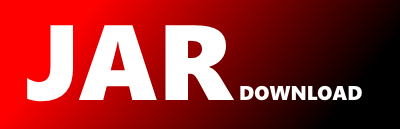
io.vlingo.lattice.grid.Grid Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vlingo-lattice Show documentation
Show all versions of vlingo-lattice Show documentation
Tooling for reactive Domain-Driven Design projects that are highly concurrent. Includes compute grid, actor caching, spaces, cross-node cluster messaging, CQRS, and Event Sourcing support.
// Copyright © 2012-2020 VLINGO LABS. All rights reserved.
//
// This Source Code Form is subject to the terms of the
// Mozilla Public License, v. 2.0. If a copy of the MPL
// was not distributed with this file, You can obtain
// one at https://mozilla.org/MPL/2.0/.
package io.vlingo.lattice.grid;
import java.util.Arrays;
import java.util.function.BiFunction;
import io.vlingo.actors.Actor;
import io.vlingo.actors.Address;
import io.vlingo.actors.AddressFactory;
import io.vlingo.actors.Configuration;
import io.vlingo.actors.Definition;
import io.vlingo.actors.Stage;
import io.vlingo.actors.World;
import io.vlingo.common.Completes;
import io.vlingo.common.identity.IdentityGeneratorType;
import io.vlingo.lattice.grid.cache.Cache;
import io.vlingo.lattice.grid.cache.CacheNodePoint;
import io.vlingo.lattice.grid.hashring.HashRing;
import io.vlingo.lattice.grid.hashring.HashedNodePoint;
import io.vlingo.lattice.grid.hashring.MurmurArrayHashRing;
public class Grid extends Stage {
private final Cache cache;
private final BiFunction> factory;
private final HashRing hashRing;
public static Grid startWith(final String worldName, final String gridNodeName) throws Exception {
mustNotExist();
final World world = World.startWithDefaults(worldName);
final AddressFactory addressFactory = new GridAddressFactory(IdentityGeneratorType.RANDOM);
final Grid grid = new Grid(world, addressFactory, gridNodeName);
GridNodeBootstrap.boot(world, grid, gridNodeName, false);
return grid;
}
public static Grid startWith(final String worldName, final java.util.Properties properties, final String gridNodeName) throws Exception {
mustNotExist();
final World world = World.start(worldName, properties);
final AddressFactory addressFactory = new GridAddressFactory(IdentityGeneratorType.RANDOM);
final Grid grid = new Grid(world, addressFactory, gridNodeName);
GridNodeBootstrap.boot(world, grid, gridNodeName, false);
return grid;
}
public static Grid startWith(final String worldName, final Configuration configuration, final String gridNodeName) throws Exception {
mustNotExist();
final World world = World.start(worldName, configuration);
final AddressFactory addressFactory = new GridAddressFactory(IdentityGeneratorType.RANDOM);
final Grid grid = new Grid(world, addressFactory, gridNodeName);
GridNodeBootstrap.boot(world, grid, gridNodeName, false);
return grid;
}
public static Grid startWith(final World world, final AddressFactory addressFactory, final String gridNodeName) throws Exception {
mustNotExist();
final Grid grid = new Grid(world, addressFactory, gridNodeName);
GridNodeBootstrap.boot(world, grid, gridNodeName, false);
return grid;
}
private static void mustNotExist() {
if (GridNodeBootstrap.exists()) {
throw new IllegalStateException("Grid already exists.");
}
}
public Grid(final World world, final AddressFactory addressFactory, final String gridNodeName) {
super(world, addressFactory, gridNodeName);
extenderStartDirectoryScanner();
this.cache = Cache.defaultCache();
this.factory = (hash, node) -> { return new CacheNodePoint(this.cache, hash, node); };
this.hashRing = new MurmurArrayHashRing<>(100, factory);
}
@Override
public T actorFor(final Class protocol, final Definition definition) {
return actorFor(protocol, definition, addressFactory().unique());
}
@Override
public T actorFor(final Class protocol, final Definition definition, final Address address) {
if (world().isTerminated()) {
throw new IllegalStateException("vlingo/lattice: Grid has stopped.");
}
if (!address.isDistributable()) {
throw new IllegalArgumentException("Address is not distributable.");
}
final T actor = super.actorFor(protocol, definition, address);
return actor;
}
@Override
public T actorFor(final Class protocol, final Class extends Actor> type, final Object...parameters) {
if (world().isTerminated()) {
throw new IllegalStateException("vlingo/lattice: Grid has stopped.");
}
final T actor = super.actorFor(protocol, Definition.has(type, Arrays.asList(parameters)), addressFactory().unique());
return actor;
}
@Override
public Completes actorOf(final Class protocol, final Address address) {
if (!address.isDistributable()) {
throw new IllegalArgumentException("Address is not distributable.");
}
return super.actorOf(protocol, address);
}
public void terminate() {
world().terminate();
}
HashRing hashRing() {
return hashRing;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy