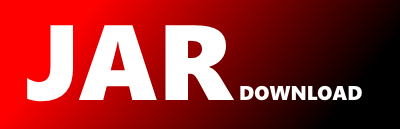
io.weaviate.client.v1.graphql.query.argument.NearImageArgument Maven / Gradle / Ivy
// Generated by delombok at Thu Jul 06 20:35:07 UTC 2023
package io.weaviate.client.v1.graphql.query.argument;
import io.weaviate.client.v1.graphql.query.util.Serializer;
import org.apache.commons.lang3.StringUtils;
import java.io.File;
import java.nio.file.Files;
import java.nio.file.Paths;
import java.util.Base64;
import java.util.LinkedHashSet;
import java.util.Set;
public class NearImageArgument implements Argument {
private final String image;
private final File imageFile;
private final Float certainty;
private final Float distance;
private String readFile(File file) {
try {
byte[] content = Files.readAllBytes(Paths.get(file.toURI()));
return Base64.getEncoder().encodeToString(content);
} catch (Exception e) {
return null;
}
}
private String getContent() {
if (StringUtils.isNotBlank(image)) {
if (image.startsWith("data:")) {
String base64 = ";base64,";
return image.substring(image.indexOf(base64) + base64.length());
}
return image;
}
if (imageFile != null) {
return readFile(imageFile);
}
return null;
}
@Override
public String build() {
Set fields = new LinkedHashSet<>();
String content = getContent();
if (StringUtils.isNotBlank(content)) {
fields.add(String.format("image:%s", Serializer.quote(content)));
}
if (certainty != null) {
fields.add(String.format("certainty:%s", certainty));
}
if (distance != null) {
fields.add(String.format("distance:%s", distance));
}
return String.format("nearImage:{%s}", String.join(" ", fields));
}
@java.lang.SuppressWarnings("all")
NearImageArgument(final String image, final File imageFile, final Float certainty, final Float distance) {
this.image = image;
this.imageFile = imageFile;
this.certainty = certainty;
this.distance = distance;
}
@java.lang.SuppressWarnings("all")
public static class NearImageArgumentBuilder {
@java.lang.SuppressWarnings("all")
private String image;
@java.lang.SuppressWarnings("all")
private File imageFile;
@java.lang.SuppressWarnings("all")
private Float certainty;
@java.lang.SuppressWarnings("all")
private Float distance;
@java.lang.SuppressWarnings("all")
NearImageArgumentBuilder() {
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public NearImageArgument.NearImageArgumentBuilder image(final String image) {
this.image = image;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public NearImageArgument.NearImageArgumentBuilder imageFile(final File imageFile) {
this.imageFile = imageFile;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public NearImageArgument.NearImageArgumentBuilder certainty(final Float certainty) {
this.certainty = certainty;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public NearImageArgument.NearImageArgumentBuilder distance(final Float distance) {
this.distance = distance;
return this;
}
@java.lang.SuppressWarnings("all")
public NearImageArgument build() {
return new NearImageArgument(this.image, this.imageFile, this.certainty, this.distance);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "NearImageArgument.NearImageArgumentBuilder(image=" + this.image + ", imageFile=" + this.imageFile + ", certainty=" + this.certainty + ", distance=" + this.distance + ")";
}
}
@java.lang.SuppressWarnings("all")
public static NearImageArgument.NearImageArgumentBuilder builder() {
return new NearImageArgument.NearImageArgumentBuilder();
}
@java.lang.SuppressWarnings("all")
public String getImage() {
return this.image;
}
@java.lang.SuppressWarnings("all")
public File getImageFile() {
return this.imageFile;
}
@java.lang.SuppressWarnings("all")
public Float getCertainty() {
return this.certainty;
}
@java.lang.SuppressWarnings("all")
public Float getDistance() {
return this.distance;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "NearImageArgument(image=" + this.getImage() + ", imageFile=" + this.getImageFile() + ", certainty=" + this.getCertainty() + ", distance=" + this.getDistance() + ")";
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof NearImageArgument)) return false;
final NearImageArgument other = (NearImageArgument) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$certainty = this.getCertainty();
final java.lang.Object other$certainty = other.getCertainty();
if (this$certainty == null ? other$certainty != null : !this$certainty.equals(other$certainty)) return false;
final java.lang.Object this$distance = this.getDistance();
final java.lang.Object other$distance = other.getDistance();
if (this$distance == null ? other$distance != null : !this$distance.equals(other$distance)) return false;
final java.lang.Object this$image = this.getImage();
final java.lang.Object other$image = other.getImage();
if (this$image == null ? other$image != null : !this$image.equals(other$image)) return false;
final java.lang.Object this$imageFile = this.getImageFile();
final java.lang.Object other$imageFile = other.getImageFile();
if (this$imageFile == null ? other$imageFile != null : !this$imageFile.equals(other$imageFile)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof NearImageArgument;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $certainty = this.getCertainty();
result = result * PRIME + ($certainty == null ? 43 : $certainty.hashCode());
final java.lang.Object $distance = this.getDistance();
result = result * PRIME + ($distance == null ? 43 : $distance.hashCode());
final java.lang.Object $image = this.getImage();
result = result * PRIME + ($image == null ? 43 : $image.hashCode());
final java.lang.Object $imageFile = this.getImageFile();
result = result * PRIME + ($imageFile == null ? 43 : $imageFile.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy