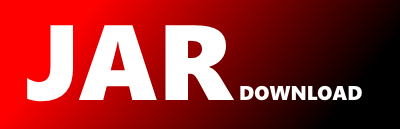
io.xream.x7.common.bean.condition.RefreshCondition Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.xream.x7.common.bean.condition;
import com.fasterxml.jackson.annotation.JsonIgnore;
import io.xream.x7.common.bean.*;
import java.util.*;
public class RefreshCondition extends ConditionCriteriaBuilder implements CriteriaCondition, Routeable {
private List refreshList = new ArrayList<>();
private String sourceScript;//FIXME
private List listX = new ArrayList<>();
private Object routeKey;
@JsonIgnore
private transient Class clz;
@JsonIgnore
private transient List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy