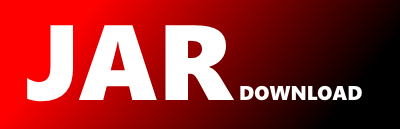
io.zeebe.protocol.record.AbstractRecordAssert Maven / Gradle / Ivy
package io.zeebe.protocol.record;
import org.assertj.core.api.AbstractObjectAssert;
import org.assertj.core.util.Objects;
/**
* Abstract base class for {@link Record} specific assertions - Generated by CustomAssertionGenerator.
*/
@javax.annotation.Generated(value="assertj-assertions-generator")
public abstract class AbstractRecordAssert, A extends Record> extends AbstractObjectAssert {
/**
* Creates a new {@link AbstractRecordAssert}
to make assertions on actual Record.
* @param actual the Record we want to make assertions on.
*/
protected AbstractRecordAssert(A actual, Class selfType) {
super(actual, selfType);
}
/**
* Verifies that the actual Record's brokerVersion is equal to the given one.
* @param brokerVersion the given brokerVersion to compare the actual Record's brokerVersion to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's brokerVersion is not equal to the given one.
*/
public S hasBrokerVersion(String brokerVersion) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting brokerVersion of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualBrokerVersion = actual.getBrokerVersion();
if (!Objects.areEqual(actualBrokerVersion, brokerVersion)) {
failWithMessage(assertjErrorMessage, actual, brokerVersion, actualBrokerVersion);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's intent is equal to the given one.
* @param intent the given intent to compare the actual Record's intent to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's intent is not equal to the given one.
*/
public S hasIntent(io.zeebe.protocol.record.intent.Intent intent) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting intent of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
io.zeebe.protocol.record.intent.Intent actualIntent = actual.getIntent();
if (!Objects.areEqual(actualIntent, intent)) {
failWithMessage(assertjErrorMessage, actual, intent, actualIntent);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's key is equal to the given one.
* @param key the given key to compare the actual Record's key to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's key is not equal to the given one.
*/
public S hasKey(long key) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting key of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check
long actualKey = actual.getKey();
if (actualKey != key) {
failWithMessage(assertjErrorMessage, actual, key, actualKey);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's partitionId is equal to the given one.
* @param partitionId the given partitionId to compare the actual Record's partitionId to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's partitionId is not equal to the given one.
*/
public S hasPartitionId(int partitionId) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting partitionId of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check
int actualPartitionId = actual.getPartitionId();
if (actualPartitionId != partitionId) {
failWithMessage(assertjErrorMessage, actual, partitionId, actualPartitionId);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's position is equal to the given one.
* @param position the given position to compare the actual Record's position to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's position is not equal to the given one.
*/
public S hasPosition(long position) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting position of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check
long actualPosition = actual.getPosition();
if (actualPosition != position) {
failWithMessage(assertjErrorMessage, actual, position, actualPosition);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's recordType is equal to the given one.
* @param recordType the given recordType to compare the actual Record's recordType to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's recordType is not equal to the given one.
*/
public S hasRecordType(RecordType recordType) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting recordType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
RecordType actualRecordType = actual.getRecordType();
if (!Objects.areEqual(actualRecordType, recordType)) {
failWithMessage(assertjErrorMessage, actual, recordType, actualRecordType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's rejectionReason is equal to the given one.
* @param rejectionReason the given rejectionReason to compare the actual Record's rejectionReason to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's rejectionReason is not equal to the given one.
*/
public S hasRejectionReason(String rejectionReason) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting rejectionReason of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
String actualRejectionReason = actual.getRejectionReason();
if (!Objects.areEqual(actualRejectionReason, rejectionReason)) {
failWithMessage(assertjErrorMessage, actual, rejectionReason, actualRejectionReason);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's rejectionType is equal to the given one.
* @param rejectionType the given rejectionType to compare the actual Record's rejectionType to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's rejectionType is not equal to the given one.
*/
public S hasRejectionType(RejectionType rejectionType) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting rejectionType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
RejectionType actualRejectionType = actual.getRejectionType();
if (!Objects.areEqual(actualRejectionType, rejectionType)) {
failWithMessage(assertjErrorMessage, actual, rejectionType, actualRejectionType);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's sourceRecordPosition is equal to the given one.
* @param sourceRecordPosition the given sourceRecordPosition to compare the actual Record's sourceRecordPosition to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's sourceRecordPosition is not equal to the given one.
*/
public S hasSourceRecordPosition(long sourceRecordPosition) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting sourceRecordPosition of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check
long actualSourceRecordPosition = actual.getSourceRecordPosition();
if (actualSourceRecordPosition != sourceRecordPosition) {
failWithMessage(assertjErrorMessage, actual, sourceRecordPosition, actualSourceRecordPosition);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's timestamp is equal to the given one.
* @param timestamp the given timestamp to compare the actual Record's timestamp to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's timestamp is not equal to the given one.
*/
public S hasTimestamp(long timestamp) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting timestamp of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// check
long actualTimestamp = actual.getTimestamp();
if (actualTimestamp != timestamp) {
failWithMessage(assertjErrorMessage, actual, timestamp, actualTimestamp);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's value is equal to the given one.
* @param value the given value to compare the actual Record's value to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's value is not equal to the given one.
*/
public S hasValue(RecordValue value) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting value of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
RecordValue actualValue = actual.getValue();
if (!Objects.areEqual(actualValue, value)) {
failWithMessage(assertjErrorMessage, actual, value, actualValue);
}
// return the current assertion for method chaining
return myself;
}
/**
* Verifies that the actual Record's valueType is equal to the given one.
* @param valueType the given valueType to compare the actual Record's valueType to.
* @return this assertion object.
* @throws AssertionError - if the actual Record's valueType is not equal to the given one.
*/
public S hasValueType(ValueType valueType) {
// check that actual Record we want to make assertions on is not null.
isNotNull();
// overrides the default error message with a more explicit one
String assertjErrorMessage = "\nExpecting valueType of:\n <%s>\nto be:\n <%s>\nbut was:\n <%s>";
// null safe check
ValueType actualValueType = actual.getValueType();
if (!Objects.areEqual(actualValueType, valueType)) {
failWithMessage(assertjErrorMessage, actual, valueType, actualValueType);
}
// return the current assertion for method chaining
return myself;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy