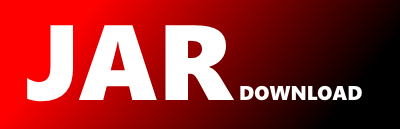
io.atomix.core.map.impl.DelegatingAsyncDistributedNavigableMap Maven / Gradle / Ivy
/*
* Copyright 2018-present Open Networking Foundation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.atomix.core.map.impl;
import com.google.common.collect.Maps;
import io.atomix.core.map.AsyncAtomicNavigableMap;
import io.atomix.core.map.AsyncDistributedNavigableMap;
import io.atomix.core.map.DistributedNavigableMap;
import io.atomix.core.map.DistributedNavigableMapType;
import io.atomix.core.set.AsyncDistributedNavigableSet;
import io.atomix.primitive.PrimitiveType;
import io.atomix.utils.time.Versioned;
import java.time.Duration;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
/**
* Delegating asynchronous distributed navigable map.
*/
public class DelegatingAsyncDistributedNavigableMap, V>
extends DelegatingAsyncDistributedSortedMap implements AsyncDistributedNavigableMap {
private final AsyncAtomicNavigableMap atomicMap;
public DelegatingAsyncDistributedNavigableMap(AsyncAtomicNavigableMap atomicMap) {
super(atomicMap);
this.atomicMap = atomicMap;
}
private Map.Entry convertEntry(Map.Entry> entry) {
return entry == null ? null : Maps.immutableEntry(entry.getKey(), Versioned.valueOrNull(entry.getValue()));
}
@Override
public PrimitiveType type() {
return DistributedNavigableMapType.instance();
}
@Override
public CompletableFuture> lowerEntry(K key) {
return atomicMap.lowerEntry(key).thenApply(this::convertEntry);
}
@Override
public CompletableFuture lowerKey(K key) {
return atomicMap.lowerKey(key);
}
@Override
public CompletableFuture> floorEntry(K key) {
return atomicMap.floorEntry(key).thenApply(this::convertEntry);
}
@Override
public CompletableFuture floorKey(K key) {
return atomicMap.floorKey(key);
}
@Override
public CompletableFuture> ceilingEntry(K key) {
return atomicMap.ceilingEntry(key).thenApply(this::convertEntry);
}
@Override
public CompletableFuture ceilingKey(K key) {
return atomicMap.ceilingKey(key);
}
@Override
public CompletableFuture> higherEntry(K key) {
return atomicMap.higherEntry(key).thenApply(this::convertEntry);
}
@Override
public CompletableFuture higherKey(K key) {
return atomicMap.higherKey(key);
}
@Override
public CompletableFuture> firstEntry() {
return atomicMap.firstEntry().thenApply(this::convertEntry);
}
@Override
public CompletableFuture> lastEntry() {
return atomicMap.lastEntry().thenApply(this::convertEntry);
}
@Override
public CompletableFuture> pollFirstEntry() {
return atomicMap.pollFirstEntry().thenApply(this::convertEntry);
}
@Override
public CompletableFuture> pollLastEntry() {
return atomicMap.pollLastEntry().thenApply(this::convertEntry);
}
@Override
public AsyncDistributedNavigableMap descendingMap() {
return new DelegatingAsyncDistributedNavigableMap<>(atomicMap.descendingMap());
}
@Override
public AsyncDistributedNavigableSet navigableKeySet() {
return atomicMap.navigableKeySet();
}
@Override
public AsyncDistributedNavigableSet descendingKeySet() {
return atomicMap.descendingKeySet();
}
@Override
public AsyncDistributedNavigableMap subMap(K fromKey, boolean fromInclusive, K toKey, boolean toInclusive) {
return new DelegatingAsyncDistributedNavigableMap<>(atomicMap.subMap(fromKey, fromInclusive, toKey, toInclusive));
}
@Override
public AsyncDistributedNavigableMap headMap(K toKey, boolean inclusive) {
return new DelegatingAsyncDistributedNavigableMap<>(atomicMap.headMap(toKey, inclusive));
}
@Override
public AsyncDistributedNavigableMap tailMap(K fromKey, boolean inclusive) {
return new DelegatingAsyncDistributedNavigableMap<>(atomicMap.tailMap(fromKey, inclusive));
}
@Override
public DistributedNavigableMap sync(Duration timeout) {
return new BlockingDistributedNavigableMap<>(this, timeout.toMillis());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy