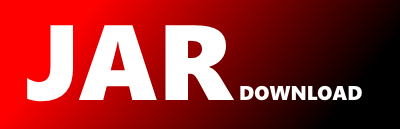
io.yawp.servlet.rest.RestAction Maven / Gradle / Ivy
package io.yawp.servlet.rest;
import io.yawp.commons.http.HttpException;
import io.yawp.commons.http.HttpResponse;
import io.yawp.commons.http.HttpVerb;
import io.yawp.commons.http.JsonResponse;
import io.yawp.commons.utils.JsonUtils;
import io.yawp.repository.EndpointFeatures;
import io.yawp.repository.FutureObject;
import io.yawp.repository.IdRef;
import io.yawp.repository.Repository;
import io.yawp.repository.actions.ActionKey;
import io.yawp.repository.hooks.RepositoryHooks;
import io.yawp.repository.query.QueryBuilder;
import io.yawp.repository.shields.Shield;
import io.yawp.repository.shields.ShieldInfo;
import io.yawp.repository.transformers.RepositoryTransformers;
import java.util.List;
import java.util.Map;
public abstract class RestAction {
private static final String DEFAULT_TRANSFORMER_NAME = "defaults";
protected static final String QUERY_OPTIONS = "q";
protected static final String TRANSFORMER = "t";
protected Repository r;
// TODO: Remove this flag, it is used only for tests. Hooks are already disabled in the RepositoryScanner class.
protected boolean enableHooks;
protected Class> endpointClazz;
protected IdRef> id;
protected Map params;
protected ActionKey customActionKey;
protected String actionName;
protected String transformerName;
protected Shield> shield;
private List> objects;
protected String requestJson;
private boolean requestBodyJsonArray;
public RestAction(String actionName) {
this.actionName = actionName;
}
public void setRepository(Repository r) {
this.r = r;
}
public void setEnableHooks(boolean enableHooks) {
this.enableHooks = enableHooks;
}
public void setEndpointClazz(Class> clazz) {
this.endpointClazz = clazz;
}
public void setId(IdRef> id) {
this.id = id;
}
public void setParams(Map params) {
this.params = params;
}
public void setCustomActionKey(ActionKey customActionKey) {
this.customActionKey = customActionKey;
}
public boolean isRequestBodyJsonArray() {
return requestBodyJsonArray;
}
public void setRequestJson(String requestJson) {
this.requestJson = requestJson;
this.requestBodyJsonArray = JsonUtils.isJsonArray(requestJson);
}
public void setRequestBodyJsonArray(boolean requestBodyJsonArray) {
this.requestBodyJsonArray = requestBodyJsonArray;
}
protected void beforeShieldHooks() {
if (objects == null) {
return;
}
for (Object object : objects) {
RepositoryHooks.beforeShield(r, object);
}
}
public abstract void shield();
public abstract Object action();
public HttpResponse execute() {
executeShield();
Object object = action();
if (HttpResponse.class.isInstance(object)) {
return (HttpResponse) object;
}
return new JsonResponse(JsonUtils.to(object));
}
private void executeShield() {
if (!enableHooks) {
return;
}
beforeShieldHooks();
if (hasShield()) {
shield();
}
}
protected QueryBuilder> query() {
if (enableHooks) {
return r.queryWithHooks(endpointClazz);
}
return r.query(endpointClazz);
}
protected void save(Object object) {
if (enableHooks) {
r.saveWithHooks(object);
} else {
r.save(object);
}
}
protected FutureObject
© 2015 - 2025 Weber Informatics LLC | Privacy Policy