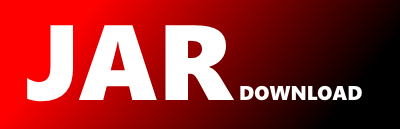
io.zeebe.clustering.gossip.GossipEventDecoder Maven / Gradle / Ivy
/* Generated SBE (Simple Binary Encoding) message codec */
package io.zeebe.clustering.gossip;
import org.agrona.MutableDirectBuffer;
import org.agrona.DirectBuffer;
import org.agrona.sbe.*;
/**
* ping probe request
*/
@SuppressWarnings("all")
public class GossipEventDecoder implements MessageDecoderFlyweight
{
public static final int BLOCK_LENGTH = 5;
public static final int TEMPLATE_ID = 0;
public static final int SCHEMA_ID = 3;
public static final int SCHEMA_VERSION = 1;
public static final java.nio.ByteOrder BYTE_ORDER = java.nio.ByteOrder.LITTLE_ENDIAN;
private final GossipEventDecoder parentMessage = this;
private DirectBuffer buffer;
protected int offset;
protected int limit;
protected int actingBlockLength;
protected int actingVersion;
public int sbeBlockLength()
{
return BLOCK_LENGTH;
}
public int sbeTemplateId()
{
return TEMPLATE_ID;
}
public int sbeSchemaId()
{
return SCHEMA_ID;
}
public int sbeSchemaVersion()
{
return SCHEMA_VERSION;
}
public String sbeSemanticType()
{
return "";
}
public DirectBuffer buffer()
{
return buffer;
}
public int offset()
{
return offset;
}
public GossipEventDecoder wrap(
final DirectBuffer buffer, final int offset, final int actingBlockLength, final int actingVersion)
{
this.buffer = buffer;
this.offset = offset;
this.actingBlockLength = actingBlockLength;
this.actingVersion = actingVersion;
limit(offset + actingBlockLength);
return this;
}
public int encodedLength()
{
return limit - offset;
}
public int limit()
{
return limit;
}
public void limit(final int limit)
{
this.limit = limit;
}
public static int eventTypeId()
{
return 0;
}
public static int eventTypeSinceVersion()
{
return 0;
}
public static int eventTypeEncodingOffset()
{
return 0;
}
public static int eventTypeEncodingLength()
{
return 1;
}
public static String eventTypeMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public GossipEventType eventType()
{
return GossipEventType.get(((short)(buffer.getByte(offset + 0) & 0xFF)));
}
public static int senderIdId()
{
return 1;
}
public static int senderIdSinceVersion()
{
return 0;
}
public static int senderIdEncodingOffset()
{
return 1;
}
public static int senderIdEncodingLength()
{
return 2;
}
public static String senderIdMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static int senderIdNullValue()
{
return 65535;
}
public static int senderIdMinValue()
{
return 0;
}
public static int senderIdMaxValue()
{
return 65534;
}
public int senderId()
{
return (buffer.getShort(offset + 1, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public static int probeMemberIdId()
{
return 2;
}
public static int probeMemberIdSinceVersion()
{
return 0;
}
public static int probeMemberIdEncodingOffset()
{
return 3;
}
public static int probeMemberIdEncodingLength()
{
return 2;
}
public static String probeMemberIdMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static int probeMemberIdNullValue()
{
return 65535;
}
public static int probeMemberIdMinValue()
{
return 0;
}
public static int probeMemberIdMaxValue()
{
return 65534;
}
public int probeMemberId()
{
return (buffer.getShort(offset + 3, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
private final MembershipEventsDecoder membershipEvents = new MembershipEventsDecoder();
public static long membershipEventsDecoderId()
{
return 3;
}
public static int membershipEventsDecoderSinceVersion()
{
return 0;
}
public MembershipEventsDecoder membershipEvents()
{
membershipEvents.wrap(parentMessage, buffer);
return membershipEvents;
}
public static class MembershipEventsDecoder
implements Iterable, java.util.Iterator
{
public static final int HEADER_SIZE = 3;
private final GroupSizeEncodingDecoder dimensions = new GroupSizeEncodingDecoder();
private GossipEventDecoder parentMessage;
private DirectBuffer buffer;
private int count;
private int index;
private int offset;
private int blockLength;
public void wrap(
final GossipEventDecoder parentMessage, final DirectBuffer buffer)
{
this.parentMessage = parentMessage;
this.buffer = buffer;
dimensions.wrap(buffer, parentMessage.limit());
blockLength = dimensions.blockLength();
count = (int)dimensions.numInGroup();
index = -1;
parentMessage.limit(parentMessage.limit() + HEADER_SIZE);
}
public static int sbeHeaderSize()
{
return HEADER_SIZE;
}
public static int sbeBlockLength()
{
return 19;
}
public int actingBlockLength()
{
return blockLength;
}
public int count()
{
return count;
}
public java.util.Iterator iterator()
{
return this;
}
public void remove()
{
throw new UnsupportedOperationException();
}
public boolean hasNext()
{
return (index + 1) < count;
}
public MembershipEventsDecoder next()
{
if (index + 1 >= count)
{
throw new java.util.NoSuchElementException();
}
offset = parentMessage.limit();
parentMessage.limit(offset + blockLength);
++index;
return this;
}
public static int eventTypeId()
{
return 4;
}
public static int eventTypeSinceVersion()
{
return 0;
}
public static int eventTypeEncodingOffset()
{
return 0;
}
public static int eventTypeEncodingLength()
{
return 1;
}
public static String eventTypeMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public MembershipEventType eventType()
{
return MembershipEventType.get(((short)(buffer.getByte(offset + 0) & 0xFF)));
}
public static int gossipEpochId()
{
return 5;
}
public static int gossipEpochSinceVersion()
{
return 0;
}
public static int gossipEpochEncodingOffset()
{
return 1;
}
public static int gossipEpochEncodingLength()
{
return 8;
}
public static String gossipEpochMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static long gossipEpochNullValue()
{
return 0xffffffffffffffffL;
}
public static long gossipEpochMinValue()
{
return 0x0L;
}
public static long gossipEpochMaxValue()
{
return 0xfffffffffffffffeL;
}
public long gossipEpoch()
{
return buffer.getLong(offset + 1, java.nio.ByteOrder.LITTLE_ENDIAN);
}
public static int gossipHeartbeatId()
{
return 6;
}
public static int gossipHeartbeatSinceVersion()
{
return 0;
}
public static int gossipHeartbeatEncodingOffset()
{
return 9;
}
public static int gossipHeartbeatEncodingLength()
{
return 8;
}
public static String gossipHeartbeatMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static long gossipHeartbeatNullValue()
{
return 0xffffffffffffffffL;
}
public static long gossipHeartbeatMinValue()
{
return 0x0L;
}
public static long gossipHeartbeatMaxValue()
{
return 0xfffffffffffffffeL;
}
public long gossipHeartbeat()
{
return buffer.getLong(offset + 9, java.nio.ByteOrder.LITTLE_ENDIAN);
}
public static int memberIdId()
{
return 7;
}
public static int memberIdSinceVersion()
{
return 0;
}
public static int memberIdEncodingOffset()
{
return 17;
}
public static int memberIdEncodingLength()
{
return 2;
}
public static String memberIdMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static int memberIdNullValue()
{
return 65535;
}
public static int memberIdMinValue()
{
return 0;
}
public static int memberIdMaxValue()
{
return 65534;
}
public int memberId()
{
return (buffer.getShort(offset + 17, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public String toString()
{
return appendTo(new StringBuilder(100)).toString();
}
public StringBuilder appendTo(final StringBuilder builder)
{
builder.append('(');
//Token{signal=BEGIN_FIELD, name='eventType', referencedName='null', description='null', id=4, version=0, deprecated=0, encodedLength=1, offset=0, componentTokenCount=9, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=BEGIN_ENUM, name='membershipEventType', referencedName='null', description='type of membership event', id=-1, version=0, deprecated=0, encodedLength=1, offset=0, componentTokenCount=7, encoding=Encoding{presence=REQUIRED, primitiveType=UINT8, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("eventType=");
builder.append(eventType());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='gossipEpoch', referencedName='null', description='null', id=5, version=0, deprecated=0, encodedLength=8, offset=1, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint64', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=8, offset=1, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT64, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("gossipEpoch=");
builder.append(gossipEpoch());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='gossipHeartbeat', referencedName='null', description='null', id=6, version=0, deprecated=0, encodedLength=8, offset=9, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint64', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=8, offset=9, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT64, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("gossipHeartbeat=");
builder.append(gossipHeartbeat());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='memberId', referencedName='null', description='null', id=7, version=0, deprecated=0, encodedLength=2, offset=17, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=17, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("memberId=");
builder.append(memberId());
builder.append(')');
return builder;
}
}
private final CustomEventsDecoder customEvents = new CustomEventsDecoder();
public static long customEventsDecoderId()
{
return 9;
}
public static int customEventsDecoderSinceVersion()
{
return 0;
}
public CustomEventsDecoder customEvents()
{
customEvents.wrap(parentMessage, buffer);
return customEvents;
}
public static class CustomEventsDecoder
implements Iterable, java.util.Iterator
{
public static final int HEADER_SIZE = 3;
private final GroupSizeEncodingDecoder dimensions = new GroupSizeEncodingDecoder();
private GossipEventDecoder parentMessage;
private DirectBuffer buffer;
private int count;
private int index;
private int offset;
private int blockLength;
public void wrap(
final GossipEventDecoder parentMessage, final DirectBuffer buffer)
{
this.parentMessage = parentMessage;
this.buffer = buffer;
dimensions.wrap(buffer, parentMessage.limit());
blockLength = dimensions.blockLength();
count = (int)dimensions.numInGroup();
index = -1;
parentMessage.limit(parentMessage.limit() + HEADER_SIZE);
}
public static int sbeHeaderSize()
{
return HEADER_SIZE;
}
public static int sbeBlockLength()
{
return 18;
}
public int actingBlockLength()
{
return blockLength;
}
public int count()
{
return count;
}
public java.util.Iterator iterator()
{
return this;
}
public void remove()
{
throw new UnsupportedOperationException();
}
public boolean hasNext()
{
return (index + 1) < count;
}
public CustomEventsDecoder next()
{
if (index + 1 >= count)
{
throw new java.util.NoSuchElementException();
}
offset = parentMessage.limit();
parentMessage.limit(offset + blockLength);
++index;
return this;
}
public static int senderGossipEpochId()
{
return 10;
}
public static int senderGossipEpochSinceVersion()
{
return 0;
}
public static int senderGossipEpochEncodingOffset()
{
return 0;
}
public static int senderGossipEpochEncodingLength()
{
return 8;
}
public static String senderGossipEpochMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static long senderGossipEpochNullValue()
{
return 0xffffffffffffffffL;
}
public static long senderGossipEpochMinValue()
{
return 0x0L;
}
public static long senderGossipEpochMaxValue()
{
return 0xfffffffffffffffeL;
}
public long senderGossipEpoch()
{
return buffer.getLong(offset + 0, java.nio.ByteOrder.LITTLE_ENDIAN);
}
public static int senderGossipHeartbeatId()
{
return 11;
}
public static int senderGossipHeartbeatSinceVersion()
{
return 0;
}
public static int senderGossipHeartbeatEncodingOffset()
{
return 8;
}
public static int senderGossipHeartbeatEncodingLength()
{
return 8;
}
public static String senderGossipHeartbeatMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static long senderGossipHeartbeatNullValue()
{
return 0xffffffffffffffffL;
}
public static long senderGossipHeartbeatMinValue()
{
return 0x0L;
}
public static long senderGossipHeartbeatMaxValue()
{
return 0xfffffffffffffffeL;
}
public long senderGossipHeartbeat()
{
return buffer.getLong(offset + 8, java.nio.ByteOrder.LITTLE_ENDIAN);
}
public static int senderIdId()
{
return 12;
}
public static int senderIdSinceVersion()
{
return 0;
}
public static int senderIdEncodingOffset()
{
return 16;
}
public static int senderIdEncodingLength()
{
return 2;
}
public static String senderIdMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "";
case TIME_UNIT: return "";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static int senderIdNullValue()
{
return 65535;
}
public static int senderIdMinValue()
{
return 0;
}
public static int senderIdMaxValue()
{
return 65534;
}
public int senderId()
{
return (buffer.getShort(offset + 16, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public static int eventTypeId()
{
return 14;
}
public static int eventTypeSinceVersion()
{
return 0;
}
public static String eventTypeCharacterEncoding()
{
return "UTF-8";
}
public static String eventTypeMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static int eventTypeHeaderLength()
{
return 2;
}
public int eventTypeLength()
{
final int limit = parentMessage.limit();
return (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public int getEventType(final MutableDirectBuffer dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public int getEventType(final byte[] dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public String eventType()
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
parentMessage.limit(limit + headerLength + dataLength);
if (0 == dataLength)
{
return "";
}
final byte[] tmp = new byte[dataLength];
buffer.getBytes(limit + headerLength, tmp, 0, dataLength);
final String value;
try
{
value = new String(tmp, "UTF-8");
}
catch (final java.io.UnsupportedEncodingException ex)
{
throw new RuntimeException(ex);
}
return value;
}
public static int payloadId()
{
return 15;
}
public static int payloadSinceVersion()
{
return 0;
}
public static String payloadCharacterEncoding()
{
return "UTF-8";
}
public static String payloadMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
case PRESENCE: return "required";
}
return "";
}
public static int payloadHeaderLength()
{
return 2;
}
public int payloadLength()
{
final int limit = parentMessage.limit();
return (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public int getPayload(final MutableDirectBuffer dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public int getPayload(final byte[] dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public String payload()
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
parentMessage.limit(limit + headerLength + dataLength);
if (0 == dataLength)
{
return "";
}
final byte[] tmp = new byte[dataLength];
buffer.getBytes(limit + headerLength, tmp, 0, dataLength);
final String value;
try
{
value = new String(tmp, "UTF-8");
}
catch (final java.io.UnsupportedEncodingException ex)
{
throw new RuntimeException(ex);
}
return value;
}
public String toString()
{
return appendTo(new StringBuilder(100)).toString();
}
public StringBuilder appendTo(final StringBuilder builder)
{
builder.append('(');
//Token{signal=BEGIN_FIELD, name='senderGossipEpoch', referencedName='null', description='null', id=10, version=0, deprecated=0, encodedLength=8, offset=0, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint64', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=8, offset=0, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT64, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("senderGossipEpoch=");
builder.append(senderGossipEpoch());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='senderGossipHeartbeat', referencedName='null', description='null', id=11, version=0, deprecated=0, encodedLength=8, offset=8, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint64', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=8, offset=8, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT64, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("senderGossipHeartbeat=");
builder.append(senderGossipHeartbeat());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='senderId', referencedName='null', description='null', id=12, version=0, deprecated=0, encodedLength=2, offset=16, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=16, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("senderId=");
builder.append(senderId());
builder.append('|');
//Token{signal=BEGIN_VAR_DATA, name='eventType', referencedName='null', description='null', id=14, version=0, deprecated=0, encodedLength=0, offset=18, componentTokenCount=6, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("eventType=");
builder.append('\'' + eventType() + '\'');
builder.append('|');
//Token{signal=BEGIN_VAR_DATA, name='payload', referencedName='null', description='null', id=15, version=0, deprecated=0, encodedLength=0, offset=-1, componentTokenCount=6, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("payload=");
builder.append('\'' + payload() + '\'');
builder.append(')');
return builder;
}
}
public String toString()
{
return appendTo(new StringBuilder(100)).toString();
}
public StringBuilder appendTo(final StringBuilder builder)
{
final int originalLimit = limit();
limit(offset + actingBlockLength);
builder.append("[GossipEvent](sbeTemplateId=");
builder.append(TEMPLATE_ID);
builder.append("|sbeSchemaId=");
builder.append(SCHEMA_ID);
builder.append("|sbeSchemaVersion=");
if (parentMessage.actingVersion != SCHEMA_VERSION)
{
builder.append(parentMessage.actingVersion);
builder.append('/');
}
builder.append(SCHEMA_VERSION);
builder.append("|sbeBlockLength=");
if (actingBlockLength != BLOCK_LENGTH)
{
builder.append(actingBlockLength);
builder.append('/');
}
builder.append(BLOCK_LENGTH);
builder.append("):");
//Token{signal=BEGIN_FIELD, name='eventType', referencedName='null', description='null', id=0, version=0, deprecated=0, encodedLength=1, offset=0, componentTokenCount=9, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=BEGIN_ENUM, name='gossipEventType', referencedName='null', description='type of the Gossip event', id=-1, version=0, deprecated=0, encodedLength=1, offset=0, componentTokenCount=7, encoding=Encoding{presence=REQUIRED, primitiveType=UINT8, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("eventType=");
builder.append(eventType());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='senderId', referencedName='null', description='null', id=1, version=0, deprecated=0, encodedLength=2, offset=1, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=1, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("senderId=");
builder.append(senderId());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='probeMemberId', referencedName='null', description='null', id=2, version=0, deprecated=0, encodedLength=2, offset=3, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', referencedName='null', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=3, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("probeMemberId=");
builder.append(probeMemberId());
builder.append('|');
//Token{signal=BEGIN_GROUP, name='membershipEvents', referencedName='null', description='null', id=3, version=0, deprecated=0, encodedLength=19, offset=5, componentTokenCount=24, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("membershipEvents=[");
MembershipEventsDecoder membershipEvents = membershipEvents();
if (membershipEvents.count() > 0)
{
while (membershipEvents.hasNext())
{
membershipEvents.next().appendTo(builder);
builder.append(',');
}
builder.setLength(builder.length() - 1);
}
builder.append(']');
builder.append('|');
//Token{signal=BEGIN_GROUP, name='customEvents', referencedName='null', description='null', id=9, version=0, deprecated=0, encodedLength=18, offset=-1, componentTokenCount=27, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='null', timeUnit=null, semanticType='null'}}
builder.append("customEvents=[");
CustomEventsDecoder customEvents = customEvents();
if (customEvents.count() > 0)
{
while (customEvents.hasNext())
{
customEvents.next().appendTo(builder);
builder.append(',');
}
builder.setLength(builder.length() - 1);
}
builder.append(']');
limit(originalLimit);
return builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy