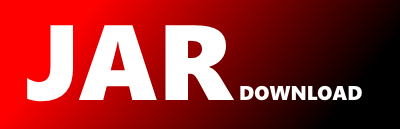
org.antlr.v4.tool.templates.codegen.Cpp.Cpp.stg Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of virtdata-lib-realer Show documentation
Show all versions of virtdata-lib-realer Show documentation
With inspiration from other libraries
/*
* [The "BSD license"]
* Copyright (c) 2015 Dan McLaughlin, Mike Lischke
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* 1. Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* 2. Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* 3. The name of the author may not be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR
* IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES
* OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED.
* IN NO EVENT SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT,
* INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT
* NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE,
* DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY
* THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF
* THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
import "Files.stg" // All file specific stuff.
cppTypeInitMap ::= [
"int":"0",
"long":"0",
"float":"0.0f",
"double":"0.0",
"bool":"false",
"short":"0",
"char":"0",
default: "nullptr" // anything other than a primitive type is an object
]
LexerHeader(lexer, atn, actionFuncs, sempredFuncs, superClass = {antlr4::Lexer}) ::= <<
class : public {
public:
enum {
= }; separator=", ", wrap, anchor>
};
enum {
= }; separator=", ", wrap, anchor>
};
enum {
= }; separator=", ", wrap, anchor>
};
(antlr4::CharStream *input);
~();
virtual std::string getGrammarFileName() const override;
virtual const std::vector\& getRuleNames() const override;
virtual const std::vector\& getChannelNames() const override;
virtual const std::vector\& getModeNames() const override;
virtual const std::vector\& getTokenNames() const override; // deprecated, use vocabulary instead
virtual antlr4::dfa::Vocabulary& getVocabulary() const override;
virtual const std::vector\ getSerializedATN() const override;
virtual const antlr4::atn::ATN& getATN() const override;
virtual void action(antlr4::RuleContext *context, size_t ruleIndex, size_t actionIndex) override;
virtual bool sempred(antlr4::RuleContext *_localctx, size_t ruleIndex, size_t predicateIndex) override;
private:
static std::vector\ _decisionToDFA;
static antlr4::atn::PredictionContextCache _sharedContextCache;
static std::vector\ _ruleNames;
static std::vector\ _tokenNames;
static std::vector\ _channelNames;
static std::vector\ _modeNames;
static std::vector\ _literalNames;
static std::vector\ _symbolicNames;
static antlr4::dfa::Vocabulary _vocabulary;
// Individual action functions triggered by action() above.
// Individual semantic predicate functions triggered by sempred() above.
struct Initializer {
Initializer();
};
static Initializer _init;
};
>>
Lexer(lexer, atn, actionFuncs, sempredFuncs, superClass = {Lexer}) ::= <<
::(CharStream *input) : (input) {
_interpreter = new atn::LexerATNSimulator(this, _atn, _decisionToDFA, _sharedContextCache);
}
::~() {
delete _interpreter;
}
std::string ::getGrammarFileName() const {
return "";
}
const std::vector\& ::getRuleNames() const {
return _ruleNames;
}
const std::vector\& ::getChannelNames() const {
return _channelNames;
}
const std::vector\& ::getModeNames() const {
return _modeNames;
}
const std::vector\& ::getTokenNames() const {
return _tokenNames;
}
dfa::Vocabulary& ::getVocabulary() const {
return _vocabulary;
}
const std::vector\ ::getSerializedATN() const {
return _serializedATN;
}
const atn::ATN& ::getATN() const {
return _atn;
}
void ::action(RuleContext *context, size_t ruleIndex, size_t actionIndex) {
switch (ruleIndex) {
: Action(dynamic_cast\< *>(context), actionIndex); break;}; separator="\n">
default:
break;
}
}
bool ::sempred(RuleContext *context, size_t ruleIndex, size_t predicateIndex) {
switch (ruleIndex) {
: return Sempred(dynamic_cast\< *>(context), predicateIndex);}; separator="\n">
default:
break;
}
return true;
}
// Static vars and initialization.
std::vector\ ::_decisionToDFA;
atn::PredictionContextCache ::_sharedContextCache;
// We own the ATN which in turn owns the ATN states.
atn::ATN ::_atn;
std::vector\ ::_serializedATN;
std::vector\ ::_ruleNames = {
"}; separator = ", ", wrap, anchor>
};
std::vector\ ::_channelNames = {
"DEFAULT_TOKEN_CHANNEL", "HIDDEN", "}; separator = ", ", wrap, anchor>
};
std::vector\ ::_modeNames = {
"}; separator = ", ", wrap, anchor>
};
std::vector\ ::_literalNames = {
}; null = "\"\"", separator = ", ", wrap, anchor>
};
std::vector\ ::_symbolicNames = {
}; null = "\"\"", separator = ", ", wrap, anchor>
};
dfa::Vocabulary ::_vocabulary(_literalNames, _symbolicNames);
std::vector\ ::_tokenNames;
::Initializer::Initializer() {
// This code could be in a static initializer lambda, but VS doesn't allow access to private class members from there.
for (size_t i = 0; i \< _symbolicNames.size(); ++i) {
std::string name = _vocabulary.getLiteralName(i);
if (name.empty()) {
name = _vocabulary.getSymbolicName(i);
}
if (name.empty()) {
_tokenNames.push_back("\");
} else {
_tokenNames.push_back(name);
}
}
}
::Initializer ::_init;
>>
RuleActionFunctionHeader(r, actions) ::= <<
void Action( *context, size_t actionIndex);
>>
RuleActionFunction(r, actions) ::= <<
void ::Action( *context, size_t actionIndex) {
switch (actionIndex) {
: break;}; separator="\n">
default:
break;
}
}
>>
RuleSempredFunctionHeader(r, actions) ::= <<
bool Sempred( *_localctx, size_t predicateIndex);
>>
RuleSempredFunction(r, actions) ::= <<
bool ::Sempred( *_localctx, size_t predicateIndex) {
switch (predicateIndex) {
: return }; separator=";\n">;
default:
break;
}
return true;
}
>>
//--------------------------------------------------------------------------------------------------
ParserHeader(parser, funcs, atn, sempredFuncs, superClass = {antlr4::Parser}) ::= <<
class : public {
public:
enum {
= }; separator=", ", wrap, anchor>
};
enum {
= }; separator=", ", wrap, anchor>
};
(antlr4::TokenStream *input);
~();
virtual std::string getGrammarFileName() const override;
virtual const antlr4::atn::ATN& getATN() const override { return _atn; };
virtual const std::vector\& getTokenNames() const override { return _tokenNames; }; // deprecated: use vocabulary instead.
virtual const std::vector\& getRuleNames() const override;
virtual antlr4::dfa::Vocabulary& getVocabulary() const override;
Context;}; separator = "\n">
virtual bool sempred(antlr4::RuleContext *_localctx, size_t ruleIndex, size_t predicateIndex) override;
private:
static std::vector\ _decisionToDFA;
static antlr4::atn::PredictionContextCache _sharedContextCache;
static std::vector\ _ruleNames;
static std::vector\ _tokenNames;
static std::vector\ _literalNames;
static std::vector\ _symbolicNames;
static antlr4::dfa::Vocabulary _vocabulary;
struct Initializer {
Initializer();
};
static Initializer _init;
};
>>
Parser(parser, funcs, atn, sempredFuncs, superClass = {Parser}) ::= <<
using namespace antlr4;
::(TokenStream *input) : (input) {
_interpreter = new atn::ParserATNSimulator(this, _atn, _decisionToDFA, _sharedContextCache);
}
::~() {
delete _interpreter;
}
std::string ::getGrammarFileName() const {
return "";
}
const std::vector\& ::getRuleNames() const {
return _ruleNames;
}
dfa::Vocabulary& ::getVocabulary() const {
return _vocabulary;
}
bool ::sempred(RuleContext *context, size_t ruleIndex, size_t predicateIndex) {
switch (ruleIndex) {
: return Sempred(dynamic_cast\< *>(context), predicateIndex);}; separator="\n">
default:
break;
}
return true;
}
// Static vars and initialization.
std::vector\ ::_decisionToDFA;
atn::PredictionContextCache ::_sharedContextCache;
// We own the ATN which in turn owns the ATN states.
atn::ATN ::_atn;
std::vector\ ::_serializedATN;
std::vector\ ::_ruleNames = {
"}; separator = ", ", wrap, anchor>
};
std::vector\ ::_literalNames = {
}; null = "\"\"", separator = ", ", wrap, anchor>
};
std::vector\ ::_symbolicNames = {
}; null = "\"\"", separator = ", ", wrap, anchor>
};
dfa::Vocabulary ::_vocabulary(_literalNames, _symbolicNames);
std::vector\ ::_tokenNames;
::Initializer::Initializer() {
for (size_t i = 0; i \< _symbolicNames.size(); ++i) {
std::string name = _vocabulary.getLiteralName(i);
if (name.empty()) {
name = _vocabulary.getSymbolicName(i);
}
if (name.empty()) {
_tokenNames.push_back("\");
} else {
_tokenNames.push_back(name);
}
}
}
::Initializer ::_init;
>>
SerializedATNHeader(model) ::= <<
static antlr4::atn::ATN _atn;
static std::vector\ _serializedATN;
>>
// Constructs the serialized ATN and writes init code for static member vars.
SerializedATN(model) ::= <<
[] = {
}>
\};}; separator="\n">
,
serializedATNSegment + sizeof(serializedATNSegment) / sizeof(serializedATNSegment[0]));
}>
_serializedATN = {
}>
};
atn::ATNDeserializer deserializer;
_atn = deserializer.deserialize(_serializedATN);
size_t count = _atn.getNumberOfDecisions();
_decisionToDFA.reserve(count);
for (size_t i = 0; i \< count; i++) {
_decisionToDFA.emplace_back(_atn.getDecisionState(i), i);
}
>>
RuleFunctionHeader(currentRule, args, code, locals, ruleCtx, altLabelCtxs, namedActions, finallyAction, postamble, exceptions) ::= <<
}; separator="\n">
* ();
>>
RuleFunction(currentRule, args, code, locals, ruleCtx, altLabelCtxs, namedActions, finallyAction, postamble, exceptions) ::= <<
}; separator = "\n">
::* ::() {
*_localctx = _tracker.createInstance\<\>(_ctx, getState()}>);
enterRule(_localctx, , ::Rule);
auto onExit = finally([=] {
exitRule();
});
try {
size_t alt;
}
catch (RecognitionException &e) {
_errHandler->reportError(this, e);
_localctx->exception = std::current_exception();
_errHandler->recover(this, _localctx->exception);
}
return _localctx;
}
>>
LeftRecursiveRuleFunctionHeader(currentRule, args, code, locals, ruleCtx, altLabelCtxs, namedActions, finallyAction, postamble) ::= <<
}; separator="\n">
* ();
* (int precedence}>);
>>
LeftRecursiveRuleFunction(currentRule, args, code, locals, ruleCtx, altLabelCtxs, namedActions, finallyAction, postamble) ::= <<
}; separator="\n">
::* ::(