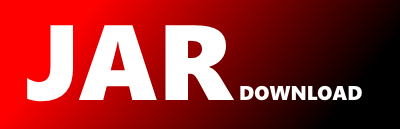
io.weaviate.client.v1.graphql.query.builder.GetBuilder Maven / Gradle / Ivy
// Generated by delombok at Thu Jul 06 20:35:08 UTC 2023
package io.weaviate.client.v1.graphql.query.builder;
import io.weaviate.client.v1.filters.WhereFilter;
import io.weaviate.client.v1.graphql.query.argument.AskArgument;
import io.weaviate.client.v1.graphql.query.argument.Bm25Argument;
import io.weaviate.client.v1.graphql.query.argument.GroupArgument;
import io.weaviate.client.v1.graphql.query.argument.GroupByArgument;
import io.weaviate.client.v1.graphql.query.argument.HybridArgument;
import io.weaviate.client.v1.graphql.query.argument.NearImageArgument;
import io.weaviate.client.v1.graphql.query.argument.NearObjectArgument;
import io.weaviate.client.v1.graphql.query.argument.NearTextArgument;
import io.weaviate.client.v1.graphql.query.argument.NearVectorArgument;
import io.weaviate.client.v1.graphql.query.argument.SortArguments;
import io.weaviate.client.v1.graphql.query.argument.WhereArgument;
import io.weaviate.client.v1.graphql.query.fields.Field;
import io.weaviate.client.v1.graphql.query.fields.Fields;
import io.weaviate.client.v1.graphql.query.fields.GenerativeSearchBuilder;
import io.weaviate.client.v1.graphql.query.util.Serializer;
import org.apache.commons.lang3.ObjectUtils;
import org.apache.commons.lang3.StringUtils;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.LinkedHashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import java.util.stream.Collectors;
import java.util.stream.Stream;
public class GetBuilder implements Query {
private final String className;
private final Fields fields;
private final Integer offset;
private final Integer limit;
private final String after;
private final Integer autocut;
private final String withConsistencyLevel;
private final WhereArgument withWhereFilter;
private final NearTextArgument withNearTextFilter;
private final Bm25Argument withBm25Filter;
private final HybridArgument withHybridFilter;
private final NearObjectArgument withNearObjectFilter;
private final AskArgument withAskArgument;
private final NearImageArgument withNearImageFilter;
private final NearVectorArgument withNearVectorFilter;
private final GroupArgument withGroupArgument;
private final SortArguments withSortArguments;
private final GenerativeSearchBuilder withGenerativeSearch;
private final GroupByArgument withGroupByArgument;
private final String tenant;
private boolean includesFilterClause() {
return ObjectUtils.anyNotNull(withWhereFilter, withNearTextFilter, withNearObjectFilter, withNearVectorFilter, withNearImageFilter, withGroupArgument, withAskArgument, withBm25Filter, withHybridFilter, limit, offset, withSortArguments, withGroupByArgument, autocut) || !StringUtils.isAllBlank(withConsistencyLevel, after, tenant);
}
private String createFilterClause() {
if (includesFilterClause()) {
Set filters = new LinkedHashSet<>();
if (StringUtils.isNotBlank(tenant)) {
filters.add(String.format("tenant:%s", Serializer.quote(tenant)));
}
if (withWhereFilter != null) {
filters.add(withWhereFilter.build());
}
if (withNearTextFilter != null) {
filters.add(withNearTextFilter.build());
}
if (withBm25Filter != null) {
filters.add(withBm25Filter.build());
}
if (withHybridFilter != null) {
filters.add(withHybridFilter.build());
}
if (withNearObjectFilter != null) {
filters.add(withNearObjectFilter.build());
}
if (withNearVectorFilter != null) {
filters.add(withNearVectorFilter.build());
}
if (withGroupArgument != null) {
filters.add(withGroupArgument.build());
}
if (withAskArgument != null) {
filters.add(withAskArgument.build());
}
if (withNearImageFilter != null) {
filters.add(withNearImageFilter.build());
}
if (limit != null) {
filters.add(String.format("limit:%s", limit));
}
if (offset != null) {
filters.add(String.format("offset:%s", offset));
}
if (StringUtils.isNotBlank(after)) {
filters.add(String.format("after:%s", Serializer.quote(after)));
}
if (withSortArguments != null) {
filters.add(withSortArguments.build());
}
if (StringUtils.isNotBlank(withConsistencyLevel)) {
filters.add(String.format("consistencyLevel:%s", Serializer.escape(withConsistencyLevel)));
}
if (withGroupByArgument != null) {
filters.add(withGroupByArgument.build());
}
if (autocut != null) {
filters.add(String.format("autocut:%s", autocut));
}
return String.format("(%s)", String.join(" ", filters));
}
return "";
}
private String createFields() {
if (ObjectUtils.allNull(fields, withGenerativeSearch)) {
return "";
}
if (withGenerativeSearch == null) {
return fields.build();
}
Field generate = withGenerativeSearch.build();
Field generateAdditional = Field.builder().name("_additional").fields(new Field[] {generate}).build();
if (fields == null) {
return generateAdditional.build();
}
// check if _additional field exists. If missing just add new _additional with generate,
// if exists merge generate into present one
Map> grouped = Arrays.stream(fields.getFields()).collect(Collectors.groupingBy(f -> "_additional".equals(f.getName())));
List additionals = grouped.getOrDefault(true, new ArrayList<>());
if (additionals.isEmpty()) {
additionals.add(generateAdditional);
} else {
Field[] mergedInternalFields = Stream.concat(Arrays.stream(additionals.get(0).getFields()), Stream.of(generate)).toArray(Field[]::new);
additionals.set(0, Field.builder().name("_additional").fields(mergedInternalFields).build());
}
Field[] allFields = Stream.concat(grouped.getOrDefault(false, new ArrayList<>()).stream(), additionals.stream()).toArray(Field[]::new);
return Fields.builder().fields(allFields).build().build();
}
@Override
public String buildQuery() {
return String.format("{Get{%s%s{%s}}}", Serializer.escape(className), createFilterClause(), createFields());
}
// created to support both types of setters: WhereArgument and deprecated WhereFilter
public static class GetBuilderBuilder {
@java.lang.SuppressWarnings("all")
private String className;
@java.lang.SuppressWarnings("all")
private Fields fields;
@java.lang.SuppressWarnings("all")
private Integer offset;
@java.lang.SuppressWarnings("all")
private Integer limit;
@java.lang.SuppressWarnings("all")
private String after;
@java.lang.SuppressWarnings("all")
private Integer autocut;
@java.lang.SuppressWarnings("all")
private String withConsistencyLevel;
@java.lang.SuppressWarnings("all")
private NearTextArgument withNearTextFilter;
@java.lang.SuppressWarnings("all")
private Bm25Argument withBm25Filter;
@java.lang.SuppressWarnings("all")
private HybridArgument withHybridFilter;
@java.lang.SuppressWarnings("all")
private NearObjectArgument withNearObjectFilter;
@java.lang.SuppressWarnings("all")
private AskArgument withAskArgument;
@java.lang.SuppressWarnings("all")
private NearImageArgument withNearImageFilter;
@java.lang.SuppressWarnings("all")
private NearVectorArgument withNearVectorFilter;
@java.lang.SuppressWarnings("all")
private GroupArgument withGroupArgument;
@java.lang.SuppressWarnings("all")
private SortArguments withSortArguments;
@java.lang.SuppressWarnings("all")
private GenerativeSearchBuilder withGenerativeSearch;
@java.lang.SuppressWarnings("all")
private GroupByArgument withGroupByArgument;
@java.lang.SuppressWarnings("all")
private String tenant;
private WhereArgument withWhereFilter;
@Deprecated
public GetBuilderBuilder withWhereFilter(WhereFilter whereFilter) {
this.withWhereFilter = WhereArgument.builder().filter(whereFilter).build();
return this;
}
public GetBuilderBuilder withWhereFilter(WhereArgument whereArgument) {
this.withWhereFilter = whereArgument;
return this;
}
@java.lang.SuppressWarnings("all")
GetBuilderBuilder() {
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder className(final String className) {
this.className = className;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder fields(final Fields fields) {
this.fields = fields;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder offset(final Integer offset) {
this.offset = offset;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder limit(final Integer limit) {
this.limit = limit;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder after(final String after) {
this.after = after;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder autocut(final Integer autocut) {
this.autocut = autocut;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withConsistencyLevel(final String withConsistencyLevel) {
this.withConsistencyLevel = withConsistencyLevel;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withNearTextFilter(final NearTextArgument withNearTextFilter) {
this.withNearTextFilter = withNearTextFilter;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withBm25Filter(final Bm25Argument withBm25Filter) {
this.withBm25Filter = withBm25Filter;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withHybridFilter(final HybridArgument withHybridFilter) {
this.withHybridFilter = withHybridFilter;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withNearObjectFilter(final NearObjectArgument withNearObjectFilter) {
this.withNearObjectFilter = withNearObjectFilter;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withAskArgument(final AskArgument withAskArgument) {
this.withAskArgument = withAskArgument;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withNearImageFilter(final NearImageArgument withNearImageFilter) {
this.withNearImageFilter = withNearImageFilter;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withNearVectorFilter(final NearVectorArgument withNearVectorFilter) {
this.withNearVectorFilter = withNearVectorFilter;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withGroupArgument(final GroupArgument withGroupArgument) {
this.withGroupArgument = withGroupArgument;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withSortArguments(final SortArguments withSortArguments) {
this.withSortArguments = withSortArguments;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withGenerativeSearch(final GenerativeSearchBuilder withGenerativeSearch) {
this.withGenerativeSearch = withGenerativeSearch;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder withGroupByArgument(final GroupByArgument withGroupByArgument) {
this.withGroupByArgument = withGroupByArgument;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public GetBuilder.GetBuilderBuilder tenant(final String tenant) {
this.tenant = tenant;
return this;
}
@java.lang.SuppressWarnings("all")
public GetBuilder build() {
return new GetBuilder(this.className, this.fields, this.offset, this.limit, this.after, this.autocut, this.withConsistencyLevel, this.withWhereFilter, this.withNearTextFilter, this.withBm25Filter, this.withHybridFilter, this.withNearObjectFilter, this.withAskArgument, this.withNearImageFilter, this.withNearVectorFilter, this.withGroupArgument, this.withSortArguments, this.withGenerativeSearch, this.withGroupByArgument, this.tenant);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GetBuilder.GetBuilderBuilder(className=" + this.className + ", fields=" + this.fields + ", offset=" + this.offset + ", limit=" + this.limit + ", after=" + this.after + ", autocut=" + this.autocut + ", withConsistencyLevel=" + this.withConsistencyLevel + ", withWhereFilter=" + this.withWhereFilter + ", withNearTextFilter=" + this.withNearTextFilter + ", withBm25Filter=" + this.withBm25Filter + ", withHybridFilter=" + this.withHybridFilter + ", withNearObjectFilter=" + this.withNearObjectFilter + ", withAskArgument=" + this.withAskArgument + ", withNearImageFilter=" + this.withNearImageFilter + ", withNearVectorFilter=" + this.withNearVectorFilter + ", withGroupArgument=" + this.withGroupArgument + ", withSortArguments=" + this.withSortArguments + ", withGenerativeSearch=" + this.withGenerativeSearch + ", withGroupByArgument=" + this.withGroupByArgument + ", tenant=" + this.tenant + ")";
}
}
@java.lang.SuppressWarnings("all")
GetBuilder(final String className, final Fields fields, final Integer offset, final Integer limit, final String after, final Integer autocut, final String withConsistencyLevel, final WhereArgument withWhereFilter, final NearTextArgument withNearTextFilter, final Bm25Argument withBm25Filter, final HybridArgument withHybridFilter, final NearObjectArgument withNearObjectFilter, final AskArgument withAskArgument, final NearImageArgument withNearImageFilter, final NearVectorArgument withNearVectorFilter, final GroupArgument withGroupArgument, final SortArguments withSortArguments, final GenerativeSearchBuilder withGenerativeSearch, final GroupByArgument withGroupByArgument, final String tenant) {
this.className = className;
this.fields = fields;
this.offset = offset;
this.limit = limit;
this.after = after;
this.autocut = autocut;
this.withConsistencyLevel = withConsistencyLevel;
this.withWhereFilter = withWhereFilter;
this.withNearTextFilter = withNearTextFilter;
this.withBm25Filter = withBm25Filter;
this.withHybridFilter = withHybridFilter;
this.withNearObjectFilter = withNearObjectFilter;
this.withAskArgument = withAskArgument;
this.withNearImageFilter = withNearImageFilter;
this.withNearVectorFilter = withNearVectorFilter;
this.withGroupArgument = withGroupArgument;
this.withSortArguments = withSortArguments;
this.withGenerativeSearch = withGenerativeSearch;
this.withGroupByArgument = withGroupByArgument;
this.tenant = tenant;
}
@java.lang.SuppressWarnings("all")
public static GetBuilder.GetBuilderBuilder builder() {
return new GetBuilder.GetBuilderBuilder();
}
@java.lang.SuppressWarnings("all")
public String getClassName() {
return this.className;
}
@java.lang.SuppressWarnings("all")
public Fields getFields() {
return this.fields;
}
@java.lang.SuppressWarnings("all")
public Integer getOffset() {
return this.offset;
}
@java.lang.SuppressWarnings("all")
public Integer getLimit() {
return this.limit;
}
@java.lang.SuppressWarnings("all")
public String getAfter() {
return this.after;
}
@java.lang.SuppressWarnings("all")
public Integer getAutocut() {
return this.autocut;
}
@java.lang.SuppressWarnings("all")
public String getWithConsistencyLevel() {
return this.withConsistencyLevel;
}
@java.lang.SuppressWarnings("all")
public WhereArgument getWithWhereFilter() {
return this.withWhereFilter;
}
@java.lang.SuppressWarnings("all")
public NearTextArgument getWithNearTextFilter() {
return this.withNearTextFilter;
}
@java.lang.SuppressWarnings("all")
public Bm25Argument getWithBm25Filter() {
return this.withBm25Filter;
}
@java.lang.SuppressWarnings("all")
public HybridArgument getWithHybridFilter() {
return this.withHybridFilter;
}
@java.lang.SuppressWarnings("all")
public NearObjectArgument getWithNearObjectFilter() {
return this.withNearObjectFilter;
}
@java.lang.SuppressWarnings("all")
public AskArgument getWithAskArgument() {
return this.withAskArgument;
}
@java.lang.SuppressWarnings("all")
public NearImageArgument getWithNearImageFilter() {
return this.withNearImageFilter;
}
@java.lang.SuppressWarnings("all")
public NearVectorArgument getWithNearVectorFilter() {
return this.withNearVectorFilter;
}
@java.lang.SuppressWarnings("all")
public GroupArgument getWithGroupArgument() {
return this.withGroupArgument;
}
@java.lang.SuppressWarnings("all")
public SortArguments getWithSortArguments() {
return this.withSortArguments;
}
@java.lang.SuppressWarnings("all")
public GenerativeSearchBuilder getWithGenerativeSearch() {
return this.withGenerativeSearch;
}
@java.lang.SuppressWarnings("all")
public GroupByArgument getWithGroupByArgument() {
return this.withGroupByArgument;
}
@java.lang.SuppressWarnings("all")
public String getTenant() {
return this.tenant;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "GetBuilder(className=" + this.getClassName() + ", fields=" + this.getFields() + ", offset=" + this.getOffset() + ", limit=" + this.getLimit() + ", after=" + this.getAfter() + ", autocut=" + this.getAutocut() + ", withConsistencyLevel=" + this.getWithConsistencyLevel() + ", withWhereFilter=" + this.getWithWhereFilter() + ", withNearTextFilter=" + this.getWithNearTextFilter() + ", withBm25Filter=" + this.getWithBm25Filter() + ", withHybridFilter=" + this.getWithHybridFilter() + ", withNearObjectFilter=" + this.getWithNearObjectFilter() + ", withAskArgument=" + this.getWithAskArgument() + ", withNearImageFilter=" + this.getWithNearImageFilter() + ", withNearVectorFilter=" + this.getWithNearVectorFilter() + ", withGroupArgument=" + this.getWithGroupArgument() + ", withSortArguments=" + this.getWithSortArguments() + ", withGenerativeSearch=" + this.getWithGenerativeSearch() + ", withGroupByArgument=" + this.getWithGroupByArgument() + ", tenant=" + this.getTenant() + ")";
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof GetBuilder)) return false;
final GetBuilder other = (GetBuilder) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$offset = this.getOffset();
final java.lang.Object other$offset = other.getOffset();
if (this$offset == null ? other$offset != null : !this$offset.equals(other$offset)) return false;
final java.lang.Object this$limit = this.getLimit();
final java.lang.Object other$limit = other.getLimit();
if (this$limit == null ? other$limit != null : !this$limit.equals(other$limit)) return false;
final java.lang.Object this$autocut = this.getAutocut();
final java.lang.Object other$autocut = other.getAutocut();
if (this$autocut == null ? other$autocut != null : !this$autocut.equals(other$autocut)) return false;
final java.lang.Object this$className = this.getClassName();
final java.lang.Object other$className = other.getClassName();
if (this$className == null ? other$className != null : !this$className.equals(other$className)) return false;
final java.lang.Object this$fields = this.getFields();
final java.lang.Object other$fields = other.getFields();
if (this$fields == null ? other$fields != null : !this$fields.equals(other$fields)) return false;
final java.lang.Object this$after = this.getAfter();
final java.lang.Object other$after = other.getAfter();
if (this$after == null ? other$after != null : !this$after.equals(other$after)) return false;
final java.lang.Object this$withConsistencyLevel = this.getWithConsistencyLevel();
final java.lang.Object other$withConsistencyLevel = other.getWithConsistencyLevel();
if (this$withConsistencyLevel == null ? other$withConsistencyLevel != null : !this$withConsistencyLevel.equals(other$withConsistencyLevel)) return false;
final java.lang.Object this$withWhereFilter = this.getWithWhereFilter();
final java.lang.Object other$withWhereFilter = other.getWithWhereFilter();
if (this$withWhereFilter == null ? other$withWhereFilter != null : !this$withWhereFilter.equals(other$withWhereFilter)) return false;
final java.lang.Object this$withNearTextFilter = this.getWithNearTextFilter();
final java.lang.Object other$withNearTextFilter = other.getWithNearTextFilter();
if (this$withNearTextFilter == null ? other$withNearTextFilter != null : !this$withNearTextFilter.equals(other$withNearTextFilter)) return false;
final java.lang.Object this$withBm25Filter = this.getWithBm25Filter();
final java.lang.Object other$withBm25Filter = other.getWithBm25Filter();
if (this$withBm25Filter == null ? other$withBm25Filter != null : !this$withBm25Filter.equals(other$withBm25Filter)) return false;
final java.lang.Object this$withHybridFilter = this.getWithHybridFilter();
final java.lang.Object other$withHybridFilter = other.getWithHybridFilter();
if (this$withHybridFilter == null ? other$withHybridFilter != null : !this$withHybridFilter.equals(other$withHybridFilter)) return false;
final java.lang.Object this$withNearObjectFilter = this.getWithNearObjectFilter();
final java.lang.Object other$withNearObjectFilter = other.getWithNearObjectFilter();
if (this$withNearObjectFilter == null ? other$withNearObjectFilter != null : !this$withNearObjectFilter.equals(other$withNearObjectFilter)) return false;
final java.lang.Object this$withAskArgument = this.getWithAskArgument();
final java.lang.Object other$withAskArgument = other.getWithAskArgument();
if (this$withAskArgument == null ? other$withAskArgument != null : !this$withAskArgument.equals(other$withAskArgument)) return false;
final java.lang.Object this$withNearImageFilter = this.getWithNearImageFilter();
final java.lang.Object other$withNearImageFilter = other.getWithNearImageFilter();
if (this$withNearImageFilter == null ? other$withNearImageFilter != null : !this$withNearImageFilter.equals(other$withNearImageFilter)) return false;
final java.lang.Object this$withNearVectorFilter = this.getWithNearVectorFilter();
final java.lang.Object other$withNearVectorFilter = other.getWithNearVectorFilter();
if (this$withNearVectorFilter == null ? other$withNearVectorFilter != null : !this$withNearVectorFilter.equals(other$withNearVectorFilter)) return false;
final java.lang.Object this$withGroupArgument = this.getWithGroupArgument();
final java.lang.Object other$withGroupArgument = other.getWithGroupArgument();
if (this$withGroupArgument == null ? other$withGroupArgument != null : !this$withGroupArgument.equals(other$withGroupArgument)) return false;
final java.lang.Object this$withSortArguments = this.getWithSortArguments();
final java.lang.Object other$withSortArguments = other.getWithSortArguments();
if (this$withSortArguments == null ? other$withSortArguments != null : !this$withSortArguments.equals(other$withSortArguments)) return false;
final java.lang.Object this$withGenerativeSearch = this.getWithGenerativeSearch();
final java.lang.Object other$withGenerativeSearch = other.getWithGenerativeSearch();
if (this$withGenerativeSearch == null ? other$withGenerativeSearch != null : !this$withGenerativeSearch.equals(other$withGenerativeSearch)) return false;
final java.lang.Object this$withGroupByArgument = this.getWithGroupByArgument();
final java.lang.Object other$withGroupByArgument = other.getWithGroupByArgument();
if (this$withGroupByArgument == null ? other$withGroupByArgument != null : !this$withGroupByArgument.equals(other$withGroupByArgument)) return false;
final java.lang.Object this$tenant = this.getTenant();
final java.lang.Object other$tenant = other.getTenant();
if (this$tenant == null ? other$tenant != null : !this$tenant.equals(other$tenant)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof GetBuilder;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $offset = this.getOffset();
result = result * PRIME + ($offset == null ? 43 : $offset.hashCode());
final java.lang.Object $limit = this.getLimit();
result = result * PRIME + ($limit == null ? 43 : $limit.hashCode());
final java.lang.Object $autocut = this.getAutocut();
result = result * PRIME + ($autocut == null ? 43 : $autocut.hashCode());
final java.lang.Object $className = this.getClassName();
result = result * PRIME + ($className == null ? 43 : $className.hashCode());
final java.lang.Object $fields = this.getFields();
result = result * PRIME + ($fields == null ? 43 : $fields.hashCode());
final java.lang.Object $after = this.getAfter();
result = result * PRIME + ($after == null ? 43 : $after.hashCode());
final java.lang.Object $withConsistencyLevel = this.getWithConsistencyLevel();
result = result * PRIME + ($withConsistencyLevel == null ? 43 : $withConsistencyLevel.hashCode());
final java.lang.Object $withWhereFilter = this.getWithWhereFilter();
result = result * PRIME + ($withWhereFilter == null ? 43 : $withWhereFilter.hashCode());
final java.lang.Object $withNearTextFilter = this.getWithNearTextFilter();
result = result * PRIME + ($withNearTextFilter == null ? 43 : $withNearTextFilter.hashCode());
final java.lang.Object $withBm25Filter = this.getWithBm25Filter();
result = result * PRIME + ($withBm25Filter == null ? 43 : $withBm25Filter.hashCode());
final java.lang.Object $withHybridFilter = this.getWithHybridFilter();
result = result * PRIME + ($withHybridFilter == null ? 43 : $withHybridFilter.hashCode());
final java.lang.Object $withNearObjectFilter = this.getWithNearObjectFilter();
result = result * PRIME + ($withNearObjectFilter == null ? 43 : $withNearObjectFilter.hashCode());
final java.lang.Object $withAskArgument = this.getWithAskArgument();
result = result * PRIME + ($withAskArgument == null ? 43 : $withAskArgument.hashCode());
final java.lang.Object $withNearImageFilter = this.getWithNearImageFilter();
result = result * PRIME + ($withNearImageFilter == null ? 43 : $withNearImageFilter.hashCode());
final java.lang.Object $withNearVectorFilter = this.getWithNearVectorFilter();
result = result * PRIME + ($withNearVectorFilter == null ? 43 : $withNearVectorFilter.hashCode());
final java.lang.Object $withGroupArgument = this.getWithGroupArgument();
result = result * PRIME + ($withGroupArgument == null ? 43 : $withGroupArgument.hashCode());
final java.lang.Object $withSortArguments = this.getWithSortArguments();
result = result * PRIME + ($withSortArguments == null ? 43 : $withSortArguments.hashCode());
final java.lang.Object $withGenerativeSearch = this.getWithGenerativeSearch();
result = result * PRIME + ($withGenerativeSearch == null ? 43 : $withGenerativeSearch.hashCode());
final java.lang.Object $withGroupByArgument = this.getWithGroupByArgument();
result = result * PRIME + ($withGroupByArgument == null ? 43 : $withGroupByArgument.hashCode());
final java.lang.Object $tenant = this.getTenant();
result = result * PRIME + ($tenant == null ? 43 : $tenant.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy