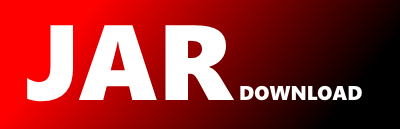
io.xream.x7.common.bean.Criteria Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.xream.x7.common.bean;
import com.fasterxml.jackson.annotation.JsonIgnore;
import io.xream.x7.common.util.BeanUtil;
import io.xream.x7.common.util.BeanUtilX;
import io.xream.x7.common.util.StringUtil;
import io.xream.x7.common.web.Paged;
import java.io.Serializable;
import java.util.*;
/**
*
* @author sim
*
*/
public class Criteria implements CriteriaCondition, Paged, Routeable,Serializable {
private static final long serialVersionUID = 7088698915888081349L;
private Class> clz;
private boolean isTotalRowsIgnored;
private int page;
private int rows;
private Object routeKey;
private List sortList;
private List fixedSortList = new ArrayList<>();
private List listX = new ArrayList<>();
private String forceIndex;
@JsonIgnore
private transient Parsed parsed;
@JsonIgnore
private transient List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy