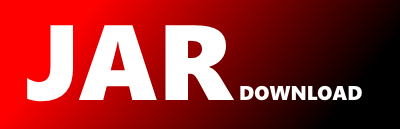
x7.repository.util.ResultSortUtil Maven / Gradle / Ivy
package x7.repository.util;
import x7.core.bean.BeanElement;
import x7.core.bean.Criteria;
import x7.core.bean.KV;
import x7.core.bean.Parsed;
import java.lang.reflect.InvocationTargetException;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
public class ResultSortUtil {
/**
* by orderIn0
* @param list
* @param criteria
* @param parsed
* @throws InvocationTargetException
* @throws IllegalAccessException
*/
public static void sort(List list, Criteria criteria, Parsed parsed) throws InvocationTargetException, IllegalAccessException {
if (list.isEmpty())
return;
List fixedSortList = criteria.getFixedSortList();
if (fixedSortList.isEmpty())
return;
KV kv0 = fixedSortList.get(0);
List tempList = new ArrayList<>();
tempList.addAll(list);
list.clear();
Class clz = parsed.getClz();
String property = kv0.k;
for (Object para : (List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy