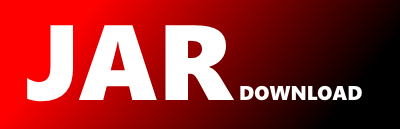
io.zeebe.servicecontainer.ServiceBuilder Maven / Gradle / Ivy
/*
* Copyright © 2017 camunda services GmbH ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package io.zeebe.servicecontainer;
import io.zeebe.servicecontainer.impl.ServiceContainerImpl;
import io.zeebe.util.sched.future.ActorFuture;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
public class ServiceBuilder {
protected final ServiceContainerImpl serviceContainer;
protected final ServiceName name;
protected final Service service;
protected ServiceName> groupName;
protected Set> dependencies = new HashSet<>();
protected Map, Collection>> injectedDependencies = new HashMap<>();
protected Map, ServiceGroupReference>> injectedReferences = new HashMap<>();
public ServiceBuilder(
ServiceName name, Service service, ServiceContainerImpl serviceContainer) {
this.name = name;
this.service = service;
this.serviceContainer = serviceContainer;
}
public ServiceBuilder group(ServiceName> groupName) {
this.groupName = groupName;
return this;
}
public ServiceBuilder dependency(ServiceName serviceName) {
dependencies.add(serviceName);
return this;
}
public ServiceBuilder dependency(
ServiceName extends T> serviceName, Injector injector) {
Collection> injectors = injectedDependencies.get(serviceName);
if (injectors == null) {
injectors = new ArrayList<>();
}
injectors.add(injector);
injectedDependencies.put(serviceName, injectors);
return dependency(serviceName);
}
public ServiceBuilder dependency(String name, Class type) {
return dependency(ServiceName.newServiceName(name, type));
}
public ServiceBuilder groupReference(
ServiceName groupName, ServiceGroupReference injector) {
injectedReferences.put(groupName, injector);
return this;
}
public ActorFuture install() {
return serviceContainer.onServiceBuilt(this);
}
public ServiceName getName() {
return name;
}
public Service getService() {
return service;
}
public Set> getDependencies() {
return dependencies;
}
public Map, Collection>> getInjectedDependencies() {
return injectedDependencies;
}
public Map, ServiceGroupReference>> getInjectedReferences() {
return injectedReferences;
}
public ServiceName> getGroupName() {
return groupName;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy