript.JavaScript.stg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of virtdata-lib-realer Show documentation
Show all versions of virtdata-lib-realer Show documentation
With inspiration from other libraries
/*
* [The "BSD license"]
* Copyright (c) 2012-2016 Terence Parr
* Copyright (c) 2012-2016 Sam Harwell
* Copyright (c) 2014 Eric Vergnaud
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
/** ANTLR tool checks output templates are compatible with tool code generation.
* For now, a simple string match used on x.y of x.y.z scheme.
* Must match Tool.VERSION during load to templates.
*
* REQUIRED.
*/
javascriptTypeInitMap ::= [
"bool":"false",
"int":"0",
"float":"0.0",
"str":"",
default:"{}" // anything other than a primitive type is an object
]
// args must be ,
ParserFile(file, parser, namedActions, contextSuperClass) ::= <<
var antlr4 = require('antlr4/index');
var Listener = require('./Listener').Listener;
var Visitor = require('./Visitor').Visitor;
>>
ListenerFile(file, header, namedActions) ::= <<
var antlr4 = require('antlr4/index');
// This class defines a complete listener for a parse tree produced by .
function Listener() {
antlr4.tree.ParseTreeListener.call(this);
return this;
}
Listener.prototype = Object.create(antlr4.tree.ParseTreeListener.prototype);
Listener.prototype.constructor = Listener;
#.
Listener.prototype.enter = function(ctx) {
\};
// Exit a parse tree produced by #.
Listener.prototype.exit = function(ctx) {
\};
}; separator="\n">
exports.Listener = Listener;
>>
VisitorFile(file, header, namedActions) ::= <<
var antlr4 = require('antlr4/index');
// This class defines a complete generic visitor for a parse tree produced by .
function Visitor() {
antlr4.tree.ParseTreeVisitor.call(this);
return this;
}
Visitor.prototype = Object.create(antlr4.tree.ParseTreeVisitor.prototype);
Visitor.prototype.constructor = Visitor;
#.
Visitor.prototype.visit = function(ctx) {
return this.visitChildren(ctx);
\};
}; separator="\n">
exports.Visitor = Visitor;
>>
fileHeader(grammarFileName, ANTLRVersion) ::= <<
// Generated from by ANTLR
// jshint ignore: start
>>
Parser(parser, funcs, atn, sempredFuncs, superClass) ::= <<
var = require('./').;
var grammarFileName = "";
var atn = new antlr4.atn.ATNDeserializer().deserialize(serializedATN);
var decisionsToDFA = atn.decisionToState.map( function(ds, index) { return new antlr4.dfa.DFA(ds, index); });
var sharedContextCache = new antlr4.PredictionContextCache();
var literalNames = [ }; null="null", separator=", ", wrap, anchor> ];
var symbolicNames = [ }; null="null", separator=", ", wrap, anchor> ];
var ruleNames = [ "}; separator=", ", wrap, anchor> ];
function (input) {
.call(this, input);
this._interp = new antlr4.atn.ParserATNSimulator(this, atn, decisionsToDFA, sharedContextCache);
this.ruleNames = ruleNames;
this.literalNames = literalNames;
this.symbolicNames = symbolicNames;
return this;
}
.prototype = Object.create(.prototype);
.prototype.constructor = ;
Object.defineProperty(.prototype, "atn", {
get : function() {
return atn;
}
});
.EOF = antlr4.Token.EOF;
. = ;}; separator="\n", wrap, anchor>
.RULE_ = ;}; separator="\n", wrap, anchor>
.prototype.sempred = function(localctx, ruleIndex, predIndex) {
switch(ruleIndex) {
:
return this._sempred(localctx, predIndex);}; separator="\n">
default:
throw "No predicate with index:" + ruleIndex;
}
};
exports. = ;
>>
dumpActions(recog, argFuncs, actionFuncs, sempredFuncs) ::= <<
.prototype.action = function(localctx, ruleIndex, actionIndex) {
switch (ruleIndex) {
:
this._action(localctx, actionIndex);
break;}; separator="\n">
default:
throw "No registered action for:" + ruleIndex;
}
};
.prototype.sempred = function(localctx, ruleIndex, predIndex) {
switch (ruleIndex) {
:
return this._sempred(localctx, predIndex);}; separator="\n">
default:
throw "No registered predicate for:" + ruleIndex;
}
};
>>
/* This generates a private method since the actionIndex is generated, making an
* overriding implementation impossible to maintain.
*/
RuleActionFunction(r, actions) ::= <<
.prototype._action = function(localctx , actionIndex) {
switch (actionIndex) {
:
break;}; separator="\n">
default:
throw "No registered action for:" + actionIndex;
}
};
>>
/* This generates a private method since the predIndex is generated, making an
* overriding implementation impossible to maintain.
*/
RuleSempredFunction(r, actions) ::= <<
.prototype._sempred = function(localctx, predIndex) {
switch(predIndex) {
:
return ;}; separator="\n">
default:
throw "No predicate with index:" + predIndex;
}
};
>>
RuleFunction(currentRule,args,code,locals,ruleCtx,altLabelCtxs,namedActions,finallyAction,postamble,exceptions) ::= <<
}; separator="\n">
. = ;
.prototype. = function(}; separator=", ">) {
var localctx = new (this, this._ctx, this.state}>);
this.enterRule(localctx, , .RULE_);
try {
} catch (re) {
if(re instanceof antlr4.error.RecognitionException) {
localctx.exception = re;
this._errHandler.reportError(this, re);
this._errHandler.recover(this, re);
} else {
throw re;
}
} finally {
this.exitRule();
}
return localctx;
};
>>
LeftRecursiveRuleFunction(currentRule,args,code,locals,ruleCtx,altLabelCtxs,
namedActions,finallyAction,postamble) ::=
<<
}; separator="\n">
.prototype. = function(_p, }>) {
if(_p===undefined) {
_p = 0;
}
var _parentctx = this._ctx;
var _parentState = this.state;
var localctx = new (this, this._ctx, _parentState}>);
var _prevctx = localctx;
var _startState = ;
this.enterRecursionRule(localctx, , .RULE_, _p);
try {
} catch( error) {
if(error instanceof antlr4.error.RecognitionException) {
localctx.exception = error;
this._errHandler.reportError(this, error);
this._errHandler.recover(this, error);
} else {
throw error;
}
} finally {
this.unrollRecursionContexts(_parentctx)
}
return localctx;
};
>>
CodeBlockForOuterMostAlt(currentOuterMostAltCodeBlock, locals, preamble, ops) ::= <<
localctx = new Context(this, localctx);
this.enterOuterAlt(localctx, );
>>
CodeBlockForAlt(currentAltCodeBlock, locals, preamble, ops) ::= <<
>>
LL1AltBlock(choice, preamble, alts, error) ::= <<
this.state = ;
this._errHandler.sync(this);
= this._input.LT(1);
switch(this._input.LA(1)) {
break;}; separator="\n">
default:
}
>>
LL1OptionalBlock(choice, alts, error) ::= <<
this.state = ;
this._errHandler.sync(this);
switch (this._input.LA(1)) {
break;}; separator="\n">
default:
break;
}
>>
LL1OptionalBlockSingleAlt(choice, expr, alts, preamble, error, followExpr) ::= <<
this.state = ;
this._errHandler.sync(this);
if() {
}
) ) !>
>>
LL1StarBlockSingleAlt(choice, loopExpr, alts, preamble, iteration) ::= <<
this.state = ;
this._errHandler.sync(this);
while() {
this.state = ;
this._errHandler.sync(this);
}
>>
LL1PlusBlockSingleAlt(choice, loopExpr, alts, preamble, iteration) ::= <<
this.state = ;
this._errHandler.sync(this);
do {
this.state = ;
this._errHandler.sync(this);
} while();
>>
// LL(*) stuff
AltBlock(choice, preamble, alts, error) ::= <<
this.state = ;
this._errHandler.sync(this);
= _input.LT(1)
var la_ = this._interp.adaptivePredict(this._input,,this._ctx);
switch(la_) {
:
break;
}; separator="\n">
}
>>
OptionalBlock(choice, alts, error) ::= <<
this.state = ;
this._errHandler.sync(this);
var la_ = this._interp.adaptivePredict(this._input,,this._ctx);
+1) {
}; separator="\n} else ">
}
>>
StarBlock(choice, alts, sync, iteration) ::= <<
this.state = ;
this._errHandler.sync(this);
var _alt = this._interp.adaptivePredict(this._input,,this._ctx)
while(_alt!= && _alt!=antlr4.atn.ATN.INVALID_ALT_NUMBER) {
if(_alt===1+1) {
}
this.state = ;
this._errHandler.sync(this);
_alt = this._interp.adaptivePredict(this._input,,this._ctx);
}
>>
PlusBlock(choice, alts, error) ::= <<
this.state = ;
this._errHandler.sync(this);
var _alt = 1+1;
do {
switch (_alt) {
+1:
break;}; separator="\n">
default:
}
this.state = ;
this._errHandler.sync(this);
_alt = this._interp.adaptivePredict(this._input,, this._ctx);
} while ( _alt!= && _alt!=antlr4.atn.ATN.INVALID_ALT_NUMBER );
>>
Sync(s) ::= "sync()"
ThrowNoViableAlt(t) ::= "throw new antlr4.error.NoViableAltException(this);"
TestSetInline(s) ::= <<
}; separator=" || ">
>>
// Javascript language spec - shift operators are 32 bits long max
testShiftInRange(shiftAmount) ::= <<
(() & ~0x1f) == 0
>>
// produces smaller bytecode only when bits.ttypes contains more than two items
bitsetBitfieldComparison(s, bits) ::= <%
(})> && ((1 \<\< ) & ()}; separator=" | ">)) !== 0)
%>
isZero ::= [
"0":true,
default:false
]
offsetShiftVar(shiftAmount, offset) ::= <%
( - )
%>
offsetShiftType(shiftAmount, offset) ::= <%
(. - ).
%>
// produces more efficient bytecode when bits.ttypes contains at most two items
bitsetInlineComparison(s, bits) ::= <%
===.}; separator=" || ">
%>
cases(ttypes) ::= <<
.:}; separator="\n">
>>
InvokeRule(r, argExprsChunks) ::= <<
this.state = ;
= }>this.