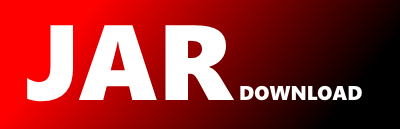
io.vlingo.lattice.grid.hashring.MurmurSortedMapHashRing Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vlingo-lattice Show documentation
Show all versions of vlingo-lattice Show documentation
Tooling for reactive Domain-Driven Design projects that are highly concurrent. Includes compute grid, actor caching, spaces, cross-node cluster messaging, CQRS, and Event Sourcing support.
package io.vlingo.lattice.grid.hashring;
import java.nio.ByteBuffer;
import java.util.SortedMap;
import java.util.TreeMap;
public class MurmurSortedMapHashRing implements HashRing {
private static final int DefaultSeed = 31;
private final int pointsPerNode;
private final int seed;
private final ByteBuffer buffer;
private final SortedMap ring;
public MurmurSortedMapHashRing(final int pointsPerNode) {
this(pointsPerNode, DefaultSeed);
}
public MurmurSortedMapHashRing(final int pointsPerNode, final int seed) {
this.pointsPerNode = pointsPerNode;
this.seed = seed;
this.buffer = ByteBuffer.allocate(64);
this.ring = new TreeMap<>();
}
@Override
public void dump() {
System.out.println("NODES: " + ring.size());
for (final T hashedNodePoint : ring.values()) {
System.out.println("NODE: " + hashedNodePoint);
}
}
@Override
public HashRing includeNode(T nodeIdentifier) {
for (int i = 0; i < pointsPerNode; i++) {
final int hash = hashed(nodeIdentifier.toString() + i);
ring.put(hash, nodeIdentifier);
}
return this;
}
private int hashed(final Object id) {
buffer.clear();
buffer.put(id.toString().getBytes());
return MurmurHash.hash32(buffer, 0, buffer.position(), seed);
}
@Override
public HashRing excludeNode(T nodeIdentifier) {
for (int i = 0; i < pointsPerNode; i++) {
final int hash = hashed(nodeIdentifier.toString() + i);
ring.remove(hash);
}
return this;
}
@Override
public T nodeOf(Object id) {
if (ring.isEmpty()) {
return null;
}
int hash = hashed(id);
if (!ring.containsKey(hash)) {
SortedMap tailMap =
ring.tailMap(hash);
hash = tailMap.isEmpty() ?
ring.firstKey() : tailMap.firstKey();
}
return ring.get(hash);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy