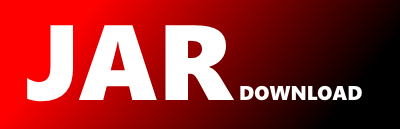
io.atomix.core.map.impl.GossipDistributedNavigableMap Maven / Gradle / Ivy
/*
* Copyright 2018-present Open Networking Foundation
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.atomix.core.map.impl;
import com.google.common.collect.Maps;
import io.atomix.core.map.MapEvent;
import io.atomix.core.map.MapEventListener;
import io.atomix.primitive.protocol.PrimitiveProtocol;
import io.atomix.primitive.protocol.map.MapDelegateEventListener;
import io.atomix.primitive.protocol.map.NavigableMapDelegate;
import java.util.Map;
import java.util.concurrent.CompletableFuture;
import java.util.concurrent.Executor;
/**
* Gossip-based distributed navigable map.
*/
public class GossipDistributedNavigableMap, V> extends AsyncDistributedNavigableJavaMap {
private final NavigableMapDelegate map;
private final Map, MapDelegateEventListener> listenerMap = Maps.newConcurrentMap();
public GossipDistributedNavigableMap(String name, PrimitiveProtocol protocol, NavigableMapDelegate map) {
super(name, protocol, map);
this.map = map;
}
@Override
public CompletableFuture addListener(MapEventListener listener, Executor executor) {
MapDelegateEventListener eventListener = event -> executor.execute(() -> {
switch (event.type()) {
case INSERT:
listener.event(new MapEvent<>(MapEvent.Type.INSERT, event.key(), event.value(), null));
break;
case UPDATE:
listener.event(new MapEvent<>(MapEvent.Type.UPDATE, event.key(), event.value(), null));
break;
case REMOVE:
listener.event(new MapEvent<>(MapEvent.Type.REMOVE, event.key(), null, event.value()));
break;
default:
break;
}
});
if (listenerMap.putIfAbsent(listener, eventListener) == null) {
return complete(() -> map.addListener(eventListener));
}
return CompletableFuture.completedFuture(null);
}
@Override
public CompletableFuture removeListener(MapEventListener listener) {
MapDelegateEventListener eventListener = listenerMap.remove(listener);
if (eventListener != null) {
return complete(() -> map.removeListener(eventListener));
}
return CompletableFuture.completedFuture(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy