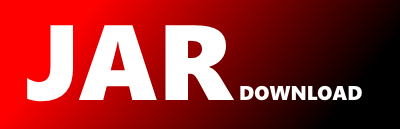
io.zeebe.clustering.management.CreatePartitionRequestDecoder Maven / Gradle / Ivy
/* Generated SBE (Simple Binary Encoding) message codec */
package io.zeebe.clustering.management;
import org.agrona.MutableDirectBuffer;
import org.agrona.DirectBuffer;
import org.agrona.sbe.*;
@javax.annotation.Generated(value = {"io.zeebe.clustering.management.CreatePartitionRequestDecoder"})
@SuppressWarnings("all")
public class CreatePartitionRequestDecoder implements MessageDecoderFlyweight
{
public static final int BLOCK_LENGTH = 2;
public static final int TEMPLATE_ID = 2;
public static final int SCHEMA_ID = 5;
public static final int SCHEMA_VERSION = 1;
private final CreatePartitionRequestDecoder parentMessage = this;
private DirectBuffer buffer;
protected int offset;
protected int limit;
protected int actingBlockLength;
protected int actingVersion;
public int sbeBlockLength()
{
return BLOCK_LENGTH;
}
public int sbeTemplateId()
{
return TEMPLATE_ID;
}
public int sbeSchemaId()
{
return SCHEMA_ID;
}
public int sbeSchemaVersion()
{
return SCHEMA_VERSION;
}
public String sbeSemanticType()
{
return "";
}
public DirectBuffer buffer()
{
return buffer;
}
public int offset()
{
return offset;
}
public CreatePartitionRequestDecoder wrap(
final DirectBuffer buffer, final int offset, final int actingBlockLength, final int actingVersion)
{
this.buffer = buffer;
this.offset = offset;
this.actingBlockLength = actingBlockLength;
this.actingVersion = actingVersion;
limit(offset + actingBlockLength);
return this;
}
public int encodedLength()
{
return limit - offset;
}
public int limit()
{
return limit;
}
public void limit(final int limit)
{
this.limit = limit;
}
public static int partitionIdId()
{
return 0;
}
public static int partitionIdSinceVersion()
{
return 0;
}
public static int partitionIdEncodingOffset()
{
return 0;
}
public static int partitionIdEncodingLength()
{
return 2;
}
public static String partitionIdMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int partitionIdNullValue()
{
return 65535;
}
public static int partitionIdMinValue()
{
return 0;
}
public static int partitionIdMaxValue()
{
return 65534;
}
public int partitionId()
{
return (buffer.getShort(offset + 0, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public static int topicNameId()
{
return 1;
}
public static int topicNameSinceVersion()
{
return 0;
}
public static String topicNameCharacterEncoding()
{
return "UTF-8";
}
public static String topicNameMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int topicNameHeaderLength()
{
return 2;
}
public int topicNameLength()
{
final int limit = parentMessage.limit();
return (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public int getTopicName(final MutableDirectBuffer dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public int getTopicName(final byte[] dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public String topicName()
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
parentMessage.limit(limit + headerLength + dataLength);
final byte[] tmp = new byte[dataLength];
buffer.getBytes(limit + headerLength, tmp, 0, dataLength);
final String value;
try
{
value = new String(tmp, "UTF-8");
}
catch (final java.io.UnsupportedEncodingException ex)
{
throw new RuntimeException(ex);
}
return value;
}
public String toString()
{
return appendTo(new StringBuilder(100)).toString();
}
public StringBuilder appendTo(final StringBuilder builder)
{
final int originalLimit = limit();
limit(offset + actingBlockLength);
builder.append("[CreatePartitionRequest](sbeTemplateId=");
builder.append(TEMPLATE_ID);
builder.append("|sbeSchemaId=");
builder.append(SCHEMA_ID);
builder.append("|sbeSchemaVersion=");
if (parentMessage.actingVersion != SCHEMA_VERSION)
{
builder.append(parentMessage.actingVersion);
builder.append('/');
}
builder.append(SCHEMA_VERSION);
builder.append("|sbeBlockLength=");
if (actingBlockLength != BLOCK_LENGTH)
{
builder.append(actingBlockLength);
builder.append('/');
}
builder.append(BLOCK_LENGTH);
builder.append("):");
//Token{signal=BEGIN_FIELD, name='partitionId', description='null', id=0, version=0, deprecated=0, encodedLength=0, offset=0, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=0, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("partitionId=");
builder.append(partitionId());
builder.append('|');
//Token{signal=BEGIN_VAR_DATA, name='topicName', description='null', id=1, version=0, deprecated=0, encodedLength=0, offset=2, componentTokenCount=6, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("topicName=");
builder.append(topicName());
limit(originalLimit);
return builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy