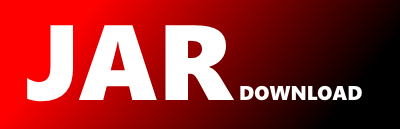
io.weaviate.client.v1.schema.model.Property Maven / Gradle / Ivy
// Generated by delombok at Thu Jul 06 20:35:08 UTC 2023
package io.weaviate.client.v1.schema.model;
import java.util.List;
public class Property {
private final String name;
private final List dataType;
private final String description;
private final String tokenization;
/**
* As of Weaviate v1.19 'indexInverted' is deprecated and replaced by 'indexFilterable'
* and 'indexSearchable'.
* See inverted index
*/
@Deprecated
private final Boolean indexInverted;
private final Boolean indexFilterable;
private final Boolean indexSearchable;
private final Object moduleConfig;
@java.lang.SuppressWarnings("all")
Property(final String name, final List dataType, final String description, final String tokenization, final Boolean indexInverted, final Boolean indexFilterable, final Boolean indexSearchable, final Object moduleConfig) {
this.name = name;
this.dataType = dataType;
this.description = description;
this.tokenization = tokenization;
this.indexInverted = indexInverted;
this.indexFilterable = indexFilterable;
this.indexSearchable = indexSearchable;
this.moduleConfig = moduleConfig;
}
@java.lang.SuppressWarnings("all")
public static class PropertyBuilder {
@java.lang.SuppressWarnings("all")
private String name;
@java.lang.SuppressWarnings("all")
private List dataType;
@java.lang.SuppressWarnings("all")
private String description;
@java.lang.SuppressWarnings("all")
private String tokenization;
@java.lang.SuppressWarnings("all")
private Boolean indexInverted;
@java.lang.SuppressWarnings("all")
private Boolean indexFilterable;
@java.lang.SuppressWarnings("all")
private Boolean indexSearchable;
@java.lang.SuppressWarnings("all")
private Object moduleConfig;
@java.lang.SuppressWarnings("all")
PropertyBuilder() {
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder name(final String name) {
this.name = name;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder dataType(final List dataType) {
this.dataType = dataType;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder description(final String description) {
this.description = description;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder tokenization(final String tokenization) {
this.tokenization = tokenization;
return this;
}
/**
* As of Weaviate v1.19 'indexInverted' is deprecated and replaced by 'indexFilterable'
* and 'indexSearchable'.
* See inverted index
* @return {@code this}.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder indexInverted(final Boolean indexInverted) {
this.indexInverted = indexInverted;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder indexFilterable(final Boolean indexFilterable) {
this.indexFilterable = indexFilterable;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder indexSearchable(final Boolean indexSearchable) {
this.indexSearchable = indexSearchable;
return this;
}
/**
* @return {@code this}.
*/
@java.lang.SuppressWarnings("all")
public Property.PropertyBuilder moduleConfig(final Object moduleConfig) {
this.moduleConfig = moduleConfig;
return this;
}
@java.lang.SuppressWarnings("all")
public Property build() {
return new Property(this.name, this.dataType, this.description, this.tokenization, this.indexInverted, this.indexFilterable, this.indexSearchable, this.moduleConfig);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "Property.PropertyBuilder(name=" + this.name + ", dataType=" + this.dataType + ", description=" + this.description + ", tokenization=" + this.tokenization + ", indexInverted=" + this.indexInverted + ", indexFilterable=" + this.indexFilterable + ", indexSearchable=" + this.indexSearchable + ", moduleConfig=" + this.moduleConfig + ")";
}
}
@java.lang.SuppressWarnings("all")
public static Property.PropertyBuilder builder() {
return new Property.PropertyBuilder();
}
@java.lang.SuppressWarnings("all")
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
public List getDataType() {
return this.dataType;
}
@java.lang.SuppressWarnings("all")
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
public String getTokenization() {
return this.tokenization;
}
/**
* As of Weaviate v1.19 'indexInverted' is deprecated and replaced by 'indexFilterable'
* and 'indexSearchable'.
* See inverted index
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
public Boolean getIndexInverted() {
return this.indexInverted;
}
@java.lang.SuppressWarnings("all")
public Boolean getIndexFilterable() {
return this.indexFilterable;
}
@java.lang.SuppressWarnings("all")
public Boolean getIndexSearchable() {
return this.indexSearchable;
}
@java.lang.SuppressWarnings("all")
public Object getModuleConfig() {
return this.moduleConfig;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public java.lang.String toString() {
return "Property(name=" + this.getName() + ", dataType=" + this.getDataType() + ", description=" + this.getDescription() + ", tokenization=" + this.getTokenization() + ", indexInverted=" + this.getIndexInverted() + ", indexFilterable=" + this.getIndexFilterable() + ", indexSearchable=" + this.getIndexSearchable() + ", moduleConfig=" + this.getModuleConfig() + ")";
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Property)) return false;
final Property other = (Property) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$indexInverted = this.getIndexInverted();
final java.lang.Object other$indexInverted = other.getIndexInverted();
if (this$indexInverted == null ? other$indexInverted != null : !this$indexInverted.equals(other$indexInverted)) return false;
final java.lang.Object this$indexFilterable = this.getIndexFilterable();
final java.lang.Object other$indexFilterable = other.getIndexFilterable();
if (this$indexFilterable == null ? other$indexFilterable != null : !this$indexFilterable.equals(other$indexFilterable)) return false;
final java.lang.Object this$indexSearchable = this.getIndexSearchable();
final java.lang.Object other$indexSearchable = other.getIndexSearchable();
if (this$indexSearchable == null ? other$indexSearchable != null : !this$indexSearchable.equals(other$indexSearchable)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$dataType = this.getDataType();
final java.lang.Object other$dataType = other.getDataType();
if (this$dataType == null ? other$dataType != null : !this$dataType.equals(other$dataType)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$tokenization = this.getTokenization();
final java.lang.Object other$tokenization = other.getTokenization();
if (this$tokenization == null ? other$tokenization != null : !this$tokenization.equals(other$tokenization)) return false;
final java.lang.Object this$moduleConfig = this.getModuleConfig();
final java.lang.Object other$moduleConfig = other.getModuleConfig();
if (this$moduleConfig == null ? other$moduleConfig != null : !this$moduleConfig.equals(other$moduleConfig)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Property;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $indexInverted = this.getIndexInverted();
result = result * PRIME + ($indexInverted == null ? 43 : $indexInverted.hashCode());
final java.lang.Object $indexFilterable = this.getIndexFilterable();
result = result * PRIME + ($indexFilterable == null ? 43 : $indexFilterable.hashCode());
final java.lang.Object $indexSearchable = this.getIndexSearchable();
result = result * PRIME + ($indexSearchable == null ? 43 : $indexSearchable.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $dataType = this.getDataType();
result = result * PRIME + ($dataType == null ? 43 : $dataType.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $tokenization = this.getTokenization();
result = result * PRIME + ($tokenization == null ? 43 : $tokenization.hashCode());
final java.lang.Object $moduleConfig = this.getModuleConfig();
result = result * PRIME + ($moduleConfig == null ? 43 : $moduleConfig.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy