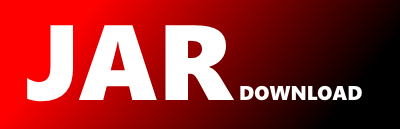
io.vlingo.lattice.model.projection.AbstractProjectionDispatcherActor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of vlingo-lattice Show documentation
Show all versions of vlingo-lattice Show documentation
Tooling for reactive Domain-Driven Design projects that are highly concurrent. Includes compute grid, actor caching, spaces, cross-node cluster messaging, CQRS, and Event Sourcing support.
// Copyright © 2012-2020 VLINGO LABS. All rights reserved.
//
// This Source Code Form is subject to the terms of the
// Mozilla Public License, v. 2.0. If a copy of the MPL
// was not distributed with this file, You can obtain
// one at https://mozilla.org/MPL/2.0/.
package io.vlingo.lattice.model.projection;
import java.util.Collection;
import java.util.List;
import io.vlingo.actors.Actor;
/**
* Abstract base of all {@code ProjectionDispatcher} types and that
* holds the pool of {@code Projection} instances that are used to
* project {@code Projectable} states based on {@code MatchableProjections}.
*/
public class AbstractProjectionDispatcherActor extends Actor implements ProjectionDispatcher {
private final MatchableProjections matchableProjections;
/**
* Construct my default state.
*/
protected AbstractProjectionDispatcherActor() {
this.matchableProjections = new MatchableProjections();
}
/**
* Construct my default state with {@code projectToDescriptions}.
* @param projectToDescriptions the {@code Collection} describing my matchable projections
*/
protected AbstractProjectionDispatcherActor(final Collection projectToDescriptions) {
this();
for (final ProjectToDescription discription : projectToDescriptions) {
final Projection projection;
if (discription.constructionParameter.isPresent()) {
projection = stage().actorFor(Projection.class, discription.projectionType, discription.constructionParameter.get());
} else {
projection = stage().actorFor(Projection.class, discription.projectionType);
}
projectTo(projection, discription.becauseOf);
}
}
//=====================================
// ProjectionDispatcher
//=====================================
/*
* @see io.vlingo.lattice.model.projection.ProjectionDispatcher#projectTo(io.vlingo.lattice.model.projection.Projection, java.lang.String[])
*/
@Override
public void projectTo(final Projection projection, final String[] whenMatchingCause) {
matchableProjections.mayDispatchTo(projection, whenMatchingCause);
}
//=====================================
// internal implementation
//=====================================
/**
* Answer whether or not I have any {@code Projection} that supports the {@code actualCause}.
* @param actualCause the String describing the cause that requires Projection
* @return boolean
*/
protected boolean hasProjectionsFor(final String actualCause) {
return !projectionsFor(actualCause).isEmpty();
}
/**
* Answer the {@code List} of my {@code Projection} that match {@code actualCause}.
* @param actualCauses the String... describing the cause that requires Projection
* @return {@code List}
*/
protected List projectionsFor(final String... actualCauses) {
return matchableProjections.matchProjections(actualCauses);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy