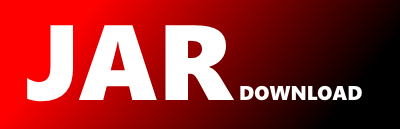
x7.core.bean.Parsed Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package x7.core.bean;
import x7.core.repository.X;
import x7.core.search.TagParsed;
import x7.core.util.BeanUtilX;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Parsed {
private boolean isChecked = false;
private Class clz;
private String tableName;
private boolean isNoSpec = true;
private final Map keyMap = new HashMap();
private final Map keyFieldMap = new HashMap();
private List beanElementList;
private Map elementMap = new HashMap();
private Map propertyMapperMap = new HashMap();
private Map mapperPropertyMap = new HashMap();
private boolean isNoCache;
private List keywordsList = new ArrayList();
private boolean isSearchable;
private Map tagMap = new HashMap();
public Class getClz() {
return clz;
}
public void setClz(Class clz) {
this.clz = clz;
}
public Parsed(Class clz){
this.clz = clz;
}
public String getId(){
return String.valueOf(keyMap.get(X.KEY_ONE));
}
public BeanElement getElement(String property){
return elementMap.get(property);
}
public Map getElementMap() {
return elementMap;
}
public Map getKeyMap() {
return keyMap;
}
public boolean contains(String property) {
return this.elementMap.containsKey(property);
}
public Map getKeyFieldMap() {
return keyFieldMap;
}
public Field getKeyField(int index){
return keyFieldMap.get(index);
}
public String getKey(int index){
if (keyMap.isEmpty() && index == X.KEY_ONE) //DEFAULT
return "id";
return keyMap.get(index);
}
public List getBeanElementList() {
return beanElementList;
}
public void setBeanElementList(List beanElementList) {
this.beanElementList = beanElementList;
for (BeanElement e : this.beanElementList){
String property = e.getProperty();
String mapper = e.getMapper();
this.elementMap.put(property, e);
this.propertyMapperMap.put(property, mapper);
this.mapperPropertyMap.put(mapper, property);
}
}
public boolean isChecked(){
return this.isChecked;
}
public void checked(){
this.isChecked = true;
}
public String getTableName() {
return tableName;
}
public void setTableName(String tableName) {
this.tableName = BeanUtilX.filterSQLKeyword(tableName);
}
public String getClzName() {
return this.clz.getSimpleName();
}
public boolean isNoCache() {
return isNoCache;
}
public void setNoCache(boolean isNoCache) {
this.isNoCache = isNoCache;
}
public List getKeywordsList() {
return keywordsList;
}
public void setKeywordsList(List keywordsList) {
this.keywordsList = keywordsList;
}
public boolean isSearchable() {
return isSearchable;
}
public void setSearchable(boolean isSearchable) {
this.isSearchable = isSearchable;
}
public String[] getKeywardsArr(){
String[] keywordsArr = new String[this.keywordsList.size()];
this.keywordsList.toArray(keywordsArr);
return keywordsArr;
}
public Map getTagMap() {
return tagMap;
}
public void setTagMap(Map tagMap) {
this.tagMap = tagMap;
}
public String getMapper(String property) {
return propertyMapperMap.get(property);
}
public String getProperty(String mapper){
return mapperPropertyMap.get(mapper);
}
public Map getPropertyMapperMap() {
return propertyMapperMap;
}
public Map getMapperPropertyMap() {
return mapperPropertyMap;
}
public boolean isNoSpec() {
return isNoSpec;
}
public void setNoSpec(boolean isNoSpec2) {
this.isNoSpec = isNoSpec2;
}
@Override
public String toString() {
return "Parsed [clz=" + clz + ", tableName=" + tableName + ", keyMap=" + keyMap + ", keyFieldMap=" + keyFieldMap
+ ", beanElementList=" + beanElementList + ", elementMap=" + elementMap +
", isNoCache=" + isNoCache + ", keywordsList=" + keywordsList
+ ", isSearchable=" + isSearchable + ", tagMap=" + tagMap + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy