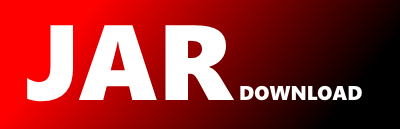
io.wizzie.normalizer.funcs.impl.DecimalPrecisionMapper Maven / Gradle / Ivy
package io.wizzie.normalizer.funcs.impl;
import io.wizzie.metrics.MetricsManager;
import io.wizzie.normalizer.funcs.MapperFunction;
import org.apache.kafka.streams.KeyValue;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.math.BigDecimal;
import java.math.RoundingMode;
import java.text.NumberFormat;
import java.util.*;
public class DecimalPrecisionMapper extends MapperFunction {
private static final Logger log = LoggerFactory.getLogger(ArithmeticMapper.class);
private final static String DIMENSIONS = "dimensions";
private final static String NUMBER_OF_DECIMALS = "numberOfDecimals";
private final static String SCALES = "scales";
private List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy