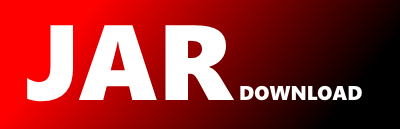
io.wisetime.generated.connect.TimeRow Maven / Gradle / Ivy
package io.wisetime.generated.connect;
import io.swagger.annotations.ApiModel;
import io.swagger.annotations.ApiModelProperty;
import javax.validation.constraints.*;
import javax.validation.Valid;
import io.swagger.annotations.*;
import java.util.Objects;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonValue;
public class TimeRow {
private @Valid String activity;
private @Valid String description;
private @Valid Integer activityHour;
private @Valid Integer durationSecs;
private @Valid Long submittedDate = null;
private @Valid String modifier;
public enum SourceEnum {
WT_DESKTOP(String.valueOf("WT_DESKTOP")), USER_MANUAL_TIME(String.valueOf("USER_MANUAL_TIME"));
private String value;
SourceEnum (String v) {
value = v;
}
public String value() {
return value;
}
@Override
@JsonValue
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SourceEnum fromValue(String v) {
for (SourceEnum b : SourceEnum.values()) {
if (String.valueOf(b.value).equals(v)) {
return b;
}
}
throw new IllegalArgumentException("Unexpected value '" + v + "'");
}
}
private @Valid SourceEnum source;
/**
**/
public TimeRow activity(String activity) {
this.activity = activity;
return this;
}
@ApiModelProperty(value = "")
@JsonProperty("activity")
public String getActivity() {
return activity;
}
public void setActivity(String activity) {
this.activity = activity;
}
/**
**/
public TimeRow description(String description) {
this.description = description;
return this;
}
@ApiModelProperty(value = "")
@JsonProperty("description")
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
/**
* The hour of the posted time in UTC. yyyyMMddhh
**/
public TimeRow activityHour(Integer activityHour) {
this.activityHour = activityHour;
return this;
}
@ApiModelProperty(value = "The hour of the posted time in UTC. yyyyMMddhh")
@JsonProperty("activityHour")
public Integer getActivityHour() {
return activityHour;
}
public void setActivityHour(Integer activityHour) {
this.activityHour = activityHour;
}
/**
**/
public TimeRow durationSecs(Integer durationSecs) {
this.durationSecs = durationSecs;
return this;
}
@ApiModelProperty(value = "")
@JsonProperty("durationSecs")
public Integer getDurationSecs() {
return durationSecs;
}
public void setDurationSecs(Integer durationSecs) {
this.durationSecs = durationSecs;
}
/**
* Time when the activity was submitted by the user in UTC. yyyyMMddHHmmSSsss
**/
public TimeRow submittedDate(Long submittedDate) {
this.submittedDate = submittedDate;
return this;
}
@ApiModelProperty(value = "Time when the activity was submitted by the user in UTC. yyyyMMddHHmmSSsss")
@JsonProperty("submittedDate")
public Long getSubmittedDate() {
return submittedDate;
}
public void setSubmittedDate(Long submittedDate) {
this.submittedDate = submittedDate;
}
/**
**/
public TimeRow modifier(String modifier) {
this.modifier = modifier;
return this;
}
@ApiModelProperty(value = "")
@JsonProperty("modifier")
public String getModifier() {
return modifier;
}
public void setModifier(String modifier) {
this.modifier = modifier;
}
/**
* This field describes the origin of the posted. Time logs can either come from the desktop client (WT_DESKTOP) or be created manually by the user (USER_MANUAL_TIME)
**/
public TimeRow source(SourceEnum source) {
this.source = source;
return this;
}
@ApiModelProperty(value = "This field describes the origin of the posted. Time logs can either come from the desktop client (WT_DESKTOP) or be created manually by the user (USER_MANUAL_TIME) ")
@JsonProperty("source")
public SourceEnum getSource() {
return source;
}
public void setSource(SourceEnum source) {
this.source = source;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
TimeRow timeRow = (TimeRow) o;
return Objects.equals(activity, timeRow.activity) &&
Objects.equals(description, timeRow.description) &&
Objects.equals(activityHour, timeRow.activityHour) &&
Objects.equals(durationSecs, timeRow.durationSecs) &&
Objects.equals(submittedDate, timeRow.submittedDate) &&
Objects.equals(modifier, timeRow.modifier) &&
Objects.equals(source, timeRow.source);
}
@Override
public int hashCode() {
return Objects.hash(activity, description, activityHour, durationSecs, submittedDate, modifier, source);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class TimeRow {\n");
sb.append(" activity: ").append(toIndentedString(activity)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" activityHour: ").append(toIndentedString(activityHour)).append("\n");
sb.append(" durationSecs: ").append(toIndentedString(durationSecs)).append("\n");
sb.append(" submittedDate: ").append(toIndentedString(submittedDate)).append("\n");
sb.append(" modifier: ").append(toIndentedString(modifier)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy