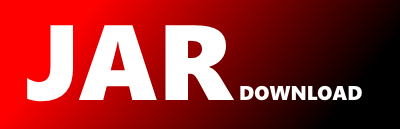
io.zeebe.clustering.raft.PollRequestDecoder Maven / Gradle / Ivy
/* Generated SBE (Simple Binary Encoding) message codec */
package io.zeebe.clustering.raft;
import org.agrona.MutableDirectBuffer;
import org.agrona.DirectBuffer;
import org.agrona.sbe.*;
@javax.annotation.Generated(value = {"io.zeebe.clustering.raft.PollRequestDecoder"})
@SuppressWarnings("all")
public class PollRequestDecoder implements MessageDecoderFlyweight
{
public static final int BLOCK_LENGTH = 18;
public static final int TEMPLATE_ID = 6;
public static final int SCHEMA_ID = 4;
public static final int SCHEMA_VERSION = 1;
private final PollRequestDecoder parentMessage = this;
private DirectBuffer buffer;
protected int offset;
protected int limit;
protected int actingBlockLength;
protected int actingVersion;
public int sbeBlockLength()
{
return BLOCK_LENGTH;
}
public int sbeTemplateId()
{
return TEMPLATE_ID;
}
public int sbeSchemaId()
{
return SCHEMA_ID;
}
public int sbeSchemaVersion()
{
return SCHEMA_VERSION;
}
public String sbeSemanticType()
{
return "";
}
public DirectBuffer buffer()
{
return buffer;
}
public int offset()
{
return offset;
}
public PollRequestDecoder wrap(
final DirectBuffer buffer, final int offset, final int actingBlockLength, final int actingVersion)
{
this.buffer = buffer;
this.offset = offset;
this.actingBlockLength = actingBlockLength;
this.actingVersion = actingVersion;
limit(offset + actingBlockLength);
return this;
}
public int encodedLength()
{
return limit - offset;
}
public int limit()
{
return limit;
}
public void limit(final int limit)
{
this.limit = limit;
}
public static int partitionIdId()
{
return 0;
}
public static int partitionIdSinceVersion()
{
return 0;
}
public static int partitionIdEncodingOffset()
{
return 0;
}
public static int partitionIdEncodingLength()
{
return 2;
}
public static String partitionIdMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int partitionIdNullValue()
{
return 65535;
}
public static int partitionIdMinValue()
{
return 0;
}
public static int partitionIdMaxValue()
{
return 65534;
}
public int partitionId()
{
return (buffer.getShort(offset + 0, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public static int termId()
{
return 1;
}
public static int termSinceVersion()
{
return 0;
}
public static int termEncodingOffset()
{
return 2;
}
public static int termEncodingLength()
{
return 2;
}
public static String termMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int termNullValue()
{
return 65535;
}
public static int termMinValue()
{
return 0;
}
public static int termMaxValue()
{
return 65534;
}
public int term()
{
return (buffer.getShort(offset + 2, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public static int lastEntryPositionId()
{
return 2;
}
public static int lastEntryPositionSinceVersion()
{
return 0;
}
public static int lastEntryPositionEncodingOffset()
{
return 4;
}
public static int lastEntryPositionEncodingLength()
{
return 8;
}
public static String lastEntryPositionMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static long lastEntryPositionNullValue()
{
return 0xffffffffffffffffL;
}
public static long lastEntryPositionMinValue()
{
return 0x0L;
}
public static long lastEntryPositionMaxValue()
{
return 0xfffffffffffffffeL;
}
public long lastEntryPosition()
{
return buffer.getLong(offset + 4, java.nio.ByteOrder.LITTLE_ENDIAN);
}
public static int lastEntryTermId()
{
return 3;
}
public static int lastEntryTermSinceVersion()
{
return 0;
}
public static int lastEntryTermEncodingOffset()
{
return 12;
}
public static int lastEntryTermEncodingLength()
{
return 4;
}
public static String lastEntryTermMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int lastEntryTermNullValue()
{
return -2147483648;
}
public static int lastEntryTermMinValue()
{
return -2147483647;
}
public static int lastEntryTermMaxValue()
{
return 2147483647;
}
public int lastEntryTerm()
{
return buffer.getInt(offset + 12, java.nio.ByteOrder.LITTLE_ENDIAN);
}
public static int portId()
{
return 4;
}
public static int portSinceVersion()
{
return 0;
}
public static int portEncodingOffset()
{
return 16;
}
public static int portEncodingLength()
{
return 2;
}
public static String portMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int portNullValue()
{
return 65535;
}
public static int portMinValue()
{
return 0;
}
public static int portMaxValue()
{
return 65534;
}
public int port()
{
return (buffer.getShort(offset + 16, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public static int topicNameId()
{
return 5;
}
public static int topicNameSinceVersion()
{
return 0;
}
public static String topicNameCharacterEncoding()
{
return "UTF-8";
}
public static String topicNameMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int topicNameHeaderLength()
{
return 2;
}
public int topicNameLength()
{
final int limit = parentMessage.limit();
return (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public int getTopicName(final MutableDirectBuffer dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public int getTopicName(final byte[] dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public String topicName()
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
parentMessage.limit(limit + headerLength + dataLength);
final byte[] tmp = new byte[dataLength];
buffer.getBytes(limit + headerLength, tmp, 0, dataLength);
final String value;
try
{
value = new String(tmp, "UTF-8");
}
catch (final java.io.UnsupportedEncodingException ex)
{
throw new RuntimeException(ex);
}
return value;
}
public static int hostId()
{
return 6;
}
public static int hostSinceVersion()
{
return 0;
}
public static String hostCharacterEncoding()
{
return "UTF-8";
}
public static String hostMetaAttribute(final MetaAttribute metaAttribute)
{
switch (metaAttribute)
{
case EPOCH: return "unix";
case TIME_UNIT: return "nanosecond";
case SEMANTIC_TYPE: return "";
}
return "";
}
public static int hostHeaderLength()
{
return 2;
}
public int hostLength()
{
final int limit = parentMessage.limit();
return (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
}
public int getHost(final MutableDirectBuffer dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public int getHost(final byte[] dst, final int dstOffset, final int length)
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
final int bytesCopied = Math.min(length, dataLength);
parentMessage.limit(limit + headerLength + dataLength);
buffer.getBytes(limit + headerLength, dst, dstOffset, bytesCopied);
return bytesCopied;
}
public String host()
{
final int headerLength = 2;
final int limit = parentMessage.limit();
final int dataLength = (int)(buffer.getShort(limit, java.nio.ByteOrder.LITTLE_ENDIAN) & 0xFFFF);
parentMessage.limit(limit + headerLength + dataLength);
final byte[] tmp = new byte[dataLength];
buffer.getBytes(limit + headerLength, tmp, 0, dataLength);
final String value;
try
{
value = new String(tmp, "UTF-8");
}
catch (final java.io.UnsupportedEncodingException ex)
{
throw new RuntimeException(ex);
}
return value;
}
public String toString()
{
return appendTo(new StringBuilder(100)).toString();
}
public StringBuilder appendTo(final StringBuilder builder)
{
final int originalLimit = limit();
limit(offset + actingBlockLength);
builder.append("[PollRequest](sbeTemplateId=");
builder.append(TEMPLATE_ID);
builder.append("|sbeSchemaId=");
builder.append(SCHEMA_ID);
builder.append("|sbeSchemaVersion=");
if (parentMessage.actingVersion != SCHEMA_VERSION)
{
builder.append(parentMessage.actingVersion);
builder.append('/');
}
builder.append(SCHEMA_VERSION);
builder.append("|sbeBlockLength=");
if (actingBlockLength != BLOCK_LENGTH)
{
builder.append(actingBlockLength);
builder.append('/');
}
builder.append(BLOCK_LENGTH);
builder.append("):");
//Token{signal=BEGIN_FIELD, name='partitionId', description='null', id=0, version=0, deprecated=0, encodedLength=0, offset=0, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=0, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("partitionId=");
builder.append(partitionId());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='term', description='null', id=1, version=0, deprecated=0, encodedLength=0, offset=2, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=2, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("term=");
builder.append(term());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='lastEntryPosition', description='null', id=2, version=0, deprecated=0, encodedLength=0, offset=4, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
//Token{signal=ENCODING, name='uint64', description='null', id=-1, version=0, deprecated=0, encodedLength=8, offset=4, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT64, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("lastEntryPosition=");
builder.append(lastEntryPosition());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='lastEntryTerm', description='null', id=3, version=0, deprecated=0, encodedLength=0, offset=12, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
//Token{signal=ENCODING, name='int32', description='null', id=-1, version=0, deprecated=0, encodedLength=4, offset=12, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=INT32, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("lastEntryTerm=");
builder.append(lastEntryTerm());
builder.append('|');
//Token{signal=BEGIN_FIELD, name='port', description='null', id=4, version=0, deprecated=0, encodedLength=0, offset=16, componentTokenCount=3, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
//Token{signal=ENCODING, name='uint16', description='null', id=-1, version=0, deprecated=0, encodedLength=2, offset=16, componentTokenCount=1, encoding=Encoding{presence=REQUIRED, primitiveType=UINT16, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("port=");
builder.append(port());
builder.append('|');
//Token{signal=BEGIN_VAR_DATA, name='topicName', description='null', id=5, version=0, deprecated=0, encodedLength=0, offset=18, componentTokenCount=6, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("topicName=");
builder.append(topicName());
builder.append('|');
//Token{signal=BEGIN_VAR_DATA, name='host', description='null', id=6, version=0, deprecated=0, encodedLength=0, offset=-1, componentTokenCount=6, encoding=Encoding{presence=REQUIRED, primitiveType=null, byteOrder=LITTLE_ENDIAN, minValue=null, maxValue=null, nullValue=null, constValue=null, characterEncoding='null', epoch='unix', timeUnit=nanosecond, semanticType='null'}}
builder.append("host=");
builder.append(host());
limit(originalLimit);
return builder;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy