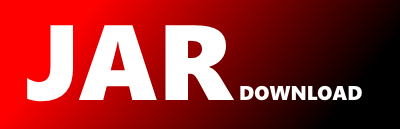
io.wizzie.normalizer.funcs.MapperStoreFunction Maven / Gradle / Ivy
package io.wizzie.normalizer.funcs;
import io.wizzie.metrics.MetricsManager;
import io.wizzie.normalizer.base.utils.ConversionUtils;
import org.apache.kafka.streams.KeyValue;
import org.apache.kafka.streams.kstream.Transformer;
import org.apache.kafka.streams.processor.ProcessorContext;
import org.apache.kafka.streams.processor.PunctuationType;
import org.apache.kafka.streams.processor.StateStore;
import org.apache.kafka.streams.state.KeyValueStore;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static io.wizzie.normalizer.base.utils.Constants.*;
/**
* This class is used to do a mapper process, but it offers a key value store to operate with multiples states
*/
public abstract class MapperStoreFunction implements Function>>,
Transformer, KeyValue>> {
private final Logger log = LoggerFactory.getLogger(getClass());
private Map properties;
private Map stores = new HashMap<>();
private List availableStores;
private String appId;
private Long windownTimeMs;
private MetricsManager metricsManager;
/**
* Initialize mapper store function
* @param properties Properties for mapper store function
* @param metricsManager MetricsManager object for mapper store function
*/
@Override
public void init(Map properties, MetricsManager metricsManager) {
this.availableStores = (List) properties.get(__STORES);
this.appId = (String) properties.get(__APP_ID);
this.windownTimeMs = ConversionUtils.toLong(properties.get(__WINDOW_TIME_MS));
this.properties = properties;
this.metricsManager = metricsManager;
}
/**
* Inialize mapper store function
* @param context The context of processor for mapper store function
*/
@Override
public void init(ProcessorContext context) {
if(windownTimeMs != null) context.schedule(windownTimeMs, PunctuationType.STREAM_TIME, this::window);
availableStores.forEach((storeName) ->
stores.put(storeName, (KeyValueStore) context.getStateStore(String.format("%s_%s", appId, storeName)))
);
prepare(properties, metricsManager);
log.info(" with {}", toString());
}
/**
* Process a Key-Value message of Kafka
* @param key The key of Kafka message
* @param value The value of Kafka message
* @return A Key-Value message after process
*/
@Override
public KeyValue> transform(String key, Map value) {
return process(key, value);
}
/**
* Close all opened stores and stop process
*/
@Override
public void close() {
stores.values().forEach(StateStore::close);
stop();
}
/**
* Allow to get a list of available stores
* @return List of available stores
*/
public List getAvailableStores(){
return availableStores;
}
/**
* Allow to get a KeyValue store of its name
* @param storeName Name of Key-Value store
* @param Value Type
* @return A Key-Value store
*/
public KeyValueStore getStore(String storeName){
return (KeyValueStore) stores.get(storeName);
}
/**
* This method allow implement window calls
* @param timestamp where the window must be called
* @return A messages that are added to the current stream
*/
public abstract KeyValue> window(long timestamp);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy