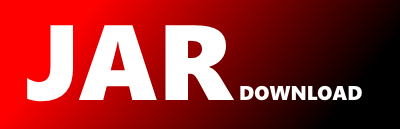
io.yawp.repository.RepositoryFeatures Maven / Gradle / Ivy
package io.yawp.repository;
import io.yawp.repository.actions.ActionKey;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
public class RepositoryFeatures {
private Map, EndpointFeatures>> endpoints;
private Map> paths;
private Map> kinds;
protected RepositoryFeatures() {
}
public RepositoryFeatures(Map, EndpointFeatures>> endpoints) {
this.endpoints = endpoints;
this.paths = new HashMap<>();
this.kinds = new HashMap<>();
initAndLoadPaths();
}
private void initAndLoadPaths() {
for (EndpointFeatures> endpoint : endpoints.values()) {
addKindToMap(endpoint);
addMapPathKind(endpoint);
}
}
private void addKindToMap(EndpointFeatures> endpoint) {
String kind = endpoint.getEndpointKind();
kinds.put(kind, endpoint.getClazz());
}
private void addMapPathKind(EndpointFeatures> endpoint) {
String endpointPath = endpoint.getEndpointPath();
if (endpointPath.isEmpty()) {
return;
}
assertIsValidPath(endpoint, endpointPath);
paths.put(endpointPath, endpoint.getClazz());
}
private void assertIsValidPath(EndpointFeatures> endpoint, String endpointPath) {
if (paths.get(endpointPath) != null) {
throw new RuntimeException("Repeated io.yawp path " + endpointPath + " for class "
+ endpoint.getClazz().getSimpleName() + " (already found in class " + paths.get(endpointPath).getSimpleName()
+ ")");
}
if (!isValidEndpointPath(endpointPath)) {
throw new RuntimeException("Invalid endpoint path " + endpointPath + " for class " + endpoint.getClazz().getSimpleName());
}
}
protected boolean isValidEndpointPath(String endpointName) {
char[] charArray = endpointName.toCharArray();
for (int i = 0; i < charArray.length; i++) {
char c = charArray[i];
if (i == 0) {
if (c != '/') {
return false;
}
continue;
}
if (!(Character.isAlphabetic(c) || c == '_' || c == '-')) {
return false;
}
}
return true;
}
public EndpointFeatures> getByClazz(Class> clazz) {
return endpoints.get(clazz);
}
public EndpointFeatures> getByPath(String endpointPath) {
Class> clazz = paths.get(endpointPath);
if (clazz == null) {
throw new EndpointNotFoundException(endpointPath);
}
return getByClazz(clazz);
}
public Class> getClazzByKind(String kind) {
return kinds.get(kind);
}
public boolean hasCustomAction(String endpointPath, ActionKey actionKey) {
EndpointFeatures> endpointFeatures = getByPath(endpointPath);
return endpointFeatures.hasCustomAction(actionKey);
}
public boolean hasCustomAction(Class> clazz, ActionKey actionKey) {
EndpointFeatures> endpointFeatures = getByClazz(clazz);
return endpointFeatures.hasCustomAction(actionKey);
}
public Set> getEndpointClazzes() {
return endpoints.keySet();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy