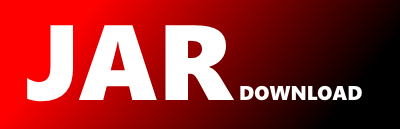
x7.core.bean.Criteria Maven / Gradle / Ivy
/*
* Licensed to the Apache Software Foundation (ASF) under one or more
* contributor license agreements. See the NOTICE file distributed with
* this work for additional information regarding copyright ownership.
* The ASF licenses this file to You under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package x7.core.bean;
import com.fasterxml.jackson.annotation.JsonIgnore;
import x7.core.util.BeanUtil;
import x7.core.util.StringUtil;
import x7.core.web.Direction;
import x7.core.web.Paged;
import java.io.Serializable;
import java.util.*;
/**
*
* @author sim
*
*/
public class Criteria implements CriteriaCondition, Paged, Serializable {
private static final long serialVersionUID = 7088698915888081349L;
private Class> clz;
private boolean isScroll = true;
private int page;
private int rows;
private List sortList;
private List fixedSortList = new ArrayList();
private List listX = new ArrayList();
private DataPermission dataPermission;//String,Or List LikeRight | In
@JsonIgnore
private transient Parsed parsed;
@JsonIgnore
public transient boolean isWhere = true;
@JsonIgnore
private transient List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy