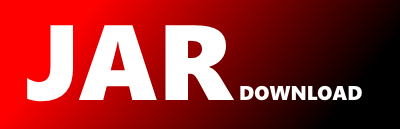
x7.core.util.IntMap Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package x7.core.util;
import java.io.Serializable;
/**
* 简单的int map
* 因为是int型,无法作null判断, 设计上用"-1"作为保留数字,key 和 value都不能为-1;
* 如果返回-1,表示没查到
* 当元数过多时, 请不要使用, 最好不要超过300个
* @author Sim
*
*/
public class IntMap implements Serializable{
private static final long serialVersionUID = 270700123037753057L;
private int capacity = 1;
private final static int KEY_BASE = -1;
private final static int VALUE_BASE = 0;
private int[] keys;
private int[] values;
public IntMap(){
this.keys = new int[1];
this.values = new int[1];
this.keys[0] = KEY_BASE;
this.values[0] = VALUE_BASE;
}
/**
* 如果未来size会超过10个,请使用
* @param capacity
*/
public IntMap(int capacity) {
if (capacity < 1){
System.out.println(" capacity必需大于0 ");
}
this.capacity = capacity;
create(capacity);
}
public int[] keys(){
int size = size();
int[] newKeys = new int[size];
int j=0;
int length = this.keys.length;
for (int i=0; i
* 如果计算出的value可能为-1
* 请硬编码解决
* @param key
* @param value
*/
public void put(int key, int value) {
if (key == -1 || value == -1){
System.out.println( " -1 is kept ");
}
int size = keys.length;
int index = -1;
for (int i=0; i < size; i++){
if (keys[i]==key || keys[i] == -1){
keys[i]=key;
index = i;
break;
}
}
if (index == -1){
int[] newKeys = new int[size+1];
int[] newValues = new int[size+1];
System.arraycopy(keys, 0, newKeys, 0, size);
System.arraycopy(values, 0, newValues, 0, size);
this.keys = newKeys;
this.values = newValues;
index = size;
this.keys[index] = key;
}
values[index] = value;
}
public void putAll(IntMap map){
for (int key : map.keys()) {
int origin = this.get(key);
this.put(key, origin + map.get(key));
}
}
public int remove(int key) throws Exception{
if (key == -1)
throw new Exception( " -1 is kept ");
int index = -1;
int size = keys.length;
for (int i=0; i < size; i++){
if (key == keys[i]){
index = i;
break;
}
}
if (index == -1)
return -1;
int result = values[index];
/*
* 移除
*/
this.keys[index] = -1;
this.values[index] = -1;
/*
* 如果MAP过长,则做实际的空间清楚
*/
int keySize = keys.length;
int length = 0;
for (int i=0; i capacity * 2){
size = size();
size = size < capacity ? capacity : size; // size 不能小于capacity
int[] newKeys = new int[size];
int[] newValues = new int[size];
int j = 0;
/*
* 复制数据
*/
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy